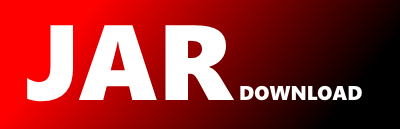
com.azure.messaging.eventgrid.systemevents.MapsGeofenceGeometry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The geofence geometry.
*/
@Fluent
public final class MapsGeofenceGeometry implements JsonSerializable {
/*
* ID of the device.
*/
private String deviceId;
/*
* Distance from the coordinate to the closest border of the geofence. Positive means the coordinate is outside of
* the geofence. If the coordinate is outside of the geofence, but more than the value of searchBuffer away from the
* closest geofence border, then the value is 999. Negative means the coordinate is inside of the geofence. If the
* coordinate is inside the polygon, but more than the value of searchBuffer away from the closest geofencing
* border,then the value is -999. A value of 999 means that there is great confidence the coordinate is well outside
* the geofence. A value of -999 means that there is great confidence the coordinate is well within the geofence.
*/
private Float distance;
/*
* The unique ID for the geofence geometry.
*/
private String geometryId;
/*
* Latitude of the nearest point of the geometry.
*/
private Float nearestLat;
/*
* Longitude of the nearest point of the geometry.
*/
private Float nearestLon;
/*
* The unique id returned from user upload service when uploading a geofence. Will not be included in geofencing
* post API.
*/
private String udId;
/**
* Creates an instance of MapsGeofenceGeometry class.
*/
public MapsGeofenceGeometry() {
}
/**
* Get the deviceId property: ID of the device.
*
* @return the deviceId value.
*/
public String getDeviceId() {
return this.deviceId;
}
/**
* Set the deviceId property: ID of the device.
*
* @param deviceId the deviceId value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setDeviceId(String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Get the distance property: Distance from the coordinate to the closest border of the geofence. Positive means the
* coordinate is outside of the geofence. If the coordinate is outside of the geofence, but more than the value of
* searchBuffer away from the closest geofence border, then the value is 999. Negative means the coordinate is
* inside of the geofence. If the coordinate is inside the polygon, but more than the value of searchBuffer away
* from the closest geofencing border,then the value is -999. A value of 999 means that there is great confidence
* the coordinate is well outside the geofence. A value of -999 means that there is great confidence the coordinate
* is well within the geofence.
*
* @return the distance value.
*/
public Float getDistance() {
return this.distance;
}
/**
* Set the distance property: Distance from the coordinate to the closest border of the geofence. Positive means the
* coordinate is outside of the geofence. If the coordinate is outside of the geofence, but more than the value of
* searchBuffer away from the closest geofence border, then the value is 999. Negative means the coordinate is
* inside of the geofence. If the coordinate is inside the polygon, but more than the value of searchBuffer away
* from the closest geofencing border,then the value is -999. A value of 999 means that there is great confidence
* the coordinate is well outside the geofence. A value of -999 means that there is great confidence the coordinate
* is well within the geofence.
*
* @param distance the distance value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setDistance(Float distance) {
this.distance = distance;
return this;
}
/**
* Get the geometryId property: The unique ID for the geofence geometry.
*
* @return the geometryId value.
*/
public String getGeometryId() {
return this.geometryId;
}
/**
* Set the geometryId property: The unique ID for the geofence geometry.
*
* @param geometryId the geometryId value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setGeometryId(String geometryId) {
this.geometryId = geometryId;
return this;
}
/**
* Get the nearestLat property: Latitude of the nearest point of the geometry.
*
* @return the nearestLat value.
*/
public Float getNearestLat() {
return this.nearestLat;
}
/**
* Set the nearestLat property: Latitude of the nearest point of the geometry.
*
* @param nearestLat the nearestLat value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setNearestLat(Float nearestLat) {
this.nearestLat = nearestLat;
return this;
}
/**
* Get the nearestLon property: Longitude of the nearest point of the geometry.
*
* @return the nearestLon value.
*/
public Float getNearestLon() {
return this.nearestLon;
}
/**
* Set the nearestLon property: Longitude of the nearest point of the geometry.
*
* @param nearestLon the nearestLon value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setNearestLon(Float nearestLon) {
this.nearestLon = nearestLon;
return this;
}
/**
* Get the udId property: The unique id returned from user upload service when uploading a geofence. Will not be
* included in geofencing post API.
*
* @return the udId value.
*/
public String getUdId() {
return this.udId;
}
/**
* Set the udId property: The unique id returned from user upload service when uploading a geofence. Will not be
* included in geofencing post API.
*
* @param udId the udId value to set.
* @return the MapsGeofenceGeometry object itself.
*/
public MapsGeofenceGeometry setUdId(String udId) {
this.udId = udId;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("deviceId", this.deviceId);
jsonWriter.writeNumberField("distance", this.distance);
jsonWriter.writeStringField("geometryId", this.geometryId);
jsonWriter.writeNumberField("nearestLat", this.nearestLat);
jsonWriter.writeNumberField("nearestLon", this.nearestLon);
jsonWriter.writeStringField("udId", this.udId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MapsGeofenceGeometry from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MapsGeofenceGeometry if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the MapsGeofenceGeometry.
*/
public static MapsGeofenceGeometry fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MapsGeofenceGeometry deserializedMapsGeofenceGeometry = new MapsGeofenceGeometry();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("deviceId".equals(fieldName)) {
deserializedMapsGeofenceGeometry.deviceId = reader.getString();
} else if ("distance".equals(fieldName)) {
deserializedMapsGeofenceGeometry.distance = reader.getNullable(JsonReader::getFloat);
} else if ("geometryId".equals(fieldName)) {
deserializedMapsGeofenceGeometry.geometryId = reader.getString();
} else if ("nearestLat".equals(fieldName)) {
deserializedMapsGeofenceGeometry.nearestLat = reader.getNullable(JsonReader::getFloat);
} else if ("nearestLon".equals(fieldName)) {
deserializedMapsGeofenceGeometry.nearestLon = reader.getNullable(JsonReader::getFloat);
} else if ("udId".equals(fieldName)) {
deserializedMapsGeofenceGeometry.udId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedMapsGeofenceGeometry;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy