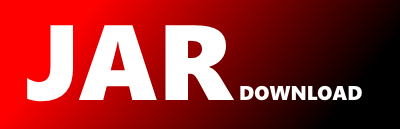
com.azure.messaging.eventgrid.package-info Maven / Gradle / Ivy
Show all versions of azure-messaging-eventgrid Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
/**
* Azure Event Grid is a highly scalable, fully
* managed event routing service. With Event Grid can connect applications and services to react to relevant events.
* This library is for publishing Event Grid events and deserializing event payloads in subscriptions.
*
* Key Concepts:
*
* - Event - Information about what happened.
* - Event Source - where the event took place.
* - Topic - the endpoint where events are published to.
* - Event Handler - the endpoint that handles the events.
* - Event Subscription - the endpoint or built-in mechanism for routing events.
*
*
* For more information see the concepts overview.
*
* Getting Started
*
* The Azure EventGrid SDK provides {@link com.azure.messaging.eventgrid.EventGridPublisherClient} and
* {@link com.azure.messaging.eventgrid.EventGridPublisherAsyncClient} for synchronous and asynchronous publishing of
* events to Azure Event Grid. These can be instantiated using the {@link com.azure.messaging.eventgrid.EventGridPublisherClientBuilder}.
*
* Authentication
*
* There are three ways to authenticate a publisher client for Azure Event Grid.
*
* Microsoft Entra ID: Using managed identity is the recommended way to authenticate. The recommended way to do so is using
* DefaultAzureCredential:
*
*
* DefaultAzureCredential credential = new DefaultAzureCredentialBuilder().build();
* EventGridPublisherClient<EventGridEvent> eventGridEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT"))
* .credential(credential)
* .buildEventGridEventPublisherClient();
*
*
*
* Topic Access Key: When a topic is created, an access key is created for that topic. It is used
* with the AzureKeyCredential:
*
*
* AzureKeyCredential credential = new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_EVENT_TOPIC_KEY"));
* EventGridPublisherClient<EventGridEvent> eventGridEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT"))
* .credential(credential)
* .buildEventGridEventPublisherClient();
*
*
*
* Shared Access Signature: A Shared Access Signature (SAS) key can be used to authenticate. First, you must create one:
*
*
* // You can get a SAS token using static methods of EventGridPublisherClient.
* String sasKey = EventGridPublisherClient.generateSas(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT"),
* new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_EVENT_TOPIC_KEY")),
* OffsetDateTime.now().plusHours(1));
*
*
*
* Once it is created, it is used with a SAS token credential:
*
*
* // Once you have this key, you can share it with anyone who needs to send events to your topic. They use it like this:
* AzureSasCredential credential = new AzureSasCredential(sasKey);
* EventGridPublisherClient<EventGridEvent> eventGridEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT"))
* .credential(credential)
* .buildEventGridEventPublisherClient();
*
*
*
*
* Send an EventGridEvent
*
* In order to interact with the Azure Event Grid service, you will need to create an instance of the {@link com.azure.messaging.eventgrid.EventGridPublisherClient} class:
*
*
* // Create a client to send events of EventGridEvent schema
* EventGridPublisherClient<EventGridEvent> eventGridEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_EVENT_ENDPOINT")) // make sure it accepts EventGridEvent
* .credential(new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_EVENT_KEY")))
* .buildEventGridEventPublisherClient();
*
*
*
*
* // Create an EventGridEvent
* User user = new User("John", "James");
* EventGridEvent eventGridEvent = new EventGridEvent("/EventGridEvents/example/source",
* "Example.EventType", BinaryData.fromObject(user), "0.1");
*
* // Send a single EventGridEvent
* eventGridEventPublisherClient.sendEvent(eventGridEvent).block();
*
* // Send a list of EventGridEvents to the EventGrid service altogether.
* // This has better performance than sending one by one.
* eventGridEventPublisherClient.sendEvents(Arrays.asList(
* eventGridEvent
* // add more EventGridEvents objects
* )).block();
*
*
*
*
* Send a Cloud Event
*
*
*
* // Create a client to send events of CloudEvent schema (com.azure.core.models.CloudEvent)
* EventGridPublisherAsyncClient<CloudEvent> cloudEventPublisherClient = new EventGridPublisherClientBuilder()
* .endpoint(System.getenv("AZURE_EVENTGRID_CLOUDEVENT_ENDPOINT")) // make sure it accepts CloudEvent
* .credential(new AzureKeyCredential(System.getenv("AZURE_EVENTGRID_CLOUDEVENT_KEY")))
* .buildCloudEventPublisherAsyncClient();
*
*
*
*
* // Create a com.azure.models.CloudEvent.
* User user = new User("Stephen", "James");
* CloudEvent cloudEventDataObject = new CloudEvent("/cloudevents/example/source", "Example.EventType",
* BinaryData.fromObject(user), CloudEventDataFormat.JSON, "application/json");
*
* // Send a single CloudEvent
* cloudEventPublisherClient.sendEvent(cloudEventDataObject).block();
*
* // Send a list of CloudEvents to the EventGrid service altogether.
* // This has better performance than sending one by one.
* cloudEventPublisherClient.sendEvents(Arrays.asList(
* cloudEventDataObject
* // add more CloudEvents objects
* )).block();
*
*
*
* @see com.azure.messaging.eventgrid.EventGridPublisherClient
* @see com.azure.messaging.eventgrid.EventGridPublisherAsyncClient
* @see com.azure.messaging.eventgrid.EventGridPublisherClientBuilder
*/
package com.azure.messaging.eventgrid;