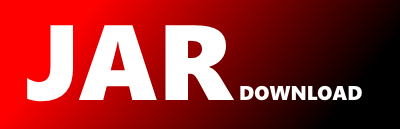
com.azure.messaging.eventgrid.systemevents.AcsRouterWorkerUpdatedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
import java.util.Map;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.Communication.RouterWorkerUpdated event.
*/
@Fluent
public final class AcsRouterWorkerUpdatedEventData implements JsonSerializable {
/*
* Router Worker Updated Worker Id
*/
private String workerId;
/*
* Router Worker Updated Queue Info
*/
private List queueAssignments;
/*
* Router Worker Updated Channel Configuration
*/
private List channelConfigurations;
/*
* Router Worker Updated Total Capacity
*/
private Integer totalCapacity;
/*
* Router Worker Updated Labels
*/
private Map labels;
/*
* Router Worker Updated Tags
*/
private Map tags;
/*
* Router Worker Properties Updated
*/
private List updatedWorkerProperties;
/**
* Creates an instance of AcsRouterWorkerUpdatedEventData class.
*/
public AcsRouterWorkerUpdatedEventData() {
}
/**
* Get the workerId property: Router Worker Updated Worker Id.
*
* @return the workerId value.
*/
public String getWorkerId() {
return this.workerId;
}
/**
* Set the workerId property: Router Worker Updated Worker Id.
*
* @param workerId the workerId value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData setWorkerId(String workerId) {
this.workerId = workerId;
return this;
}
/**
* Get the queueAssignments property: Router Worker Updated Queue Info.
*
* @return the queueAssignments value.
*/
public List getQueueAssignments() {
return this.queueAssignments;
}
/**
* Set the queueAssignments property: Router Worker Updated Queue Info.
*
* @param queueAssignments the queueAssignments value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData setQueueAssignments(List queueAssignments) {
this.queueAssignments = queueAssignments;
return this;
}
/**
* Get the channelConfigurations property: Router Worker Updated Channel Configuration.
*
* @return the channelConfigurations value.
*/
public List getChannelConfigurations() {
return this.channelConfigurations;
}
/**
* Set the channelConfigurations property: Router Worker Updated Channel Configuration.
*
* @param channelConfigurations the channelConfigurations value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData
setChannelConfigurations(List channelConfigurations) {
this.channelConfigurations = channelConfigurations;
return this;
}
/**
* Get the totalCapacity property: Router Worker Updated Total Capacity.
*
* @return the totalCapacity value.
*/
public Integer getTotalCapacity() {
return this.totalCapacity;
}
/**
* Set the totalCapacity property: Router Worker Updated Total Capacity.
*
* @param totalCapacity the totalCapacity value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData setTotalCapacity(Integer totalCapacity) {
this.totalCapacity = totalCapacity;
return this;
}
/**
* Get the labels property: Router Worker Updated Labels.
*
* @return the labels value.
*/
public Map getLabels() {
return this.labels;
}
/**
* Set the labels property: Router Worker Updated Labels.
*
* @param labels the labels value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData setLabels(Map labels) {
this.labels = labels;
return this;
}
/**
* Get the tags property: Router Worker Updated Tags.
*
* @return the tags value.
*/
public Map getTags() {
return this.tags;
}
/**
* Set the tags property: Router Worker Updated Tags.
*
* @param tags the tags value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData setTags(Map tags) {
this.tags = tags;
return this;
}
/**
* Get the updatedWorkerProperties property: Router Worker Properties Updated.
*
* @return the updatedWorkerProperties value.
*/
public List getUpdatedWorkerProperties() {
return this.updatedWorkerProperties;
}
/**
* Set the updatedWorkerProperties property: Router Worker Properties Updated.
*
* @param updatedWorkerProperties the updatedWorkerProperties value to set.
* @return the AcsRouterWorkerUpdatedEventData object itself.
*/
public AcsRouterWorkerUpdatedEventData
setUpdatedWorkerProperties(List updatedWorkerProperties) {
this.updatedWorkerProperties = updatedWorkerProperties;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("workerId", this.workerId);
jsonWriter.writeArrayField("queueAssignments", this.queueAssignments,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("channelConfigurations", this.channelConfigurations,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeNumberField("totalCapacity", this.totalCapacity);
jsonWriter.writeMapField("labels", this.labels, (writer, element) -> writer.writeString(element));
jsonWriter.writeMapField("tags", this.tags, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("updatedWorkerProperties", this.updatedWorkerProperties,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AcsRouterWorkerUpdatedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AcsRouterWorkerUpdatedEventData if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AcsRouterWorkerUpdatedEventData.
*/
public static AcsRouterWorkerUpdatedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AcsRouterWorkerUpdatedEventData deserializedAcsRouterWorkerUpdatedEventData
= new AcsRouterWorkerUpdatedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("workerId".equals(fieldName)) {
deserializedAcsRouterWorkerUpdatedEventData.workerId = reader.getString();
} else if ("queueAssignments".equals(fieldName)) {
List queueAssignments
= reader.readArray(reader1 -> AcsRouterQueueDetails.fromJson(reader1));
deserializedAcsRouterWorkerUpdatedEventData.queueAssignments = queueAssignments;
} else if ("channelConfigurations".equals(fieldName)) {
List channelConfigurations
= reader.readArray(reader1 -> AcsRouterChannelConfiguration.fromJson(reader1));
deserializedAcsRouterWorkerUpdatedEventData.channelConfigurations = channelConfigurations;
} else if ("totalCapacity".equals(fieldName)) {
deserializedAcsRouterWorkerUpdatedEventData.totalCapacity = reader.getNullable(JsonReader::getInt);
} else if ("labels".equals(fieldName)) {
Map labels = reader.readMap(reader1 -> reader1.getString());
deserializedAcsRouterWorkerUpdatedEventData.labels = labels;
} else if ("tags".equals(fieldName)) {
Map tags = reader.readMap(reader1 -> reader1.getString());
deserializedAcsRouterWorkerUpdatedEventData.tags = tags;
} else if ("updatedWorkerProperties".equals(fieldName)) {
List updatedWorkerProperties
= reader.readArray(reader1 -> AcsRouterUpdatedWorkerProperty.fromString(reader1.getString()));
deserializedAcsRouterWorkerUpdatedEventData.updatedWorkerProperties = updatedWorkerProperties;
} else {
reader.skipChildren();
}
}
return deserializedAcsRouterWorkerUpdatedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy