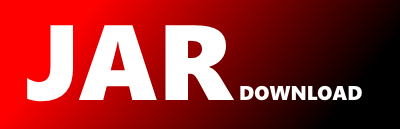
com.azure.messaging.eventgrid.systemevents.MediaJobOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.JsonFlatten;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
/** The event data for a Job output. */
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "@odata\\.type",
defaultImpl = MediaJobOutput.class)
@JsonTypeName("MediaJobOutput")
@JsonSubTypes({@JsonSubTypes.Type(name = "#Microsoft.Media.JobOutputAsset", value = MediaJobOutputAsset.class)})
@JsonFlatten
@Fluent
public class MediaJobOutput {
/*
* Gets the Job output error.
*/
@JsonProperty(value = "error")
private MediaJobError error;
/*
* Gets the Job output label.
*/
@JsonProperty(value = "label")
private String label;
/*
* Gets the Job output progress.
*/
@JsonProperty(value = "progress", required = true)
private long progress;
/*
* Gets the Job output state.
*/
@JsonProperty(value = "state", required = true)
private MediaJobState state;
/** Creates an instance of MediaJobOutput class. */
public MediaJobOutput() {}
/**
* Get the error property: Gets the Job output error.
*
* @return the error value.
*/
public MediaJobError getError() {
return this.error;
}
/**
* Set the error property: Gets the Job output error.
*
* @param error the error value to set.
* @return the MediaJobOutput object itself.
*/
public MediaJobOutput setError(MediaJobError error) {
this.error = error;
return this;
}
/**
* Get the label property: Gets the Job output label.
*
* @return the label value.
*/
public String getLabel() {
return this.label;
}
/**
* Set the label property: Gets the Job output label.
*
* @param label the label value to set.
* @return the MediaJobOutput object itself.
*/
public MediaJobOutput setLabel(String label) {
this.label = label;
return this;
}
/**
* Get the progress property: Gets the Job output progress.
*
* @return the progress value.
*/
public long getProgress() {
return this.progress;
}
/**
* Set the progress property: Gets the Job output progress.
*
* @param progress the progress value to set.
* @return the MediaJobOutput object itself.
*/
public MediaJobOutput setProgress(long progress) {
this.progress = progress;
return this;
}
/**
* Get the state property: Gets the Job output state.
*
* @return the state value.
*/
public MediaJobState getState() {
return this.state;
}
/**
* Set the state property: Gets the Job output state.
*
* @param state the state value to set.
* @return the MediaJobOutput object itself.
*/
public MediaJobOutput setState(MediaJobState state) {
this.state = state;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy