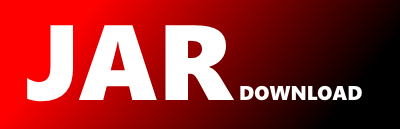
com.azure.messaging.eventgrid.systemevents.AcsSmsDeliveryReportReceivedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.List;
/** Schema of the Data property of an EventGridEvent for a Microsoft.Communication.SMSDeliveryReportReceived event. */
@Fluent
public final class AcsSmsDeliveryReportReceivedEventData extends AcsSmsEventBaseProperties {
/*
* Status of Delivery
*/
@JsonProperty(value = "deliveryStatus")
private String deliveryStatus;
/*
* Details about Delivery Status
*/
@JsonProperty(value = "deliveryStatusDetails")
private String deliveryStatusDetails;
/*
* List of details of delivery attempts made
*/
@JsonProperty(value = "deliveryAttempts")
private List deliveryAttempts;
/*
* The time at which the SMS delivery report was received
*/
@JsonProperty(value = "receivedTimestamp")
private OffsetDateTime receivedTimestamp;
/*
* Customer Content
*/
@JsonProperty(value = "tag")
private String tag;
/** Creates an instance of AcsSmsDeliveryReportReceivedEventData class. */
public AcsSmsDeliveryReportReceivedEventData() {}
/**
* Get the deliveryStatus property: Status of Delivery.
*
* @return the deliveryStatus value.
*/
public String getDeliveryStatus() {
return this.deliveryStatus;
}
/**
* Set the deliveryStatus property: Status of Delivery.
*
* @param deliveryStatus the deliveryStatus value to set.
* @return the AcsSmsDeliveryReportReceivedEventData object itself.
*/
public AcsSmsDeliveryReportReceivedEventData setDeliveryStatus(String deliveryStatus) {
this.deliveryStatus = deliveryStatus;
return this;
}
/**
* Get the deliveryStatusDetails property: Details about Delivery Status.
*
* @return the deliveryStatusDetails value.
*/
public String getDeliveryStatusDetails() {
return this.deliveryStatusDetails;
}
/**
* Set the deliveryStatusDetails property: Details about Delivery Status.
*
* @param deliveryStatusDetails the deliveryStatusDetails value to set.
* @return the AcsSmsDeliveryReportReceivedEventData object itself.
*/
public AcsSmsDeliveryReportReceivedEventData setDeliveryStatusDetails(String deliveryStatusDetails) {
this.deliveryStatusDetails = deliveryStatusDetails;
return this;
}
/**
* Get the deliveryAttempts property: List of details of delivery attempts made.
*
* @return the deliveryAttempts value.
*/
public List getDeliveryAttempts() {
return this.deliveryAttempts;
}
/**
* Set the deliveryAttempts property: List of details of delivery attempts made.
*
* @param deliveryAttempts the deliveryAttempts value to set.
* @return the AcsSmsDeliveryReportReceivedEventData object itself.
*/
public AcsSmsDeliveryReportReceivedEventData setDeliveryAttempts(
List deliveryAttempts) {
this.deliveryAttempts = deliveryAttempts;
return this;
}
/**
* Get the receivedTimestamp property: The time at which the SMS delivery report was received.
*
* @return the receivedTimestamp value.
*/
public OffsetDateTime getReceivedTimestamp() {
return this.receivedTimestamp;
}
/**
* Set the receivedTimestamp property: The time at which the SMS delivery report was received.
*
* @param receivedTimestamp the receivedTimestamp value to set.
* @return the AcsSmsDeliveryReportReceivedEventData object itself.
*/
public AcsSmsDeliveryReportReceivedEventData setReceivedTimestamp(OffsetDateTime receivedTimestamp) {
this.receivedTimestamp = receivedTimestamp;
return this;
}
/**
* Get the tag property: Customer Content.
*
* @return the tag value.
*/
public String getTag() {
return this.tag;
}
/**
* Set the tag property: Customer Content.
*
* @param tag the tag value to set.
* @return the AcsSmsDeliveryReportReceivedEventData object itself.
*/
public AcsSmsDeliveryReportReceivedEventData setTag(String tag) {
this.tag = tag;
return this;
}
/** {@inheritDoc} */
@Override
public AcsSmsDeliveryReportReceivedEventData setMessageId(String messageId) {
super.setMessageId(messageId);
return this;
}
/** {@inheritDoc} */
@Override
public AcsSmsDeliveryReportReceivedEventData setFrom(String from) {
super.setFrom(from);
return this;
}
/** {@inheritDoc} */
@Override
public AcsSmsDeliveryReportReceivedEventData setTo(String to) {
super.setTo(to);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy