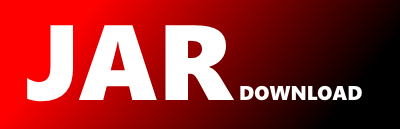
com.azure.messaging.eventgrid.systemevents.DeviceTelemetryEventProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.Map;
/**
* Schema of the Data property of an EventGridEvent for a device telemetry event (DeviceTelemetry).
*/
@Fluent
public class DeviceTelemetryEventProperties implements JsonSerializable {
/*
* The content of the message from the device.
*/
private Object body;
/*
* Application properties are user-defined strings that can be added to the message. These fields are optional.
*/
private Map properties;
/*
* System properties help identify contents and source of the messages.
*/
private Map systemProperties;
/**
* Creates an instance of DeviceTelemetryEventProperties class.
*/
public DeviceTelemetryEventProperties() {
}
/**
* Get the body property: The content of the message from the device.
*
* @return the body value.
*/
public Object getBody() {
return this.body;
}
/**
* Set the body property: The content of the message from the device.
*
* @param body the body value to set.
* @return the DeviceTelemetryEventProperties object itself.
*/
public DeviceTelemetryEventProperties setBody(Object body) {
this.body = body;
return this;
}
/**
* Get the properties property: Application properties are user-defined strings that can be added to the message.
* These fields are optional.
*
* @return the properties value.
*/
public Map getProperties() {
return this.properties;
}
/**
* Set the properties property: Application properties are user-defined strings that can be added to the message.
* These fields are optional.
*
* @param properties the properties value to set.
* @return the DeviceTelemetryEventProperties object itself.
*/
public DeviceTelemetryEventProperties setProperties(Map properties) {
this.properties = properties;
return this;
}
/**
* Get the systemProperties property: System properties help identify contents and source of the messages.
*
* @return the systemProperties value.
*/
public Map getSystemProperties() {
return this.systemProperties;
}
/**
* Set the systemProperties property: System properties help identify contents and source of the messages.
*
* @param systemProperties the systemProperties value to set.
* @return the DeviceTelemetryEventProperties object itself.
*/
public DeviceTelemetryEventProperties setSystemProperties(Map systemProperties) {
this.systemProperties = systemProperties;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeUntypedField("body", this.body);
jsonWriter.writeMapField("properties", this.properties, (writer, element) -> writer.writeString(element));
jsonWriter.writeMapField("systemProperties", this.systemProperties,
(writer, element) -> writer.writeString(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DeviceTelemetryEventProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DeviceTelemetryEventProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the DeviceTelemetryEventProperties.
*/
public static DeviceTelemetryEventProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DeviceTelemetryEventProperties deserializedDeviceTelemetryEventProperties
= new DeviceTelemetryEventProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("body".equals(fieldName)) {
deserializedDeviceTelemetryEventProperties.body = reader.readUntyped();
} else if ("properties".equals(fieldName)) {
Map properties = reader.readMap(reader1 -> reader1.getString());
deserializedDeviceTelemetryEventProperties.properties = properties;
} else if ("systemProperties".equals(fieldName)) {
Map systemProperties = reader.readMap(reader1 -> reader1.getString());
deserializedDeviceTelemetryEventProperties.systemProperties = systemProperties;
} else {
reader.skipChildren();
}
}
return deserializedDeviceTelemetryEventProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy