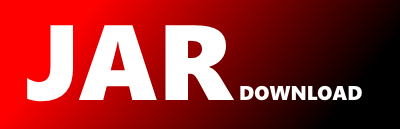
com.azure.maps.weather.models.StormForecast Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-maps-weather Show documentation
Show all versions of azure-maps-weather Show documentation
This package contains the Microsoft Azure SDK for Weather SDK. For documentation on how to use this package, please see https://docs.microsoft.com/en-us/rest/api/maps/weather. Package tag 1.0-preview.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.maps.weather.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.maps.weather.implementation.models.LatLongPair;
import java.io.IOException;
import java.util.List;
import com.azure.core.models.GeoPosition;
/**
* Government-issued storm forecast.
*/
@Fluent
public final class StormForecast implements JsonSerializable {
/*
* Datetime the forecast is valid, displayed in ISO8601 format.
*/
private String timestamp;
/*
* Datetime the forecast was created, displayed in ISO8601 format.
*/
private String initializedTimestamp;
/*
* Coordinates of the storm
*/
private LatLongPair coordinates;
/*
* Maximum wind gust speed associated with the storm. May be NULL.
*/
private WeatherUnitDetails maxWindGust;
/*
* Maximum sustained wind speed associated with the storm. May be NULL.
*/
private WeatherUnitDetails sustainedWind;
/*
* Possible status values include:- Cyclonic storm
- Deep depression
- Depression
- Extremely severe cyclonic storm
- Hurricane category (1-5)
- Intense tropical cyclone
- Moderate tropical storm
- Post-tropical cyclone
- Potential tropical cyclone
- Severe cyclonic storm
- Severe tropical storm
- Subtropical
- Super cyclonic storm
- Tropical cyclone
- Tropical cyclone category (1-5)
- Tropical depression
- Tropical disturbance
- Tropical storm
- Typhoon
- Very intense tropical cyclone
- Very severe cyclonic storm
- Very strong typhoon
- Violent typhoon
*/
private String status;
/*
* Contains information about the forecast window for the storm during the specified time period (not the entire cone). If windowGeometry=true in the request, this object will include geoJSON details for window geometry.
*/
private WeatherWindow weatherWindow;
/*
* Displayed when details=true or radiiGeometry=true in the request.
*/
private List windRadiiSummary;
/**
* Set default StormForecast constructor to private
*/
private StormForecast() {
}
/**
* Get the timestamp property: Datetime the forecast is valid, displayed in ISO8601 format.
*
* @return the timestamp value.
*/
public String getTimestamp() {
return this.timestamp;
}
/**
* Get the initializedTimestamp property: Datetime the forecast was created, displayed in ISO8601 format.
*
* @return the initializedTimestamp value.
*/
public String getInitializedTimestamp() {
return this.initializedTimestamp;
}
/**
* Get the coordinates property: Coordinates of the storm.
*
* @return the coordinates value.
*/
public GeoPosition getCoordinates() {
return new GeoPosition(this.coordinates.getLongitude(), this.coordinates.getLatitude());
}
/**
* Get the maxWindGust property: Maximum wind gust speed associated with the storm. May be NULL.
*
* @return the maxWindGust value.
*/
public WeatherUnitDetails getMaxWindGust() {
return this.maxWindGust;
}
/**
* Get the sustainedWind property: Maximum sustained wind speed associated with the storm. May be NULL.
*
* @return the sustainedWind value.
*/
public WeatherUnitDetails getSustainedWind() {
return this.sustainedWind;
}
/**
* Get the status property: Possible status values include:<ul><li>Cyclonic
* storm</li><li>Deep depression</li><li>Depression</li><li>Extremely severe
* cyclonic storm</li><li>Hurricane category (1-5)</li><li>Intense tropical
* cyclone</li><li>Moderate tropical storm</li><li>Post-tropical
* cyclone</li><li>Potential tropical cyclone</li><li>Severe cyclonic
* storm</li><li>Severe tropical storm</li><li>Subtropical</li><li>Super
* cyclonic storm</li><li>Tropical cyclone</li><li>Tropical cyclone category
* (1-5)</li><li>Tropical depression</li><li>Tropical
* disturbance</li><li>Tropical storm</li><li>Typhoon</li><li>Very intense
* tropical cyclone</li><li>Very severe cyclonic storm</li><li>Very strong
* typhoon</li><li>Violent typhoon</li></ul>.
*
* @return the status value.
*/
public String getStatus() {
return this.status;
}
/**
* Get the weatherWindow property: Contains information about the forecast window for the storm during the specified
* time period (not the entire cone). If windowGeometry=true in the request, this object will include geoJSON
* details for window geometry.
*
* @return the weatherWindow value.
*/
public WeatherWindow getWeatherWindow() {
return this.weatherWindow;
}
/**
* Get the windRadiiSummary property: Displayed when details=true or radiiGeometry=true in the request.
*
* @return the windRadiiSummary value.
*/
public List getWindRadiiSummary() {
return this.windRadiiSummary;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("dateTime", this.timestamp);
jsonWriter.writeStringField("initializedDateTime", this.initializedTimestamp);
jsonWriter.writeJsonField("location", this.coordinates);
jsonWriter.writeJsonField("maxWindGust", this.maxWindGust);
jsonWriter.writeJsonField("sustainedWind", this.sustainedWind);
jsonWriter.writeStringField("status", this.status);
jsonWriter.writeJsonField("window", this.weatherWindow);
jsonWriter.writeArrayField("windRadiiSummary", this.windRadiiSummary,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StormForecast from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StormForecast if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the StormForecast.
*/
public static StormForecast fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StormForecast deserializedStormForecast = new StormForecast();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("dateTime".equals(fieldName)) {
deserializedStormForecast.timestamp = reader.getString();
} else if ("initializedDateTime".equals(fieldName)) {
deserializedStormForecast.initializedTimestamp = reader.getString();
} else if ("location".equals(fieldName)) {
deserializedStormForecast.coordinates = LatLongPair.fromJson(reader);
} else if ("maxWindGust".equals(fieldName)) {
deserializedStormForecast.maxWindGust = WeatherUnitDetails.fromJson(reader);
} else if ("sustainedWind".equals(fieldName)) {
deserializedStormForecast.sustainedWind = WeatherUnitDetails.fromJson(reader);
} else if ("status".equals(fieldName)) {
deserializedStormForecast.status = reader.getString();
} else if ("window".equals(fieldName)) {
deserializedStormForecast.weatherWindow = WeatherWindow.fromJson(reader);
} else if ("windRadiiSummary".equals(fieldName)) {
List windRadiiSummary
= reader.readArray(reader1 -> StormWindRadiiSummary.fromJson(reader1));
deserializedStormForecast.windRadiiSummary = windRadiiSummary;
} else {
reader.skipChildren();
}
}
return deserializedStormForecast;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy