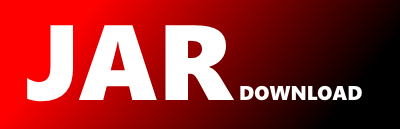
com.azure.messaging.eventgrid.systemevents.DeviceConnectionStateEventProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of the Data property of an EventGridEvent for a device connection state event (DeviceConnected,
* DeviceDisconnected).
*/
@Fluent
public class DeviceConnectionStateEventProperties implements JsonSerializable {
/*
* The unique identifier of the device. This case-sensitive string can be up to 128 characters long, and supports
* ASCII 7-bit alphanumeric characters plus the following special characters: - : . + % _ # * ? ! ( ) , = @ ; $
* '.
*/
private String deviceId;
/*
* The unique identifier of the module. This case-sensitive string can be up to 128 characters long, and supports
* ASCII 7-bit alphanumeric characters plus the following special characters: - : . + % _ # * ? ! ( ) , = @ ; $
* '.
*/
private String moduleId;
/*
* Name of the IoT Hub where the device was created or deleted.
*/
private String hubName;
/*
* Information about the device connection state event.
*/
private DeviceConnectionStateEventInfo deviceConnectionStateEventInfo;
/**
* Creates an instance of DeviceConnectionStateEventProperties class.
*/
public DeviceConnectionStateEventProperties() {
}
/**
* Get the deviceId property: The unique identifier of the device. This case-sensitive string can be up to 128
* characters long, and supports ASCII 7-bit alphanumeric characters plus the following special characters: - : . +
* % _ # * ? ! ( ) , = @ ; $ '.
*
* @return the deviceId value.
*/
public String getDeviceId() {
return this.deviceId;
}
/**
* Set the deviceId property: The unique identifier of the device. This case-sensitive string can be up to 128
* characters long, and supports ASCII 7-bit alphanumeric characters plus the following special characters: - : . +
* % _ # * ? ! ( ) , = @ ; $ '.
*
* @param deviceId the deviceId value to set.
* @return the DeviceConnectionStateEventProperties object itself.
*/
public DeviceConnectionStateEventProperties setDeviceId(String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Get the moduleId property: The unique identifier of the module. This case-sensitive string can be up to 128
* characters long, and supports ASCII 7-bit alphanumeric characters plus the following special characters: - : . +
* % _ # * ? ! ( ) , = @ ; $ '.
*
* @return the moduleId value.
*/
public String getModuleId() {
return this.moduleId;
}
/**
* Set the moduleId property: The unique identifier of the module. This case-sensitive string can be up to 128
* characters long, and supports ASCII 7-bit alphanumeric characters plus the following special characters: - : . +
* % _ # * ? ! ( ) , = @ ; $ '.
*
* @param moduleId the moduleId value to set.
* @return the DeviceConnectionStateEventProperties object itself.
*/
public DeviceConnectionStateEventProperties setModuleId(String moduleId) {
this.moduleId = moduleId;
return this;
}
/**
* Get the hubName property: Name of the IoT Hub where the device was created or deleted.
*
* @return the hubName value.
*/
public String getHubName() {
return this.hubName;
}
/**
* Set the hubName property: Name of the IoT Hub where the device was created or deleted.
*
* @param hubName the hubName value to set.
* @return the DeviceConnectionStateEventProperties object itself.
*/
public DeviceConnectionStateEventProperties setHubName(String hubName) {
this.hubName = hubName;
return this;
}
/**
* Get the deviceConnectionStateEventInfo property: Information about the device connection state event.
*
* @return the deviceConnectionStateEventInfo value.
*/
public DeviceConnectionStateEventInfo getDeviceConnectionStateEventInfo() {
return this.deviceConnectionStateEventInfo;
}
/**
* Set the deviceConnectionStateEventInfo property: Information about the device connection state event.
*
* @param deviceConnectionStateEventInfo the deviceConnectionStateEventInfo value to set.
* @return the DeviceConnectionStateEventProperties object itself.
*/
public DeviceConnectionStateEventProperties
setDeviceConnectionStateEventInfo(DeviceConnectionStateEventInfo deviceConnectionStateEventInfo) {
this.deviceConnectionStateEventInfo = deviceConnectionStateEventInfo;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("deviceId", this.deviceId);
jsonWriter.writeStringField("moduleId", this.moduleId);
jsonWriter.writeStringField("hubName", this.hubName);
jsonWriter.writeJsonField("deviceConnectionStateEventInfo", this.deviceConnectionStateEventInfo);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DeviceConnectionStateEventProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DeviceConnectionStateEventProperties if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the DeviceConnectionStateEventProperties.
*/
public static DeviceConnectionStateEventProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DeviceConnectionStateEventProperties deserializedDeviceConnectionStateEventProperties
= new DeviceConnectionStateEventProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("deviceId".equals(fieldName)) {
deserializedDeviceConnectionStateEventProperties.deviceId = reader.getString();
} else if ("moduleId".equals(fieldName)) {
deserializedDeviceConnectionStateEventProperties.moduleId = reader.getString();
} else if ("hubName".equals(fieldName)) {
deserializedDeviceConnectionStateEventProperties.hubName = reader.getString();
} else if ("deviceConnectionStateEventInfo".equals(fieldName)) {
deserializedDeviceConnectionStateEventProperties.deviceConnectionStateEventInfo
= DeviceConnectionStateEventInfo.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedDeviceConnectionStateEventProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy