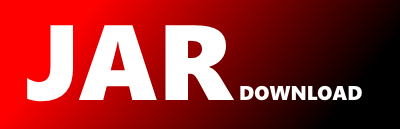
com.azure.messaging.eventgrid.systemevents.ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of the Data property of an EventGridEvent for a
* Microsoft.ServiceBus.ActiveMessagesAvailablePeriodicNotifications event.
*/
@Fluent
public final class ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData
implements JsonSerializable {
/*
* The namespace name of the Microsoft.ServiceBus resource.
*/
private String namespaceName;
/*
* The endpoint of the Microsoft.ServiceBus resource.
*/
private String requestUri;
/*
* The entity type of the Microsoft.ServiceBus resource. Could be one of 'queue' or 'subscriber'.
*/
private String entityType;
/*
* The name of the Microsoft.ServiceBus queue. If the entity type is of type 'subscriber', then this value will be
* null.
*/
private String queueName;
/*
* The name of the Microsoft.ServiceBus topic. If the entity type is of type 'queue', then this value will be null.
*/
private String topicName;
/*
* The name of the Microsoft.ServiceBus topic's subscription. If the entity type is of type 'queue', then this
* value will be null.
*/
private String subscriptionName;
/**
* Creates an instance of ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData class.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData() {
}
/**
* Get the namespaceName property: The namespace name of the Microsoft.ServiceBus resource.
*
* @return the namespaceName value.
*/
public String getNamespaceName() {
return this.namespaceName;
}
/**
* Set the namespaceName property: The namespace name of the Microsoft.ServiceBus resource.
*
* @param namespaceName the namespaceName value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData setNamespaceName(String namespaceName) {
this.namespaceName = namespaceName;
return this;
}
/**
* Get the requestUri property: The endpoint of the Microsoft.ServiceBus resource.
*
* @return the requestUri value.
*/
public String getRequestUri() {
return this.requestUri;
}
/**
* Set the requestUri property: The endpoint of the Microsoft.ServiceBus resource.
*
* @param requestUri the requestUri value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData setRequestUri(String requestUri) {
this.requestUri = requestUri;
return this;
}
/**
* Get the entityType property: The entity type of the Microsoft.ServiceBus resource. Could be one of 'queue' or
* 'subscriber'.
*
* @return the entityType value.
*/
public String getEntityType() {
return this.entityType;
}
/**
* Set the entityType property: The entity type of the Microsoft.ServiceBus resource. Could be one of 'queue' or
* 'subscriber'.
*
* @param entityType the entityType value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData setEntityType(String entityType) {
this.entityType = entityType;
return this;
}
/**
* Get the queueName property: The name of the Microsoft.ServiceBus queue. If the entity type is of type
* 'subscriber', then this value will be null.
*
* @return the queueName value.
*/
public String getQueueName() {
return this.queueName;
}
/**
* Set the queueName property: The name of the Microsoft.ServiceBus queue. If the entity type is of type
* 'subscriber', then this value will be null.
*
* @param queueName the queueName value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData setQueueName(String queueName) {
this.queueName = queueName;
return this;
}
/**
* Get the topicName property: The name of the Microsoft.ServiceBus topic. If the entity type is of type 'queue',
* then this value will be null.
*
* @return the topicName value.
*/
public String getTopicName() {
return this.topicName;
}
/**
* Set the topicName property: The name of the Microsoft.ServiceBus topic. If the entity type is of type 'queue',
* then this value will be null.
*
* @param topicName the topicName value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData setTopicName(String topicName) {
this.topicName = topicName;
return this;
}
/**
* Get the subscriptionName property: The name of the Microsoft.ServiceBus topic's subscription. If the entity type
* is of type 'queue', then this value will be null.
*
* @return the subscriptionName value.
*/
public String getSubscriptionName() {
return this.subscriptionName;
}
/**
* Set the subscriptionName property: The name of the Microsoft.ServiceBus topic's subscription. If the entity type
* is of type 'queue', then this value will be null.
*
* @param subscriptionName the subscriptionName value to set.
* @return the ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData object itself.
*/
public ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData
setSubscriptionName(String subscriptionName) {
this.subscriptionName = subscriptionName;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("namespaceName", this.namespaceName);
jsonWriter.writeStringField("requestUri", this.requestUri);
jsonWriter.writeStringField("entityType", this.entityType);
jsonWriter.writeStringField("queueName", this.queueName);
jsonWriter.writeStringField("topicName", this.topicName);
jsonWriter.writeStringField("subscriptionName", this.subscriptionName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData if the JsonReader was
* pointing to an instance of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the
* ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.
*/
public static ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData fromJson(JsonReader jsonReader)
throws IOException {
return jsonReader.readObject(reader -> {
ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData
= new ServiceBusActiveMessagesAvailablePeriodicNotificationsEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("namespaceName".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.namespaceName
= reader.getString();
} else if ("requestUri".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.requestUri
= reader.getString();
} else if ("entityType".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.entityType
= reader.getString();
} else if ("queueName".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.queueName
= reader.getString();
} else if ("topicName".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.topicName
= reader.getString();
} else if ("subscriptionName".equals(fieldName)) {
deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData.subscriptionName
= reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedServiceBusActiveMessagesAvailablePeriodicNotificationsEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy