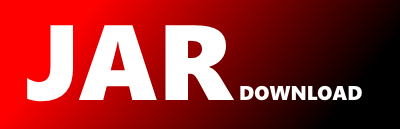
com.azure.messaging.eventgrid.systemevents.AcsChatEventInThreadBaseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of common properties of all thread-level chat events.
*/
@Fluent
public class AcsChatEventInThreadBaseProperties implements JsonSerializable {
/*
* The transaction id will be used as co-relation vector
*/
private String transactionId;
/*
* The chat thread id
*/
private String threadId;
/**
* Creates an instance of AcsChatEventInThreadBaseProperties class.
*/
public AcsChatEventInThreadBaseProperties() {
}
/**
* Get the transactionId property: The transaction id will be used as co-relation vector.
*
* @return the transactionId value.
*/
public String getTransactionId() {
return this.transactionId;
}
/**
* Set the transactionId property: The transaction id will be used as co-relation vector.
*
* @param transactionId the transactionId value to set.
* @return the AcsChatEventInThreadBaseProperties object itself.
*/
public AcsChatEventInThreadBaseProperties setTransactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Get the threadId property: The chat thread id.
*
* @return the threadId value.
*/
public String getThreadId() {
return this.threadId;
}
/**
* Set the threadId property: The chat thread id.
*
* @param threadId the threadId value to set.
* @return the AcsChatEventInThreadBaseProperties object itself.
*/
public AcsChatEventInThreadBaseProperties setThreadId(String threadId) {
this.threadId = threadId;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("transactionId", this.transactionId);
jsonWriter.writeStringField("threadId", this.threadId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AcsChatEventInThreadBaseProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AcsChatEventInThreadBaseProperties if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AcsChatEventInThreadBaseProperties.
*/
public static AcsChatEventInThreadBaseProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AcsChatEventInThreadBaseProperties deserializedAcsChatEventInThreadBaseProperties
= new AcsChatEventInThreadBaseProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("transactionId".equals(fieldName)) {
deserializedAcsChatEventInThreadBaseProperties.transactionId = reader.getString();
} else if ("threadId".equals(fieldName)) {
deserializedAcsChatEventInThreadBaseProperties.threadId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAcsChatEventInThreadBaseProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy