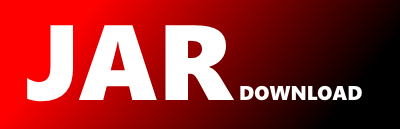
com.azure.messaging.eventgrid.systemevents.MachineLearningServicesDatasetDriftDetectedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.MachineLearningServices.DatasetDriftDetected event.
*/
@Fluent
public final class MachineLearningServicesDatasetDriftDetectedEventData
implements JsonSerializable {
/*
* The ID of the data drift monitor that triggered the event.
*/
private String dataDriftId;
/*
* The name of the data drift monitor that triggered the event.
*/
private String dataDriftName;
/*
* The ID of the Run that detected data drift.
*/
private String runId;
/*
* The ID of the base Dataset used to detect drift.
*/
private String baseDatasetId;
/*
* The ID of the target Dataset used to detect drift.
*/
private String targetDatasetId;
/*
* The coefficient result that triggered the event.
*/
private Double driftCoefficient;
/*
* The start time of the target dataset time series that resulted in drift detection.
*/
private OffsetDateTime startTime;
/*
* The end time of the target dataset time series that resulted in drift detection.
*/
private OffsetDateTime endTime;
/**
* Creates an instance of MachineLearningServicesDatasetDriftDetectedEventData class.
*/
public MachineLearningServicesDatasetDriftDetectedEventData() {
}
/**
* Get the dataDriftId property: The ID of the data drift monitor that triggered the event.
*
* @return the dataDriftId value.
*/
public String getDataDriftId() {
return this.dataDriftId;
}
/**
* Set the dataDriftId property: The ID of the data drift monitor that triggered the event.
*
* @param dataDriftId the dataDriftId value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setDataDriftId(String dataDriftId) {
this.dataDriftId = dataDriftId;
return this;
}
/**
* Get the dataDriftName property: The name of the data drift monitor that triggered the event.
*
* @return the dataDriftName value.
*/
public String getDataDriftName() {
return this.dataDriftName;
}
/**
* Set the dataDriftName property: The name of the data drift monitor that triggered the event.
*
* @param dataDriftName the dataDriftName value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setDataDriftName(String dataDriftName) {
this.dataDriftName = dataDriftName;
return this;
}
/**
* Get the runId property: The ID of the Run that detected data drift.
*
* @return the runId value.
*/
public String getRunId() {
return this.runId;
}
/**
* Set the runId property: The ID of the Run that detected data drift.
*
* @param runId the runId value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setRunId(String runId) {
this.runId = runId;
return this;
}
/**
* Get the baseDatasetId property: The ID of the base Dataset used to detect drift.
*
* @return the baseDatasetId value.
*/
public String getBaseDatasetId() {
return this.baseDatasetId;
}
/**
* Set the baseDatasetId property: The ID of the base Dataset used to detect drift.
*
* @param baseDatasetId the baseDatasetId value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setBaseDatasetId(String baseDatasetId) {
this.baseDatasetId = baseDatasetId;
return this;
}
/**
* Get the targetDatasetId property: The ID of the target Dataset used to detect drift.
*
* @return the targetDatasetId value.
*/
public String getTargetDatasetId() {
return this.targetDatasetId;
}
/**
* Set the targetDatasetId property: The ID of the target Dataset used to detect drift.
*
* @param targetDatasetId the targetDatasetId value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setTargetDatasetId(String targetDatasetId) {
this.targetDatasetId = targetDatasetId;
return this;
}
/**
* Get the driftCoefficient property: The coefficient result that triggered the event.
*
* @return the driftCoefficient value.
*/
public Double getDriftCoefficient() {
return this.driftCoefficient;
}
/**
* Set the driftCoefficient property: The coefficient result that triggered the event.
*
* @param driftCoefficient the driftCoefficient value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setDriftCoefficient(Double driftCoefficient) {
this.driftCoefficient = driftCoefficient;
return this;
}
/**
* Get the startTime property: The start time of the target dataset time series that resulted in drift detection.
*
* @return the startTime value.
*/
public OffsetDateTime getStartTime() {
return this.startTime;
}
/**
* Set the startTime property: The start time of the target dataset time series that resulted in drift detection.
*
* @param startTime the startTime value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setStartTime(OffsetDateTime startTime) {
this.startTime = startTime;
return this;
}
/**
* Get the endTime property: The end time of the target dataset time series that resulted in drift detection.
*
* @return the endTime value.
*/
public OffsetDateTime getEndTime() {
return this.endTime;
}
/**
* Set the endTime property: The end time of the target dataset time series that resulted in drift detection.
*
* @param endTime the endTime value to set.
* @return the MachineLearningServicesDatasetDriftDetectedEventData object itself.
*/
public MachineLearningServicesDatasetDriftDetectedEventData setEndTime(OffsetDateTime endTime) {
this.endTime = endTime;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("dataDriftId", this.dataDriftId);
jsonWriter.writeStringField("dataDriftName", this.dataDriftName);
jsonWriter.writeStringField("runId", this.runId);
jsonWriter.writeStringField("baseDatasetId", this.baseDatasetId);
jsonWriter.writeStringField("targetDatasetId", this.targetDatasetId);
jsonWriter.writeNumberField("driftCoefficient", this.driftCoefficient);
jsonWriter.writeStringField("startTime",
this.startTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.startTime));
jsonWriter.writeStringField("endTime",
this.endTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.endTime));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MachineLearningServicesDatasetDriftDetectedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MachineLearningServicesDatasetDriftDetectedEventData if the JsonReader was pointing to an
* instance of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MachineLearningServicesDatasetDriftDetectedEventData.
*/
public static MachineLearningServicesDatasetDriftDetectedEventData fromJson(JsonReader jsonReader)
throws IOException {
return jsonReader.readObject(reader -> {
MachineLearningServicesDatasetDriftDetectedEventData deserializedMachineLearningServicesDatasetDriftDetectedEventData
= new MachineLearningServicesDatasetDriftDetectedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("dataDriftId".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.dataDriftId = reader.getString();
} else if ("dataDriftName".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.dataDriftName = reader.getString();
} else if ("runId".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.runId = reader.getString();
} else if ("baseDatasetId".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.baseDatasetId = reader.getString();
} else if ("targetDatasetId".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.targetDatasetId
= reader.getString();
} else if ("driftCoefficient".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.driftCoefficient
= reader.getNullable(JsonReader::getDouble);
} else if ("startTime".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.startTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("endTime".equals(fieldName)) {
deserializedMachineLearningServicesDatasetDriftDetectedEventData.endTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedMachineLearningServicesDatasetDriftDetectedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy