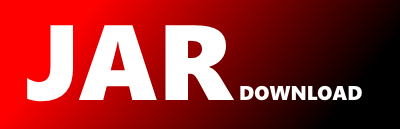
com.azure.messaging.eventgrid.namespaces.EventGridSenderAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
This package contains the Microsoft Azure EventGrid Namespaces client library.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.messaging.eventgrid.namespaces;
import com.azure.core.annotation.Generated;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.exception.ClientAuthenticationException;
import com.azure.core.exception.HttpResponseException;
import com.azure.core.exception.ResourceModifiedException;
import com.azure.core.exception.ResourceNotFoundException;
import com.azure.core.http.rest.RequestOptions;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.models.CloudEvent;
import com.azure.core.util.BinaryData;
import com.azure.messaging.eventgrid.namespaces.implementation.EventGridSenderClientImpl;
import java.util.List;
import reactor.core.publisher.Mono;
/**
* Initializes a new instance of the asynchronous EventGridSenderClient type.
*/
@ServiceClient(builder = EventGridSenderClientBuilder.class, isAsync = true)
public final class EventGridSenderAsyncClient {
@Generated
private final EventGridSenderClientImpl serviceClient;
private final String topicName;
/**
* Initializes an instance of EventGridSenderAsyncClient class.
*
* @param serviceClient the service client implementation.
* @param topicName the topicName for this client.
*/
EventGridSenderAsyncClient(EventGridSenderClientImpl serviceClient, String topicName) {
this.serviceClient = serviceClient;
this.topicName = topicName;
}
/**
* Publish a single Cloud Event to a namespace topic.
* Request Body Schema
*
*
* {@code
* {
* id: String (Required)
* source: String (Required)
* data: BinaryData (Optional)
* data_base64: byte[] (Optional)
* type: String (Required)
* time: OffsetDateTime (Optional)
* specversion: String (Required)
* dataschema: String (Optional)
* datacontenttype: String (Optional)
* subject: String (Optional)
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* }
* }
*
*
* @param topicName Topic Name.
* @param event Single Cloud Event being published.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Publish operation along with {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> sendWithResponse(String topicName, BinaryData event, RequestOptions requestOptions) {
return this.serviceClient.sendWithResponseAsync(topicName, event, requestOptions);
}
/**
* Publish a batch of Cloud Events to a namespace topic.
* Request Body Schema
*
*
* {@code
* [
* (Required){
* id: String (Required)
* source: String (Required)
* data: BinaryData (Optional)
* data_base64: byte[] (Optional)
* type: String (Required)
* time: OffsetDateTime (Optional)
* specversion: String (Required)
* dataschema: String (Optional)
* datacontenttype: String (Optional)
* subject: String (Optional)
* }
* ]
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* }
* }
*
*
* @param topicName Topic Name.
* @param events Array of Cloud Events being published.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Publish operation along with {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> sendEventsWithResponse(String topicName, BinaryData events,
RequestOptions requestOptions) {
return this.serviceClient.sendEventsWithResponseAsync(topicName, events, requestOptions);
}
/**
* Publish a single Cloud Event to a namespace topic.
*
* @param event Single Cloud Event being published.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of the Publish operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono send(CloudEvent event) {
RequestOptions requestOptions = new RequestOptions();
return sendWithResponse(topicName, BinaryData.fromObject(event), requestOptions).then();
}
/**
* Publish a single Cloud Event to a namespace topic.
*
* @param event Single Cloud Event being published.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of the Publish operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> sendWithResponse(CloudEvent event, RequestOptions requestOptions) {
return sendWithResponse(topicName, BinaryData.fromObject(event), requestOptions).map(response -> {
return new SimpleResponse<>(response, null);
});
}
/**
* Publish a batch of Cloud Events to a namespace topic.
*
* @param events Array of Cloud Events being published.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of the Publish operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono send(List events) {
RequestOptions requestOptions = new RequestOptions();
return sendEventsWithResponse(topicName, BinaryData.fromObject(events), requestOptions).then();
}
/**
* Publish a batch of Cloud Events to a namespace topic.
*
* @param events Array of Cloud Events being published.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of the Publish operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> sendWithResponse(List events, RequestOptions requestOptions) {
return sendEventsWithResponse(topicName, BinaryData.fromObject(events), requestOptions).map(response -> {
return new SimpleResponse<>(response, null);
});
}
/**
* Gets the topicName for this client.
*
* @return the topic name.
*/
public String getTopicName() {
return topicName;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy