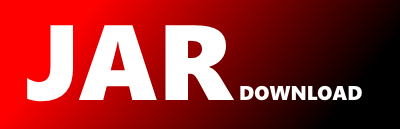
com.azure.messaging.eventgrid.systemevents.HealthcareFhirResourceUpdatedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.HealthcareApis.FhirResourceUpdated event.
*/
@Fluent
public final class HealthcareFhirResourceUpdatedEventData
implements JsonSerializable {
/*
* Type of HL7 FHIR resource.
*/
private HealthcareFhirResourceType fhirResourceType;
/*
* Domain name of FHIR account for this resource.
*/
private String fhirServiceHostName;
/*
* Id of HL7 FHIR resource.
*/
private String fhirResourceId;
/*
* VersionId of HL7 FHIR resource. It changes when the resource is created, updated, or deleted(soft-deletion).
*/
private Long fhirResourceVersionId;
/**
* Creates an instance of HealthcareFhirResourceUpdatedEventData class.
*/
public HealthcareFhirResourceUpdatedEventData() {
}
/**
* Get the fhirResourceType property: Type of HL7 FHIR resource.
*
* @return the fhirResourceType value.
*/
public HealthcareFhirResourceType getFhirResourceType() {
return this.fhirResourceType;
}
/**
* Set the fhirResourceType property: Type of HL7 FHIR resource.
*
* @param fhirResourceType the fhirResourceType value to set.
* @return the HealthcareFhirResourceUpdatedEventData object itself.
*/
public HealthcareFhirResourceUpdatedEventData setFhirResourceType(HealthcareFhirResourceType fhirResourceType) {
this.fhirResourceType = fhirResourceType;
return this;
}
/**
* Get the fhirServiceHostName property: Domain name of FHIR account for this resource.
*
* @return the fhirServiceHostName value.
*/
public String getFhirServiceHostName() {
return this.fhirServiceHostName;
}
/**
* Set the fhirServiceHostName property: Domain name of FHIR account for this resource.
*
* @param fhirServiceHostName the fhirServiceHostName value to set.
* @return the HealthcareFhirResourceUpdatedEventData object itself.
*/
public HealthcareFhirResourceUpdatedEventData setFhirServiceHostName(String fhirServiceHostName) {
this.fhirServiceHostName = fhirServiceHostName;
return this;
}
/**
* Get the fhirResourceId property: Id of HL7 FHIR resource.
*
* @return the fhirResourceId value.
*/
public String getFhirResourceId() {
return this.fhirResourceId;
}
/**
* Set the fhirResourceId property: Id of HL7 FHIR resource.
*
* @param fhirResourceId the fhirResourceId value to set.
* @return the HealthcareFhirResourceUpdatedEventData object itself.
*/
public HealthcareFhirResourceUpdatedEventData setFhirResourceId(String fhirResourceId) {
this.fhirResourceId = fhirResourceId;
return this;
}
/**
* Get the fhirResourceVersionId property: VersionId of HL7 FHIR resource. It changes when the resource is created,
* updated, or deleted(soft-deletion).
*
* @return the fhirResourceVersionId value.
*/
public Long getFhirResourceVersionId() {
return this.fhirResourceVersionId;
}
/**
* Set the fhirResourceVersionId property: VersionId of HL7 FHIR resource. It changes when the resource is created,
* updated, or deleted(soft-deletion).
*
* @param fhirResourceVersionId the fhirResourceVersionId value to set.
* @return the HealthcareFhirResourceUpdatedEventData object itself.
*/
public HealthcareFhirResourceUpdatedEventData setFhirResourceVersionId(Long fhirResourceVersionId) {
this.fhirResourceVersionId = fhirResourceVersionId;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("resourceType",
this.fhirResourceType == null ? null : this.fhirResourceType.toString());
jsonWriter.writeStringField("resourceFhirAccount", this.fhirServiceHostName);
jsonWriter.writeStringField("resourceFhirId", this.fhirResourceId);
jsonWriter.writeNumberField("resourceVersionId", this.fhirResourceVersionId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HealthcareFhirResourceUpdatedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HealthcareFhirResourceUpdatedEventData if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the HealthcareFhirResourceUpdatedEventData.
*/
public static HealthcareFhirResourceUpdatedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HealthcareFhirResourceUpdatedEventData deserializedHealthcareFhirResourceUpdatedEventData
= new HealthcareFhirResourceUpdatedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("resourceType".equals(fieldName)) {
deserializedHealthcareFhirResourceUpdatedEventData.fhirResourceType
= HealthcareFhirResourceType.fromString(reader.getString());
} else if ("resourceFhirAccount".equals(fieldName)) {
deserializedHealthcareFhirResourceUpdatedEventData.fhirServiceHostName = reader.getString();
} else if ("resourceFhirId".equals(fieldName)) {
deserializedHealthcareFhirResourceUpdatedEventData.fhirResourceId = reader.getString();
} else if ("resourceVersionId".equals(fieldName)) {
deserializedHealthcareFhirResourceUpdatedEventData.fhirResourceVersionId
= reader.getNullable(JsonReader::getLong);
} else {
reader.skipChildren();
}
}
return deserializedHealthcareFhirResourceUpdatedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy