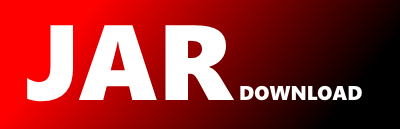
com.azure.messaging.eventgrid.namespaces.implementation.EventGridReceiverClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
This package contains the Microsoft Azure EventGrid Namespaces client library.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.messaging.eventgrid.namespaces.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.exception.ClientAuthenticationException;
import com.azure.core.exception.HttpResponseException;
import com.azure.core.exception.ResourceModifiedException;
import com.azure.core.exception.ResourceNotFoundException;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.HttpPipelineBuilder;
import com.azure.core.http.policy.RetryPolicy;
import com.azure.core.http.policy.UserAgentPolicy;
import com.azure.core.http.rest.RequestOptions;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.util.BinaryData;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.serializer.JacksonAdapter;
import com.azure.core.util.serializer.SerializerAdapter;
import com.azure.messaging.eventgrid.namespaces.EventGridServiceVersion;
import reactor.core.publisher.Mono;
/**
* Initializes a new instance of the EventGridReceiverClient type.
*/
public final class EventGridReceiverClientImpl {
/**
* The proxy service used to perform REST calls.
*/
private final EventGridReceiverClientService service;
/**
* The host name of the namespace, e.g. namespaceName1.westus-1.eventgrid.azure.net.
*/
private final String endpoint;
/**
* Gets The host name of the namespace, e.g. namespaceName1.westus-1.eventgrid.azure.net.
*
* @return the endpoint value.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
* Service version.
*/
private final EventGridServiceVersion serviceVersion;
/**
* Gets Service version.
*
* @return the serviceVersion value.
*/
public EventGridServiceVersion getServiceVersion() {
return this.serviceVersion;
}
/**
* The HTTP pipeline to send requests through.
*/
private final HttpPipeline httpPipeline;
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
public HttpPipeline getHttpPipeline() {
return this.httpPipeline;
}
/**
* The serializer to serialize an object into a string.
*/
private final SerializerAdapter serializerAdapter;
/**
* Gets The serializer to serialize an object into a string.
*
* @return the serializerAdapter value.
*/
public SerializerAdapter getSerializerAdapter() {
return this.serializerAdapter;
}
/**
* Initializes an instance of EventGridReceiverClient client.
*
* @param endpoint The host name of the namespace, e.g. namespaceName1.westus-1.eventgrid.azure.net.
* @param serviceVersion Service version.
*/
public EventGridReceiverClientImpl(String endpoint, EventGridServiceVersion serviceVersion) {
this(new HttpPipelineBuilder().policies(new UserAgentPolicy(), new RetryPolicy()).build(),
JacksonAdapter.createDefaultSerializerAdapter(), endpoint, serviceVersion);
}
/**
* Initializes an instance of EventGridReceiverClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param endpoint The host name of the namespace, e.g. namespaceName1.westus-1.eventgrid.azure.net.
* @param serviceVersion Service version.
*/
public EventGridReceiverClientImpl(HttpPipeline httpPipeline, String endpoint,
EventGridServiceVersion serviceVersion) {
this(httpPipeline, JacksonAdapter.createDefaultSerializerAdapter(), endpoint, serviceVersion);
}
/**
* Initializes an instance of EventGridReceiverClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param serializerAdapter The serializer to serialize an object into a string.
* @param endpoint The host name of the namespace, e.g. namespaceName1.westus-1.eventgrid.azure.net.
* @param serviceVersion Service version.
*/
public EventGridReceiverClientImpl(HttpPipeline httpPipeline, SerializerAdapter serializerAdapter, String endpoint,
EventGridServiceVersion serviceVersion) {
this.httpPipeline = httpPipeline;
this.serializerAdapter = serializerAdapter;
this.endpoint = endpoint;
this.serviceVersion = serviceVersion;
this.service
= RestProxy.create(EventGridReceiverClientService.class, this.httpPipeline, this.getSerializerAdapter());
}
/**
* The interface defining all the services for EventGridReceiverClient to be used by the proxy service to perform
* REST calls.
*/
@Host("{endpoint}")
@ServiceInterface(name = "EventGridReceiverCli")
public interface EventGridReceiverClientService {
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:receive")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> receive(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName, @HeaderParam("Accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:receive")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response receiveSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName, @HeaderParam("Accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:acknowledge")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> acknowledge(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData acknowledgeRequest, RequestOptions requestOptions,
Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:acknowledge")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response acknowledgeSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData acknowledgeRequest, RequestOptions requestOptions,
Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:release")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> release(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData releaseRequest, RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:release")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response releaseSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData releaseRequest, RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:reject")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> reject(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData rejectRequest, RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:reject")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response rejectSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData rejectRequest, RequestOptions requestOptions, Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:renewLock")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> renewLocks(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData renewLocksRequest, RequestOptions requestOptions,
Context context);
@Post("/topics/{topicName}/eventsubscriptions/{eventSubscriptionName}:renewLock")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response renewLocksSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("topicName") String topicName,
@PathParam("eventSubscriptionName") String eventSubscriptionName,
@HeaderParam("Content-Type") String contentType, @HeaderParam("Accept") String accept,
@BodyParam("application/json") BinaryData renewLocksRequest, RequestOptions requestOptions,
Context context);
}
/**
* Receive a batch of Cloud Events from a subscription.
* Query Parameters
*
* Query Parameters
* Name Type Required Description
* maxEvents Integer No Max Events count to be received. Minimum value is 1, while
* maximum value is 100 events. If not specified, the default value is 1.
* maxWaitTime Duration No Max wait time value for receive operation in Seconds. It
* is the time in seconds that the server approximately waits for the availability of an event and responds to the
* request. If an event is available, the broker responds immediately to the client. Minimum value is 10 seconds,
* while maximum value is 120 seconds. If not specified, the default value is 60 seconds.
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
* Response Body Schema
*
*
* {@code
* {
* value (Required): [
* (Required){
* brokerProperties (Required): {
* lockToken: String (Required)
* deliveryCount: int (Required)
* }
* event (Required): {
* id: String (Required)
* source: String (Required)
* data: BinaryData (Optional)
* data_base64: byte[] (Optional)
* type: String (Required)
* time: OffsetDateTime (Optional)
* specversion: String (Required)
* dataschema: String (Optional)
* datacontenttype: String (Optional)
* subject: String (Optional)
* }
* }
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return details of the Receive operation response along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> receiveWithResponseAsync(String topicName, String eventSubscriptionName,
RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.receive(this.getEndpoint(),
this.getServiceVersion().getVersion(), topicName, eventSubscriptionName, accept, requestOptions, context));
}
/**
* Receive a batch of Cloud Events from a subscription.
* Query Parameters
*
* Query Parameters
* Name Type Required Description
* maxEvents Integer No Max Events count to be received. Minimum value is 1, while
* maximum value is 100 events. If not specified, the default value is 1.
* maxWaitTime Duration No Max wait time value for receive operation in Seconds. It
* is the time in seconds that the server approximately waits for the availability of an event and responds to the
* request. If an event is available, the broker responds immediately to the client. Minimum value is 10 seconds,
* while maximum value is 120 seconds. If not specified, the default value is 60 seconds.
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
* Response Body Schema
*
*
* {@code
* {
* value (Required): [
* (Required){
* brokerProperties (Required): {
* lockToken: String (Required)
* deliveryCount: int (Required)
* }
* event (Required): {
* id: String (Required)
* source: String (Required)
* data: BinaryData (Optional)
* data_base64: byte[] (Optional)
* type: String (Required)
* time: OffsetDateTime (Optional)
* specversion: String (Required)
* dataschema: String (Optional)
* datacontenttype: String (Optional)
* subject: String (Optional)
* }
* }
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return details of the Receive operation response along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response receiveWithResponse(String topicName, String eventSubscriptionName,
RequestOptions requestOptions) {
final String accept = "application/json";
return service.receiveSync(this.getEndpoint(), this.getServiceVersion().getVersion(), topicName,
eventSubscriptionName, accept, requestOptions, Context.NONE);
}
/**
* Acknowledge a batch of Cloud Events. The response will include the set of successfully acknowledged lock tokens,
* along with other failed lock tokens with their corresponding error information. Successfully acknowledged events
* will no longer be available to be received by any consumer.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param acknowledgeRequest The acknowledgeRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Acknowledge operation along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> acknowledgeWithResponseAsync(String topicName, String eventSubscriptionName,
BinaryData acknowledgeRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.acknowledge(this.getEndpoint(), this.getServiceVersion().getVersion(),
topicName, eventSubscriptionName, contentType, accept, acknowledgeRequest, requestOptions, context));
}
/**
* Acknowledge a batch of Cloud Events. The response will include the set of successfully acknowledged lock tokens,
* along with other failed lock tokens with their corresponding error information. Successfully acknowledged events
* will no longer be available to be received by any consumer.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param acknowledgeRequest The acknowledgeRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Acknowledge operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response acknowledgeWithResponse(String topicName, String eventSubscriptionName,
BinaryData acknowledgeRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return service.acknowledgeSync(this.getEndpoint(), this.getServiceVersion().getVersion(), topicName,
eventSubscriptionName, contentType, accept, acknowledgeRequest, requestOptions, Context.NONE);
}
/**
* Release a batch of Cloud Events. The response will include the set of successfully released lock tokens, along
* with other failed lock tokens with their corresponding error information. Successfully released events can be
* received by consumers.
* Query Parameters
*
* Query Parameters
* Name Type Required Description
* releaseDelayInSeconds String No Release cloud events with the specified delay in
* seconds. Allowed values: "0", "10", "60", "600", "3600".
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param releaseRequest The releaseRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Release operation along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> releaseWithResponseAsync(String topicName, String eventSubscriptionName,
BinaryData releaseRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.release(this.getEndpoint(), this.getServiceVersion().getVersion(),
topicName, eventSubscriptionName, contentType, accept, releaseRequest, requestOptions, context));
}
/**
* Release a batch of Cloud Events. The response will include the set of successfully released lock tokens, along
* with other failed lock tokens with their corresponding error information. Successfully released events can be
* received by consumers.
* Query Parameters
*
* Query Parameters
* Name Type Required Description
* releaseDelayInSeconds String No Release cloud events with the specified delay in
* seconds. Allowed values: "0", "10", "60", "600", "3600".
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param releaseRequest The releaseRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Release operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response releaseWithResponse(String topicName, String eventSubscriptionName,
BinaryData releaseRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return service.releaseSync(this.getEndpoint(), this.getServiceVersion().getVersion(), topicName,
eventSubscriptionName, contentType, accept, releaseRequest, requestOptions, Context.NONE);
}
/**
* Reject a batch of Cloud Events. The response will include the set of successfully rejected lock tokens, along
* with other failed lock tokens with their corresponding error information. Successfully rejected events will be
* dead-lettered and can no longer be received by a consumer.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param rejectRequest The rejectRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Reject operation along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> rejectWithResponseAsync(String topicName, String eventSubscriptionName,
BinaryData rejectRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return FluxUtil.withContext(context -> service.reject(this.getEndpoint(), this.getServiceVersion().getVersion(),
topicName, eventSubscriptionName, contentType, accept, rejectRequest, requestOptions, context));
}
/**
* Reject a batch of Cloud Events. The response will include the set of successfully rejected lock tokens, along
* with other failed lock tokens with their corresponding error information. Successfully rejected events will be
* dead-lettered and can no longer be received by a consumer.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param rejectRequest The rejectRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the Reject operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response rejectWithResponse(String topicName, String eventSubscriptionName,
BinaryData rejectRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return service.rejectSync(this.getEndpoint(), this.getServiceVersion().getVersion(), topicName,
eventSubscriptionName, contentType, accept, rejectRequest, requestOptions, Context.NONE);
}
/**
* Renew locks for a batch of Cloud Events. The response will include the set of successfully renewed lock tokens,
* along with other failed lock tokens with their corresponding error information. Successfully renewed locks will
* ensure that the associated event is only available to the consumer that holds the renewed lock.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param renewLocksRequest The renewLocksRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the RenewLock operation along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> renewLocksWithResponseAsync(String topicName, String eventSubscriptionName,
BinaryData renewLocksRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.renewLocks(this.getEndpoint(), this.getServiceVersion().getVersion(),
topicName, eventSubscriptionName, contentType, accept, renewLocksRequest, requestOptions, context));
}
/**
* Renew locks for a batch of Cloud Events. The response will include the set of successfully renewed lock tokens,
* along with other failed lock tokens with their corresponding error information. Successfully renewed locks will
* ensure that the associated event is only available to the consumer that holds the renewed lock.
* Request Body Schema
*
*
* {@code
* {
* lockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* Response Body Schema
*
*
* {@code
* {
* failedLockTokens (Required): [
* (Required){
* lockToken: String (Required)
* error (Required): {
* code: String (Required)
* message: String (Required)
* target: String (Optional)
* details (Optional): [
* (recursive schema, see above)
* ]
* innererror (Optional): {
* code: String (Optional)
* innererror (Optional): (recursive schema, see innererror above)
* }
* }
* }
* ]
* succeededLockTokens (Required): [
* String (Required)
* ]
* }
* }
*
*
* @param topicName Topic Name.
* @param eventSubscriptionName Event Subscription Name.
* @param renewLocksRequest The renewLocksRequest parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the result of the RenewLock operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response renewLocksWithResponse(String topicName, String eventSubscriptionName,
BinaryData renewLocksRequest, RequestOptions requestOptions) {
final String contentType = "application/json";
final String accept = "application/json";
return service.renewLocksSync(this.getEndpoint(), this.getServiceVersion().getVersion(), topicName,
eventSubscriptionName, contentType, accept, renewLocksRequest, requestOptions, Context.NONE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy