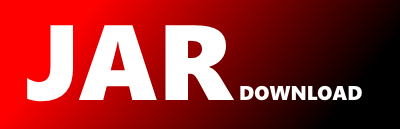
com.azure.messaging.eventgrid.namespaces.package-info Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
Show all versions of azure-messaging-eventgrid-namespaces Show documentation
This package contains the Microsoft Azure EventGrid Namespaces client library.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
/**
*
* Azure Event Grid allows you to easily build applications with event-based architectures. The Event Grid service fully
* manages all routing of events from any source, to any destination, for any application. Azure service events and
* custom events can be published directly to the service, where the events can then be filtered and sent to various
* recipients, such as built-in handlers or custom webhooks. To learn more about Azure Event Grid: What is Event Grid?
*
*
* Use the client library for Azure Event Grid Namespaces to:
*
* - Publish events to Event Grid topics using the Cloud Event schema
* - Consume and settle Cloud Events.
*
*
* Authentication
*
* In order to send events, we need an endpoint to send to and authentication for the endpoint. The authentication can
* be
* a key credential or an Entra ID credential. The endpoint and key can both be obtained through the Azure Portal or Azure CLI.
*
* Endpoint
*
* The endpoint is listed on the dashboard of the topic or domain in the Azure
* Portal,
* or can be obtained using the following command in Azure CLI:
*
* * az eventgrid topic show --name <your-resource-name> --resource-group <your-resource-group-name> --query "endpoint"
*
*
* Entra ID Token authentication
* Azure Event Grid provides integration with Entra ID for identity-based authentication of requests.
* With Entra ID, you can use role-based access control (RBAC) to grant access to your Azure Event Grid resources to
* users, groups, or applications.
* To send events to a topic or domain with a `TokenCredential`, the authenticated identity should have the "EventGrid
* Data Sender" role assigned.
*
* This authentication method is preferred.
*
*
*
*
* Access Key
*
* The keys are listed in the "Access Keys" tab of the Azure Portal, or can
* be
* obtained
* using the following command in Azure CLI. Anyone of the keys
* listed will work.
*
* * az eventgrid topic key list --name <your-resource-name> --resource-group <your-resource-group-name>
*
*
*
* * EventGridSenderClient client = new EventGridSenderClientBuilder().endpoint("your endpoint")
* .topicName("your topic")
* .credential(new AzureKeyCredential("your access key"))
* .buildClient();
*
*
*
* Key concepts
* Event Grid Namespace
* A namespace is a
* management container for other resources. It allows for grouping of related resources in order to manage them under
* one subscription.
* Namespace Topic
* A namespace
* topic is a topic that is created within an Event Grid namespace. The client publishes events to an HTTP namespace
* endpoint specifying a namespace topic where published events are logically contained. A namespace topic only supports
* the CloudEvent v1.0 schema.
* Event Subscription
* An event
* subscription is a configuration resource associated with a single topic.
*
* Examples
* Sending an event
*
* * EventGridSenderClient client = new EventGridSenderClientBuilder().endpoint("your endpoint")
* .topicName("your topic")
* .credential(new DefaultAzureCredentialBuilder().build())
* .buildClient();
*
*
*
* * User user = new User("John", "Doe");
* CloudEvent cloudEvent
* = new CloudEvent("source", "type", BinaryData.fromObject(user), CloudEventDataFormat.JSON, "application/json");
* client.send(cloudEvent);
*
*
*
* Receiving and Settling events
*
* *
* EventGridReceiverClient client = new EventGridReceiverClientBuilder().endpoint("your endpoint")
* .topicName("your topic")
* .subscriptionName("your subscription")
* .credential(new DefaultAzureCredentialBuilder().build())
* .buildClient();
*
* // Receive optionally takes a maximum number of events and a duration to wait. The defaults are
* // 1 event and 60 seconds.
* ReceiveResult result = client.receive(2, Duration.ofSeconds(10));
*
* // The result contains the received events and the details of the operation. Use the details to obtain
* // lock tokens for settling the event. Lock tokens are opaque strings that are used to acknowledge,
* // release, or reject the event.
*
* result.getDetails().forEach(details -> {
* CloudEvent event = details.getEvent();
* // Based on some examination of the event, it might be acknowledged, released, or rejected.
* User user = event.getData().toObject(User.class);
* if (user.getFirstName().equals("John")) {
* // Acknowledge the event.
* client.acknowledge(Arrays.asList(details.getBrokerProperties().getLockToken()));
* } else if (user.getFirstName().equals("Jane")) {
* // Release the event.
* client.release(Arrays.asList(details.getBrokerProperties().getLockToken()));
* } else {
* // Reject the event.
* client.reject(Arrays.asList(details.getBrokerProperties().getLockToken()));
* }
* });
*
*
*
*
*/
package com.azure.messaging.eventgrid.namespaces;