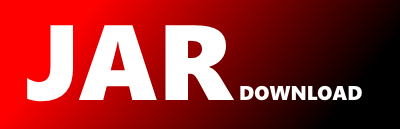
com.azure.messaging.eventgrid.systemevents.HealthcareDicomImageDeletedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.HealthcareApis.DicomImageDeleted event.
*/
@Fluent
public final class HealthcareDicomImageDeletedEventData
implements JsonSerializable {
/*
* Data partition name
*/
private String partitionName;
/*
* Unique identifier for the Study
*/
private String imageStudyInstanceUid;
/*
* Unique identifier for the Series
*/
private String imageSeriesInstanceUid;
/*
* Unique identifier for the DICOM Image
*/
private String imageSopInstanceUid;
/*
* Host name of the DICOM account for this image.
*/
private String serviceHostName;
/*
* Sequence number of the DICOM Service within Azure Health Data Services. It is unique for every image creation and
* deletion within the service.
*/
private Long sequenceNumber;
/**
* Creates an instance of HealthcareDicomImageDeletedEventData class.
*/
public HealthcareDicomImageDeletedEventData() {
}
/**
* Get the partitionName property: Data partition name.
*
* @return the partitionName value.
*/
public String getPartitionName() {
return this.partitionName;
}
/**
* Set the partitionName property: Data partition name.
*
* @param partitionName the partitionName value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setPartitionName(String partitionName) {
this.partitionName = partitionName;
return this;
}
/**
* Get the imageStudyInstanceUid property: Unique identifier for the Study.
*
* @return the imageStudyInstanceUid value.
*/
public String getImageStudyInstanceUid() {
return this.imageStudyInstanceUid;
}
/**
* Set the imageStudyInstanceUid property: Unique identifier for the Study.
*
* @param imageStudyInstanceUid the imageStudyInstanceUid value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setImageStudyInstanceUid(String imageStudyInstanceUid) {
this.imageStudyInstanceUid = imageStudyInstanceUid;
return this;
}
/**
* Get the imageSeriesInstanceUid property: Unique identifier for the Series.
*
* @return the imageSeriesInstanceUid value.
*/
public String getImageSeriesInstanceUid() {
return this.imageSeriesInstanceUid;
}
/**
* Set the imageSeriesInstanceUid property: Unique identifier for the Series.
*
* @param imageSeriesInstanceUid the imageSeriesInstanceUid value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setImageSeriesInstanceUid(String imageSeriesInstanceUid) {
this.imageSeriesInstanceUid = imageSeriesInstanceUid;
return this;
}
/**
* Get the imageSopInstanceUid property: Unique identifier for the DICOM Image.
*
* @return the imageSopInstanceUid value.
*/
public String getImageSopInstanceUid() {
return this.imageSopInstanceUid;
}
/**
* Set the imageSopInstanceUid property: Unique identifier for the DICOM Image.
*
* @param imageSopInstanceUid the imageSopInstanceUid value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setImageSopInstanceUid(String imageSopInstanceUid) {
this.imageSopInstanceUid = imageSopInstanceUid;
return this;
}
/**
* Get the serviceHostName property: Host name of the DICOM account for this image.
*
* @return the serviceHostName value.
*/
public String getServiceHostName() {
return this.serviceHostName;
}
/**
* Set the serviceHostName property: Host name of the DICOM account for this image.
*
* @param serviceHostName the serviceHostName value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setServiceHostName(String serviceHostName) {
this.serviceHostName = serviceHostName;
return this;
}
/**
* Get the sequenceNumber property: Sequence number of the DICOM Service within Azure Health Data Services. It is
* unique for every image creation and deletion within the service.
*
* @return the sequenceNumber value.
*/
public Long getSequenceNumber() {
return this.sequenceNumber;
}
/**
* Set the sequenceNumber property: Sequence number of the DICOM Service within Azure Health Data Services. It is
* unique for every image creation and deletion within the service.
*
* @param sequenceNumber the sequenceNumber value to set.
* @return the HealthcareDicomImageDeletedEventData object itself.
*/
public HealthcareDicomImageDeletedEventData setSequenceNumber(Long sequenceNumber) {
this.sequenceNumber = sequenceNumber;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("partitionName", this.partitionName);
jsonWriter.writeStringField("imageStudyInstanceUid", this.imageStudyInstanceUid);
jsonWriter.writeStringField("imageSeriesInstanceUid", this.imageSeriesInstanceUid);
jsonWriter.writeStringField("imageSopInstanceUid", this.imageSopInstanceUid);
jsonWriter.writeStringField("serviceHostName", this.serviceHostName);
jsonWriter.writeNumberField("sequenceNumber", this.sequenceNumber);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HealthcareDicomImageDeletedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HealthcareDicomImageDeletedEventData if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the HealthcareDicomImageDeletedEventData.
*/
public static HealthcareDicomImageDeletedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HealthcareDicomImageDeletedEventData deserializedHealthcareDicomImageDeletedEventData
= new HealthcareDicomImageDeletedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("partitionName".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.partitionName = reader.getString();
} else if ("imageStudyInstanceUid".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.imageStudyInstanceUid = reader.getString();
} else if ("imageSeriesInstanceUid".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.imageSeriesInstanceUid = reader.getString();
} else if ("imageSopInstanceUid".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.imageSopInstanceUid = reader.getString();
} else if ("serviceHostName".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.serviceHostName = reader.getString();
} else if ("sequenceNumber".equals(fieldName)) {
deserializedHealthcareDicomImageDeletedEventData.sequenceNumber
= reader.getNullable(JsonReader::getLong);
} else {
reader.skipChildren();
}
}
return deserializedHealthcareDicomImageDeletedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy