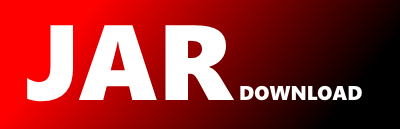
com.azure.messaging.eventgrid.systemevents.MachineLearningServicesModelDeployedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
/** Schema of the Data property of an EventGridEvent for a Microsoft.MachineLearningServices.ModelDeployed event. */
@Fluent
public final class MachineLearningServicesModelDeployedEventData {
/*
* The name of the deployed service.
*/
@JsonProperty(value = "serviceName")
private String serviceName;
/*
* The compute type (e.g. ACI, AKS) of the deployed service.
*/
@JsonProperty(value = "serviceComputeType")
private String serviceComputeType;
/*
* A common separated list of model IDs. The IDs of the models deployed in the service.
*/
@JsonProperty(value = "modelIds")
private String modelIds;
/*
* The tags of the deployed service.
*/
@JsonProperty(value = "serviceTags")
private Object serviceTags;
/*
* The properties of the deployed service.
*/
@JsonProperty(value = "serviceProperties")
private Object serviceProperties;
/** Creates an instance of MachineLearningServicesModelDeployedEventData class. */
public MachineLearningServicesModelDeployedEventData() {}
/**
* Get the serviceName property: The name of the deployed service.
*
* @return the serviceName value.
*/
public String getServiceName() {
return this.serviceName;
}
/**
* Set the serviceName property: The name of the deployed service.
*
* @param serviceName the serviceName value to set.
* @return the MachineLearningServicesModelDeployedEventData object itself.
*/
public MachineLearningServicesModelDeployedEventData setServiceName(String serviceName) {
this.serviceName = serviceName;
return this;
}
/**
* Get the serviceComputeType property: The compute type (e.g. ACI, AKS) of the deployed service.
*
* @return the serviceComputeType value.
*/
public String getServiceComputeType() {
return this.serviceComputeType;
}
/**
* Set the serviceComputeType property: The compute type (e.g. ACI, AKS) of the deployed service.
*
* @param serviceComputeType the serviceComputeType value to set.
* @return the MachineLearningServicesModelDeployedEventData object itself.
*/
public MachineLearningServicesModelDeployedEventData setServiceComputeType(String serviceComputeType) {
this.serviceComputeType = serviceComputeType;
return this;
}
/**
* Get the modelIds property: A common separated list of model IDs. The IDs of the models deployed in the service.
*
* @return the modelIds value.
*/
public String getModelIds() {
return this.modelIds;
}
/**
* Set the modelIds property: A common separated list of model IDs. The IDs of the models deployed in the service.
*
* @param modelIds the modelIds value to set.
* @return the MachineLearningServicesModelDeployedEventData object itself.
*/
public MachineLearningServicesModelDeployedEventData setModelIds(String modelIds) {
this.modelIds = modelIds;
return this;
}
/**
* Get the serviceTags property: The tags of the deployed service.
*
* @return the serviceTags value.
*/
public Object getServiceTags() {
return this.serviceTags;
}
/**
* Set the serviceTags property: The tags of the deployed service.
*
* @param serviceTags the serviceTags value to set.
* @return the MachineLearningServicesModelDeployedEventData object itself.
*/
public MachineLearningServicesModelDeployedEventData setServiceTags(Object serviceTags) {
this.serviceTags = serviceTags;
return this;
}
/**
* Get the serviceProperties property: The properties of the deployed service.
*
* @return the serviceProperties value.
*/
public Object getServiceProperties() {
return this.serviceProperties;
}
/**
* Set the serviceProperties property: The properties of the deployed service.
*
* @param serviceProperties the serviceProperties value to set.
* @return the MachineLearningServicesModelDeployedEventData object itself.
*/
public MachineLearningServicesModelDeployedEventData setServiceProperties(Object serviceProperties) {
this.serviceProperties = serviceProperties;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy