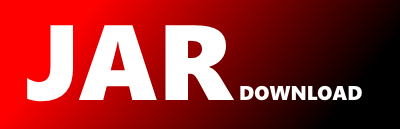
com.azure.messaging.eventgrid.systemevents.MediaLiveEventIncomingStreamReceivedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Immutable;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Encoder connect event data. Schema of the data property of an EventGridEvent for a
* Microsoft.Media.LiveEventIncomingStreamReceived event.
*/
@Immutable
public final class MediaLiveEventIncomingStreamReceivedEventData {
/*
* Gets the ingest URL provided by the live event.
*/
@JsonProperty(value = "ingestUrl", access = JsonProperty.Access.WRITE_ONLY)
private String ingestUrl;
/*
* Gets the type of the track (Audio / Video).
*/
@JsonProperty(value = "trackType", access = JsonProperty.Access.WRITE_ONLY)
private String trackType;
/*
* Gets the track name.
*/
@JsonProperty(value = "trackName", access = JsonProperty.Access.WRITE_ONLY)
private String trackName;
/*
* Gets the bitrate of the track.
*/
@JsonProperty(value = "bitrate", access = JsonProperty.Access.WRITE_ONLY)
private Long bitrate;
/*
* Gets the remote IP.
*/
@JsonProperty(value = "encoderIp", access = JsonProperty.Access.WRITE_ONLY)
private String encoderIp;
/*
* Gets the remote port.
*/
@JsonProperty(value = "encoderPort", access = JsonProperty.Access.WRITE_ONLY)
private String encoderPort;
/*
* Gets the first timestamp of the data chunk received.
*/
@JsonProperty(value = "timestamp", access = JsonProperty.Access.WRITE_ONLY)
private String timestamp;
/*
* Gets the duration of the first data chunk.
*/
@JsonProperty(value = "duration", access = JsonProperty.Access.WRITE_ONLY)
private String duration;
/*
* Gets the timescale in which timestamp is represented.
*/
@JsonProperty(value = "timescale", access = JsonProperty.Access.WRITE_ONLY)
private String timescale;
/** Creates an instance of MediaLiveEventIncomingStreamReceivedEventData class. */
public MediaLiveEventIncomingStreamReceivedEventData() {}
/**
* Get the ingestUrl property: Gets the ingest URL provided by the live event.
*
* @return the ingestUrl value.
*/
public String getIngestUrl() {
return this.ingestUrl;
}
/**
* Get the trackType property: Gets the type of the track (Audio / Video).
*
* @return the trackType value.
*/
public String getTrackType() {
return this.trackType;
}
/**
* Get the trackName property: Gets the track name.
*
* @return the trackName value.
*/
public String getTrackName() {
return this.trackName;
}
/**
* Get the bitrate property: Gets the bitrate of the track.
*
* @return the bitrate value.
*/
public Long getBitrate() {
return this.bitrate;
}
/**
* Get the encoderIp property: Gets the remote IP.
*
* @return the encoderIp value.
*/
public String getEncoderIp() {
return this.encoderIp;
}
/**
* Get the encoderPort property: Gets the remote port.
*
* @return the encoderPort value.
*/
public String getEncoderPort() {
return this.encoderPort;
}
/**
* Get the timestamp property: Gets the first timestamp of the data chunk received.
*
* @return the timestamp value.
*/
public String getTimestamp() {
return this.timestamp;
}
/**
* Get the duration property: Gets the duration of the first data chunk.
*
* @return the duration value.
*/
public String getDuration() {
return this.duration;
}
/**
* Get the timescale property: Gets the timescale in which timestamp is represented.
*
* @return the timescale value.
*/
public String getTimescale() {
return this.timescale;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy