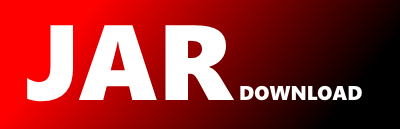
com.azure.messaging.eventgrid.systemevents.MediaLiveEventIngestHeartbeatEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Immutable;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Ingest heartbeat event data. Schema of the data property of an EventGridEvent for a
* Microsoft.Media.LiveEventIngestHeartbeat event.
*/
@Immutable
public final class MediaLiveEventIngestHeartbeatEventData
implements JsonSerializable {
/*
* Gets the type of the track (Audio / Video).
*/
private String trackType;
/*
* Gets the track name.
*/
private String trackName;
/*
* Gets the Live Transcription language.
*/
private String transcriptionLanguage;
/*
* Gets the Live Transcription state.
*/
private String transcriptionState;
/*
* Gets the bitrate of the track.
*/
private Long bitrate;
/*
* Gets the incoming bitrate.
*/
private Long incomingBitrate;
/*
* Gets the track ingest drift value.
*/
private String ingestDriftValue;
/*
* Gets the arrival UTC time of the last fragment.
*/
private String lastFragmentArrivalTime;
/*
* Gets the last timestamp.
*/
private String lastTimestamp;
/*
* Gets the timescale of the last timestamp.
*/
private String timescale;
/*
* Gets the fragment Overlap count.
*/
private Long overlapCount;
/*
* Gets the fragment Discontinuity count.
*/
private Long discontinuityCount;
/*
* Gets Non increasing count.
*/
private Long nonincreasingCount;
/*
* Gets a value indicating whether unexpected bitrate is present or not.
*/
private Boolean unexpectedBitrate;
/*
* Gets the state of the live event.
*/
private String state;
/*
* Gets a value indicating whether preview is healthy or not.
*/
private Boolean healthy;
static final ClientLogger LOGGER = new ClientLogger(MediaLiveEventIngestHeartbeatEventData.class);
/**
* Creates an instance of MediaLiveEventIngestHeartbeatEventData class.
*/
public MediaLiveEventIngestHeartbeatEventData() {
}
/**
* Get the trackType property: Gets the type of the track (Audio / Video).
*
* @return the trackType value.
*/
public String getTrackType() {
return this.trackType;
}
/**
* Get the trackName property: Gets the track name.
*
* @return the trackName value.
*/
public String getTrackName() {
return this.trackName;
}
/**
* Get the transcriptionLanguage property: Gets the Live Transcription language.
*
* @return the transcriptionLanguage value.
*/
public String getTranscriptionLanguage() {
return this.transcriptionLanguage;
}
/**
* Get the transcriptionState property: Gets the Live Transcription state.
*
* @return the transcriptionState value.
*/
public String getTranscriptionState() {
return this.transcriptionState;
}
/**
* Get the bitrate property: Gets the bitrate of the track.
*
* @return the bitrate value.
*/
public Long getBitrate() {
return this.bitrate;
}
/**
* Get the incomingBitrate property: Gets the incoming bitrate.
*
* @return the incomingBitrate value.
*/
public Long getIncomingBitrate() {
return this.incomingBitrate;
}
/**
* Get the ingestDriftValue property: Gets the track ingest drift value.
*
* @return the ingestDriftValue value.
*/
public Integer getIngestDriftValue() {
if ("n/a".equals(this.ingestDriftValue)) {
return null;
}
try {
return Integer.parseInt(this.ingestDriftValue);
} catch (NumberFormatException ex) {
LOGGER.logExceptionAsError(ex);
return null;
}
}
/**
* Get the lastFragmentArrivalTime property: Gets the arrival UTC time of the last fragment.
*
* @return the lastFragmentArrivalTime value.
*/
public OffsetDateTime getLastFragmentArrivalTime() {
return OffsetDateTime.parse(this.lastFragmentArrivalTime);
}
/**
* Get the lastTimestamp property: Gets the last timestamp.
*
* @return the lastTimestamp value.
*/
public String getLastTimestamp() {
return this.lastTimestamp;
}
/**
* Get the timescale property: Gets the timescale of the last timestamp.
*
* @return the timescale value.
*/
public String getTimescale() {
return this.timescale;
}
/**
* Get the overlapCount property: Gets the fragment Overlap count.
*
* @return the overlapCount value.
*/
public Long getOverlapCount() {
return this.overlapCount;
}
/**
* Get the discontinuityCount property: Gets the fragment Discontinuity count.
*
* @return the discontinuityCount value.
*/
public Long getDiscontinuityCount() {
return this.discontinuityCount;
}
/**
* Get the nonincreasingCount property: Gets Non increasing count.
*
* @return the nonincreasingCount value.
*/
public Long getNonincreasingCount() {
return this.nonincreasingCount;
}
/**
* Get the unexpectedBitrate property: Gets a value indicating whether unexpected bitrate is present or not.
*
* @return the unexpectedBitrate value.
*/
public Boolean isUnexpectedBitrate() {
return this.unexpectedBitrate;
}
/**
* Get the state property: Gets the state of the live event.
*
* @return the state value.
*/
public String getState() {
return this.state;
}
/**
* Get the healthy property: Gets a value indicating whether preview is healthy or not.
*
* @return the healthy value.
*/
public Boolean isHealthy() {
return this.healthy;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MediaLiveEventIngestHeartbeatEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MediaLiveEventIngestHeartbeatEventData if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MediaLiveEventIngestHeartbeatEventData.
*/
public static MediaLiveEventIngestHeartbeatEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MediaLiveEventIngestHeartbeatEventData deserializedMediaLiveEventIngestHeartbeatEventData
= new MediaLiveEventIngestHeartbeatEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("trackType".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.trackType = reader.getString();
} else if ("trackName".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.trackName = reader.getString();
} else if ("transcriptionLanguage".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.transcriptionLanguage = reader.getString();
} else if ("transcriptionState".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.transcriptionState = reader.getString();
} else if ("bitrate".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.bitrate
= reader.getNullable(JsonReader::getLong);
} else if ("incomingBitrate".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.incomingBitrate
= reader.getNullable(JsonReader::getLong);
} else if ("ingestDriftValue".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.ingestDriftValue = reader.getString();
} else if ("lastFragmentArrivalTime".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.lastFragmentArrivalTime = reader.getString();
} else if ("lastTimestamp".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.lastTimestamp = reader.getString();
} else if ("timescale".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.timescale = reader.getString();
} else if ("overlapCount".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.overlapCount
= reader.getNullable(JsonReader::getLong);
} else if ("discontinuityCount".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.discontinuityCount
= reader.getNullable(JsonReader::getLong);
} else if ("nonincreasingCount".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.nonincreasingCount
= reader.getNullable(JsonReader::getLong);
} else if ("unexpectedBitrate".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.unexpectedBitrate
= reader.getNullable(JsonReader::getBoolean);
} else if ("state".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.state = reader.getString();
} else if ("healthy".equals(fieldName)) {
deserializedMediaLiveEventIngestHeartbeatEventData.healthy
= reader.getNullable(JsonReader::getBoolean);
} else {
reader.skipChildren();
}
}
return deserializedMediaLiveEventIngestHeartbeatEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy