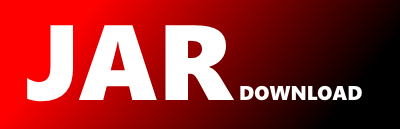
com.azure.messaging.eventgrid.systemevents.AcsChatThreadCreatedWithUserEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.Map;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.Communication.ChatThreadCreatedWithUser event.
*/
@Fluent
public final class AcsChatThreadCreatedWithUserEventData extends AcsChatThreadEventBaseProperties {
/*
* The communication identifier of the user who created the thread
*/
private CommunicationIdentifierModel createdByCommunicationIdentifier;
/*
* The thread properties
*/
private Map properties;
/*
* The thread metadata
*/
private Map metadata;
/*
* The list of properties of participants who are part of the thread
*/
private List participants;
/**
* Creates an instance of AcsChatThreadCreatedWithUserEventData class.
*/
public AcsChatThreadCreatedWithUserEventData() {
}
/**
* Get the createdByCommunicationIdentifier property: The communication identifier of the user who created the
* thread.
*
* @return the createdByCommunicationIdentifier value.
*/
public CommunicationIdentifierModel getCreatedByCommunicationIdentifier() {
return this.createdByCommunicationIdentifier;
}
/**
* Set the createdByCommunicationIdentifier property: The communication identifier of the user who created the
* thread.
*
* @param createdByCommunicationIdentifier the createdByCommunicationIdentifier value to set.
* @return the AcsChatThreadCreatedWithUserEventData object itself.
*/
public AcsChatThreadCreatedWithUserEventData
setCreatedByCommunicationIdentifier(CommunicationIdentifierModel createdByCommunicationIdentifier) {
this.createdByCommunicationIdentifier = createdByCommunicationIdentifier;
return this;
}
/**
* Get the properties property: The thread properties.
*
* @return the properties value.
*/
public Map getProperties() {
return this.properties;
}
/**
* Set the properties property: The thread properties.
*
* @param properties the properties value to set.
* @return the AcsChatThreadCreatedWithUserEventData object itself.
*/
public AcsChatThreadCreatedWithUserEventData setProperties(Map properties) {
this.properties = properties;
return this;
}
/**
* Get the metadata property: The thread metadata.
*
* @return the metadata value.
*/
public Map getMetadata() {
return this.metadata;
}
/**
* Set the metadata property: The thread metadata.
*
* @param metadata the metadata value to set.
* @return the AcsChatThreadCreatedWithUserEventData object itself.
*/
public AcsChatThreadCreatedWithUserEventData setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Get the participants property: The list of properties of participants who are part of the thread.
*
* @return the participants value.
*/
public List getParticipants() {
return this.participants;
}
/**
* Set the participants property: The list of properties of participants who are part of the thread.
*
* @param participants the participants value to set.
* @return the AcsChatThreadCreatedWithUserEventData object itself.
*/
public AcsChatThreadCreatedWithUserEventData
setParticipants(List participants) {
this.participants = participants;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AcsChatThreadCreatedWithUserEventData setCreateTime(OffsetDateTime createTime) {
super.setCreateTime(createTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AcsChatThreadCreatedWithUserEventData setVersion(Long version) {
super.setVersion(version);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AcsChatThreadCreatedWithUserEventData
setRecipientCommunicationIdentifier(CommunicationIdentifierModel recipientCommunicationIdentifier) {
super.setRecipientCommunicationIdentifier(recipientCommunicationIdentifier);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AcsChatThreadCreatedWithUserEventData setTransactionId(String transactionId) {
super.setTransactionId(transactionId);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AcsChatThreadCreatedWithUserEventData setThreadId(String threadId) {
super.setThreadId(threadId);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("recipientCommunicationIdentifier", getRecipientCommunicationIdentifier());
jsonWriter.writeStringField("transactionId", getTransactionId());
jsonWriter.writeStringField("threadId", getThreadId());
jsonWriter.writeStringField("createTime",
getCreateTime() == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(getCreateTime()));
jsonWriter.writeNumberField("version", getVersion());
jsonWriter.writeJsonField("createdByCommunicationIdentifier", this.createdByCommunicationIdentifier);
jsonWriter.writeMapField("properties", this.properties, (writer, element) -> writer.writeUntyped(element));
jsonWriter.writeMapField("metadata", this.metadata, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("participants", this.participants, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AcsChatThreadCreatedWithUserEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AcsChatThreadCreatedWithUserEventData if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AcsChatThreadCreatedWithUserEventData.
*/
public static AcsChatThreadCreatedWithUserEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AcsChatThreadCreatedWithUserEventData deserializedAcsChatThreadCreatedWithUserEventData
= new AcsChatThreadCreatedWithUserEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("recipientCommunicationIdentifier".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData
.setRecipientCommunicationIdentifier(CommunicationIdentifierModel.fromJson(reader));
} else if ("transactionId".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData.setTransactionId(reader.getString());
} else if ("threadId".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData.setThreadId(reader.getString());
} else if ("createTime".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData.setCreateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("version".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData
.setVersion(reader.getNullable(JsonReader::getLong));
} else if ("createdByCommunicationIdentifier".equals(fieldName)) {
deserializedAcsChatThreadCreatedWithUserEventData.createdByCommunicationIdentifier
= CommunicationIdentifierModel.fromJson(reader);
} else if ("properties".equals(fieldName)) {
Map properties = reader.readMap(reader1 -> reader1.readUntyped());
deserializedAcsChatThreadCreatedWithUserEventData.properties = properties;
} else if ("metadata".equals(fieldName)) {
Map metadata = reader.readMap(reader1 -> reader1.getString());
deserializedAcsChatThreadCreatedWithUserEventData.metadata = metadata;
} else if ("participants".equals(fieldName)) {
List participants
= reader.readArray(reader1 -> AcsChatThreadParticipantProperties.fromJson(reader1));
deserializedAcsChatThreadCreatedWithUserEventData.participants = participants;
} else {
reader.skipChildren();
}
}
return deserializedAcsChatThreadCreatedWithUserEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy