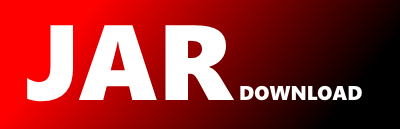
com.azure.messaging.eventgrid.systemevents.AcsEmailDeliveryReportReceivedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.Communication.EmailDeliveryReportReceived event.
*/
@Fluent
public final class AcsEmailDeliveryReportReceivedEventData
implements JsonSerializable {
/*
* The Sender Email Address
*/
private String sender;
/*
* The recipient Email Address
*/
private String recipient;
/*
* The Id of the email been sent
*/
private String messageId;
/*
* The status of the email. Any value other than Delivered is considered failed.
*/
private AcsEmailDeliveryReportStatus status;
/*
* Detailed information about the status if any
*/
private AcsEmailDeliveryReportStatusDetails deliveryStatusDetails;
/*
* The time at which the email delivery report received timestamp
*/
private OffsetDateTime deliveryAttemptTimestamp;
/**
* Creates an instance of AcsEmailDeliveryReportReceivedEventData class.
*/
public AcsEmailDeliveryReportReceivedEventData() {
}
/**
* Get the sender property: The Sender Email Address.
*
* @return the sender value.
*/
public String getSender() {
return this.sender;
}
/**
* Set the sender property: The Sender Email Address.
*
* @param sender the sender value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData setSender(String sender) {
this.sender = sender;
return this;
}
/**
* Get the recipient property: The recipient Email Address.
*
* @return the recipient value.
*/
public String getRecipient() {
return this.recipient;
}
/**
* Set the recipient property: The recipient Email Address.
*
* @param recipient the recipient value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData setRecipient(String recipient) {
this.recipient = recipient;
return this;
}
/**
* Get the messageId property: The Id of the email been sent.
*
* @return the messageId value.
*/
public String getMessageId() {
return this.messageId;
}
/**
* Set the messageId property: The Id of the email been sent.
*
* @param messageId the messageId value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData setMessageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Get the status property: The status of the email. Any value other than Delivered is considered failed.
*
* @return the status value.
*/
public AcsEmailDeliveryReportStatus getStatus() {
return this.status;
}
/**
* Set the status property: The status of the email. Any value other than Delivered is considered failed.
*
* @param status the status value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData setStatus(AcsEmailDeliveryReportStatus status) {
this.status = status;
return this;
}
/**
* Get the deliveryStatusDetails property: Detailed information about the status if any.
*
* @return the deliveryStatusDetails value.
*/
public AcsEmailDeliveryReportStatusDetails getDeliveryStatusDetails() {
return this.deliveryStatusDetails;
}
/**
* Set the deliveryStatusDetails property: Detailed information about the status if any.
*
* @param deliveryStatusDetails the deliveryStatusDetails value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData
setDeliveryStatusDetails(AcsEmailDeliveryReportStatusDetails deliveryStatusDetails) {
this.deliveryStatusDetails = deliveryStatusDetails;
return this;
}
/**
* Get the deliveryAttemptTimestamp property: The time at which the email delivery report received timestamp.
*
* @return the deliveryAttemptTimestamp value.
*/
public OffsetDateTime getDeliveryAttemptTimestamp() {
return this.deliveryAttemptTimestamp;
}
/**
* Set the deliveryAttemptTimestamp property: The time at which the email delivery report received timestamp.
*
* @param deliveryAttemptTimestamp the deliveryAttemptTimestamp value to set.
* @return the AcsEmailDeliveryReportReceivedEventData object itself.
*/
public AcsEmailDeliveryReportReceivedEventData
setDeliveryAttemptTimestamp(OffsetDateTime deliveryAttemptTimestamp) {
this.deliveryAttemptTimestamp = deliveryAttemptTimestamp;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("sender", this.sender);
jsonWriter.writeStringField("recipient", this.recipient);
jsonWriter.writeStringField("messageId", this.messageId);
jsonWriter.writeStringField("status", this.status == null ? null : this.status.toString());
jsonWriter.writeJsonField("deliveryStatusDetails", this.deliveryStatusDetails);
jsonWriter.writeStringField("deliveryAttemptTimestamp",
this.deliveryAttemptTimestamp == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.deliveryAttemptTimestamp));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AcsEmailDeliveryReportReceivedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AcsEmailDeliveryReportReceivedEventData if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AcsEmailDeliveryReportReceivedEventData.
*/
public static AcsEmailDeliveryReportReceivedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AcsEmailDeliveryReportReceivedEventData deserializedAcsEmailDeliveryReportReceivedEventData
= new AcsEmailDeliveryReportReceivedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("sender".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.sender = reader.getString();
} else if ("recipient".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.recipient = reader.getString();
} else if ("messageId".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.messageId = reader.getString();
} else if ("status".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.status
= AcsEmailDeliveryReportStatus.fromString(reader.getString());
} else if ("deliveryStatusDetails".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.deliveryStatusDetails
= AcsEmailDeliveryReportStatusDetails.fromJson(reader);
} else if ("deliveryAttemptTimestamp".equals(fieldName)) {
deserializedAcsEmailDeliveryReportReceivedEventData.deliveryAttemptTimestamp = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedAcsEmailDeliveryReportReceivedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy