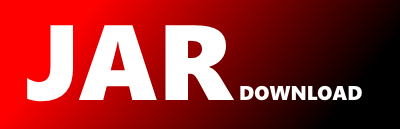
com.azure.messaging.eventgrid.systemevents.MediaLiveEventIncomingStreamsOutOfSyncEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Incoming streams out of sync event data. Schema of the data property of an EventGridEvent for a
* Microsoft.Media.LiveEventIncomingStreamsOutOfSync event.
*/
@Immutable
public final class MediaLiveEventIncomingStreamsOutOfSyncEventData
implements JsonSerializable {
/*
* Gets the minimum last timestamp received.
*/
private String minLastTimestamp;
/*
* Gets the type of stream with minimum last timestamp.
*/
private String typeOfStreamWithMinLastTimestamp;
/*
* Gets the maximum timestamp among all the tracks (audio or video).
*/
private String maxLastTimestamp;
/*
* Gets the type of stream with maximum last timestamp.
*/
private String typeOfStreamWithMaxLastTimestamp;
/*
* Gets the timescale in which "MinLastTimestamp" is represented.
*/
private String timescaleOfMinLastTimestamp;
/*
* Gets the timescale in which "MaxLastTimestamp" is represented.
*/
private String timescaleOfMaxLastTimestamp;
/**
* Creates an instance of MediaLiveEventIncomingStreamsOutOfSyncEventData class.
*/
public MediaLiveEventIncomingStreamsOutOfSyncEventData() {
}
/**
* Get the minLastTimestamp property: Gets the minimum last timestamp received.
*
* @return the minLastTimestamp value.
*/
public String getMinLastTimestamp() {
return this.minLastTimestamp;
}
/**
* Get the typeOfStreamWithMinLastTimestamp property: Gets the type of stream with minimum last timestamp.
*
* @return the typeOfStreamWithMinLastTimestamp value.
*/
public String getTypeOfStreamWithMinLastTimestamp() {
return this.typeOfStreamWithMinLastTimestamp;
}
/**
* Get the maxLastTimestamp property: Gets the maximum timestamp among all the tracks (audio or video).
*
* @return the maxLastTimestamp value.
*/
public String getMaxLastTimestamp() {
return this.maxLastTimestamp;
}
/**
* Get the typeOfStreamWithMaxLastTimestamp property: Gets the type of stream with maximum last timestamp.
*
* @return the typeOfStreamWithMaxLastTimestamp value.
*/
public String getTypeOfStreamWithMaxLastTimestamp() {
return this.typeOfStreamWithMaxLastTimestamp;
}
/**
* Get the timescaleOfMinLastTimestamp property: Gets the timescale in which "MinLastTimestamp" is represented.
*
* @return the timescaleOfMinLastTimestamp value.
*/
public String getTimescaleOfMinLastTimestamp() {
return this.timescaleOfMinLastTimestamp;
}
/**
* Get the timescaleOfMaxLastTimestamp property: Gets the timescale in which "MaxLastTimestamp" is represented.
*
* @return the timescaleOfMaxLastTimestamp value.
*/
public String getTimescaleOfMaxLastTimestamp() {
return this.timescaleOfMaxLastTimestamp;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MediaLiveEventIncomingStreamsOutOfSyncEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MediaLiveEventIncomingStreamsOutOfSyncEventData if the JsonReader was pointing to an
* instance of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MediaLiveEventIncomingStreamsOutOfSyncEventData.
*/
public static MediaLiveEventIncomingStreamsOutOfSyncEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MediaLiveEventIncomingStreamsOutOfSyncEventData deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData
= new MediaLiveEventIncomingStreamsOutOfSyncEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("minLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.minLastTimestamp = reader.getString();
} else if ("typeOfStreamWithMinLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.typeOfStreamWithMinLastTimestamp
= reader.getString();
} else if ("maxLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.maxLastTimestamp = reader.getString();
} else if ("typeOfStreamWithMaxLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.typeOfStreamWithMaxLastTimestamp
= reader.getString();
} else if ("timescaleOfMinLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.timescaleOfMinLastTimestamp
= reader.getString();
} else if ("timescaleOfMaxLastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData.timescaleOfMaxLastTimestamp
= reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedMediaLiveEventIncomingStreamsOutOfSyncEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy