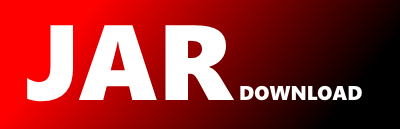
com.azure.messaging.eventgrid.EventGridPublisherClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.messaging.eventgrid;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.credential.AzureKeyCredential;
import com.azure.core.credential.AzureSasCredential;
import com.azure.core.http.rest.Response;
import com.azure.core.models.CloudEvent;
import com.azure.core.util.Context;
import java.time.OffsetDateTime;
/**
* A service client that publishes events to an EventGrid topic or domain. Use {@link EventGridPublisherClientBuilder}
* to create an instance of this client. Note that this is simply a synchronous convenience layer over the
* {@link EventGridPublisherAsyncClient}, which has more efficient asynchronous functionality and is recommended.
*
* Create EventGridPublisherClient for CloudEvent Samples
*
*
* // Create a client to send events of CloudEvent schema (com.azure.core.models.CloudEvent)
* EventGridPublisherClient<CloudEvent> cloudEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_CLOUDEVENT_ENDPOINT")) // make sure it accepts CloudEvent
* .credential(new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_CLOUDEVENT_KEY")))
* .buildCloudEventPublisherClient();
*
*
*
* Send CloudEvent Samples
*
*
* // Create a com.azure.models.CloudEvent.
* User user = new User("Stephen", "James");
* CloudEvent cloudEventDataObject = new CloudEvent("/cloudevents/example/source", "Example.EventType",
* BinaryData.fromObject(user), CloudEventDataFormat.JSON, "application/json");
*
* // Send a single CloudEvent
* cloudEventPublisherClient.sendEvent(cloudEventDataObject);
*
* // Send a list of CloudEvents to the EventGrid service altogether.
* // This has better performance than sending one by one.
* cloudEventPublisherClient.sendEvents(Arrays.asList(
* cloudEventDataObject
* // add more CloudEvents objects
* ));
*
*
*
* Create EventGridPublisherClient for EventGridEvent Samples
*
*
* // Create a client to send events of EventGridEvent schema
* EventGridPublisherClient<EventGridEvent> eventGridEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT")) // make sure it accepts EventGridEvent
* .credential(new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_EVENT_KEY")))
* .buildEventGridEventPublisherClient();
*
*
*
* Send EventGridEvent Samples
*
*
* // Create an EventGridEvent
* User user = new User("John", "James");
* EventGridEvent eventGridEvent = new EventGridEvent("/EventGridEvents/example/source",
* "Example.EventType", BinaryData.fromObject(user), "0.1");
*
* // Send a single EventGridEvent
* eventGridEventPublisherClient.sendEvent(eventGridEvent);
*
* // Send a list of EventGridEvents to the EventGrid service altogether.
* // This has better performance than sending one by one.
* eventGridEventPublisherClient.sendEvents(Arrays.asList(
* eventGridEvent
* // add more EventGridEvents objects
* ));
*
*
*
* Create EventGridPublisherClient for Custom Event Schema Samples
*
*
* // Create a client to send events of custom event
* EventGridPublisherClient<BinaryData> customEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_CUSTOM_EVENT_ENDPOINT")) // make sure it accepts custom events
* .credential(new AzureKeyCredential(System.getenv("AZURE_CUSTOM_EVENT_KEY")))
* .buildCustomEventPublisherClient();
*
*
*
* Send Custom Event Schema Samples
*
*
* // Create an custom event object
* Map<String, Object> customEvent = new HashMap<String, Object>() {
* {
* put("id", UUID.randomUUID().toString());
* put("subject", "Test");
* put("foo", "bar");
* put("type", "Microsoft.MockPublisher.TestEvent");
* put("data", 100.0);
* put("dataVersion", "0.1");
* }
* };
*
* // Send a single custom event
* customEventPublisherClient.sendEvent(BinaryData.fromObject(customEvent));
*
* // Send a list of custom events to the EventGrid service altogether.
* // This has better performance than sending one by one.
* customEventPublisherClient.sendEvents(Arrays.asList(
* BinaryData.fromObject(customEvent)
* // add more custom events in BinaryData
* ));
*
*
*
* @see EventGridEvent
* @see CloudEvent
*/
@ServiceClient(builder = EventGridPublisherClientBuilder.class)
public final class EventGridPublisherClient {
private final EventGridPublisherAsyncClient asyncClient;
EventGridPublisherClient(EventGridPublisherAsyncClient client) {
this.asyncClient = client;
}
/**
* Generate a shared access signature to provide time-limited authentication for requests to the Event Grid
* service with the latest Event Grid service API defined in {@link EventGridServiceVersion#getLatest()}.
* @param endpoint the endpoint of the Event Grid topic or domain.
* @param expirationTime the time in which the signature should expire, no longer providing authentication.
* @param keyCredential the access key obtained from the Event Grid topic or domain.
*
* @return the shared access signature string which can be used to construct an instance of
* {@link AzureSasCredential}.
*
* @throws NullPointerException if keyCredential or expirationTime is {@code null}.
* @throws RuntimeException if java security doesn't have algorithm "hmacSHA256".
*/
public static String generateSas(String endpoint, AzureKeyCredential keyCredential, OffsetDateTime expirationTime) {
return EventGridPublisherAsyncClient.generateSas(endpoint, keyCredential, expirationTime,
EventGridServiceVersion.getLatest());
}
/**
* Generate a shared access signature to provide time-limited authentication for requests to the Event Grid
* service.
* @param endpoint the endpoint of the Event Grid topic or domain.
* @param expirationTime the time in which the signature should expire, no longer providing authentication.
* @param keyCredential the access key obtained from the Event Grid topic or domain.
* @param apiVersion the EventGrid service api version defined in {@link EventGridServiceVersion}
*
* @return the shared access signature string which can be used to construct an instance of
* {@link AzureSasCredential}.
*
* @throws NullPointerException if keyCredential or expirationTime is {@code null}.
* @throws RuntimeException if java security doesn't have algorithm "hmacSHA256".
*/
public static String generateSas(String endpoint, AzureKeyCredential keyCredential, OffsetDateTime expirationTime,
EventGridServiceVersion apiVersion) {
return EventGridPublisherAsyncClient.generateSas(endpoint, keyCredential, expirationTime, apiVersion);
}
/**
* Publishes the given events to the given topic or domain.
* @param events the cloud events to publish.
* @throws NullPointerException if events is {@code null}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void sendEvents(Iterable events) {
asyncClient.sendEvents(events).block();
}
/**
* Publishes the given events to the set topic or domain and gives the response issued by EventGrid.
* @param events the events to publish.
* @param context the context to use along the pipeline.
*
* @return the response from the EventGrid service.
* @throws NullPointerException if events is {@code null}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response sendEventsWithResponse(Iterable events, Context context) {
return asyncClient.sendEventsWithResponse(events, context).block();
}
/**
* Publishes the given events to the set topic or domain and gives the response issued by EventGrid.
* @param events the events to publish.
* @param channelName the channel name to send to Event Grid service. This is only applicable for sending
* Cloud Events to a partner topic in partner namespace. For more details, refer to
* Partner Events Overview.
* @param context the context to use along the pipeline.
*
* @return the response from the EventGrid service.
* @throws NullPointerException if events is {@code null}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response sendEventsWithResponse(Iterable events, String channelName,
Context context) {
return asyncClient.sendEventsWithResponse(events, channelName, context).block();
}
/**
* Publishes the given event to the set topic or domain and gives the response issued by EventGrid.
* @param event the event to publish.
*
* @throws NullPointerException if events is {@code null}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void sendEvent(T event) {
asyncClient.sendEvent(event).block();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy