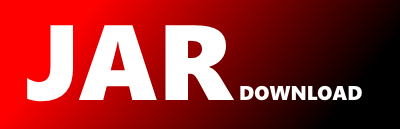
com.azure.messaging.eventgrid.implementation.models.EventGridEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
import java.time.temporal.TemporalAccessor;
import java.time.temporal.TemporalQueries;
/**
* Properties of an event published to an Event Grid topic using the EventGrid Schema.
*/
@Fluent
public final class EventGridEvent implements JsonSerializable {
/*
* An unique identifier for the event.
*/
private String id;
/*
* The resource path of the event source.
*/
private String topic;
/*
* A resource path relative to the topic path.
*/
private String subject;
/*
* Event data specific to the event type.
*/
private Object data;
/*
* The type of the event that occurred.
*/
private String eventType;
/*
* The time (in UTC) the event was generated.
*/
private OffsetDateTime eventTime;
/*
* The schema version of the event metadata.
*/
private String metadataVersion;
/*
* The schema version of the data object.
*/
private String dataVersion;
/**
* Creates an instance of EventGridEvent class.
*/
public EventGridEvent() {
}
/**
* Get the id property: An unique identifier for the event.
*
* @return the id value.
*/
public String getId() {
return this.id;
}
/**
* Set the id property: An unique identifier for the event.
*
* @param id the id value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setId(String id) {
this.id = id;
return this;
}
/**
* Get the topic property: The resource path of the event source.
*
* @return the topic value.
*/
public String getTopic() {
return this.topic;
}
/**
* Set the topic property: The resource path of the event source.
*
* @param topic the topic value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setTopic(String topic) {
this.topic = topic;
return this;
}
/**
* Get the subject property: A resource path relative to the topic path.
*
* @return the subject value.
*/
public String getSubject() {
return this.subject;
}
/**
* Set the subject property: A resource path relative to the topic path.
*
* @param subject the subject value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setSubject(String subject) {
this.subject = subject;
return this;
}
/**
* Get the data property: Event data specific to the event type.
*
* @return the data value.
*/
public Object getData() {
return this.data;
}
/**
* Set the data property: Event data specific to the event type.
*
* @param data the data value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setData(Object data) {
this.data = data;
return this;
}
/**
* Get the eventType property: The type of the event that occurred.
*
* @return the eventType value.
*/
public String getEventType() {
return this.eventType;
}
/**
* Set the eventType property: The type of the event that occurred.
*
* @param eventType the eventType value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setEventType(String eventType) {
this.eventType = eventType;
return this;
}
/**
* Get the eventTime property: The time (in UTC) the event was generated.
*
* @return the eventTime value.
*/
public OffsetDateTime getEventTime() {
return this.eventTime;
}
/**
* Set the eventTime property: The time (in UTC) the event was generated.
*
* @param eventTime the eventTime value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setEventTime(OffsetDateTime eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* Get the metadataVersion property: The schema version of the event metadata.
*
* @return the metadataVersion value.
*/
public String getMetadataVersion() {
return this.metadataVersion;
}
/**
* Get the dataVersion property: The schema version of the data object.
*
* @return the dataVersion value.
*/
public String getDataVersion() {
return this.dataVersion;
}
/**
* Set the dataVersion property: The schema version of the data object.
*
* @param dataVersion the dataVersion value to set.
* @return the EventGridEvent object itself.
*/
public EventGridEvent setDataVersion(String dataVersion) {
this.dataVersion = dataVersion;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", this.id);
jsonWriter.writeStringField("subject", this.subject);
jsonWriter.writeUntypedField("data", this.data);
jsonWriter.writeStringField("eventType", this.eventType);
jsonWriter.writeStringField("eventTime",
this.eventTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.eventTime));
jsonWriter.writeStringField("dataVersion", this.dataVersion);
jsonWriter.writeStringField("topic", this.topic);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of EventGridEvent from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of EventGridEvent if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the EventGridEvent.
*/
public static EventGridEvent fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
EventGridEvent deserializedEventGridEvent = new EventGridEvent();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedEventGridEvent.id = reader.getString();
} else if ("subject".equals(fieldName)) {
deserializedEventGridEvent.subject = reader.getString();
} else if ("data".equals(fieldName)) {
deserializedEventGridEvent.data = reader.readUntyped();
} else if ("eventType".equals(fieldName)) {
deserializedEventGridEvent.eventType = reader.getString();
} else if ("eventTime".equals(fieldName)) {
deserializedEventGridEvent.eventTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("dataVersion".equals(fieldName)) {
deserializedEventGridEvent.dataVersion = reader.getString();
} else if ("topic".equals(fieldName)) {
deserializedEventGridEvent.topic = reader.getString();
} else if ("metadataVersion".equals(fieldName)) {
deserializedEventGridEvent.metadataVersion = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedEventGridEvent;
});
}
/**
* Attempts to parse an ISO datetime string as best possible. The initial attempt will use
* {@link OffsetDateTime#from(TemporalAccessor)} and will fall back to
* {@link LocalDateTime#from(TemporalAccessor)} and apply {@link ZoneOffset#UTC} as the
* timezone.
*
* @param datetimeString The datetime string to parse.
* @return The {@link OffsetDateTime} representing the string.
* @throws DateTimeParseException If the datetime is neither an ISO offset datetime or ISO local datetime.
*/
private static OffsetDateTime parseOffsetDateTimeBest(String datetimeString) {
TemporalAccessor temporal
= DateTimeFormatter.ISO_DATE_TIME.parseBest(datetimeString, OffsetDateTime::from, LocalDateTime::from);
return (temporal.query(TemporalQueries.offset()) == null)
? LocalDateTime.from(temporal).atOffset(ZoneOffset.UTC)
: OffsetDateTime.from(temporal);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy