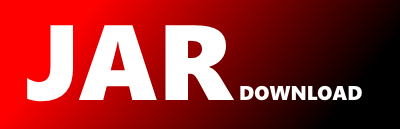
com.azure.messaging.eventgrid.systemevents.PolicyInsightsPolicyStateCreatedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.PolicyInsights.PolicyStateCreated event.
*/
@Fluent
public final class PolicyInsightsPolicyStateCreatedEventData
implements JsonSerializable {
/*
* The time that the resource was scanned by Azure Policy in the Universal ISO 8601 DateTime format
* yyyy-MM-ddTHH:mm:ss.fffffffZ.
*/
private OffsetDateTime timestamp;
/*
* The resource ID of the policy assignment.
*/
private String policyAssignmentId;
/*
* The resource ID of the policy definition.
*/
private String policyDefinitionId;
/*
* The reference ID for the policy definition inside the initiative definition, if the policy assignment is for an
* initiative. May be empty.
*/
private String policyDefinitionReferenceId;
/*
* The compliance state of the resource with respect to the policy assignment.
*/
private String complianceState;
/*
* The subscription ID of the resource.
*/
private String subscriptionId;
/*
* The compliance reason code. May be empty.
*/
private String complianceReasonCode;
/**
* Creates an instance of PolicyInsightsPolicyStateCreatedEventData class.
*/
public PolicyInsightsPolicyStateCreatedEventData() {
}
/**
* Get the timestamp property: The time that the resource was scanned by Azure Policy in the Universal ISO 8601
* DateTime format yyyy-MM-ddTHH:mm:ss.fffffffZ.
*
* @return the timestamp value.
*/
public OffsetDateTime getTimestamp() {
return this.timestamp;
}
/**
* Set the timestamp property: The time that the resource was scanned by Azure Policy in the Universal ISO 8601
* DateTime format yyyy-MM-ddTHH:mm:ss.fffffffZ.
*
* @param timestamp the timestamp value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setTimestamp(OffsetDateTime timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Get the policyAssignmentId property: The resource ID of the policy assignment.
*
* @return the policyAssignmentId value.
*/
public String getPolicyAssignmentId() {
return this.policyAssignmentId;
}
/**
* Set the policyAssignmentId property: The resource ID of the policy assignment.
*
* @param policyAssignmentId the policyAssignmentId value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setPolicyAssignmentId(String policyAssignmentId) {
this.policyAssignmentId = policyAssignmentId;
return this;
}
/**
* Get the policyDefinitionId property: The resource ID of the policy definition.
*
* @return the policyDefinitionId value.
*/
public String getPolicyDefinitionId() {
return this.policyDefinitionId;
}
/**
* Set the policyDefinitionId property: The resource ID of the policy definition.
*
* @param policyDefinitionId the policyDefinitionId value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setPolicyDefinitionId(String policyDefinitionId) {
this.policyDefinitionId = policyDefinitionId;
return this;
}
/**
* Get the policyDefinitionReferenceId property: The reference ID for the policy definition inside the initiative
* definition, if the policy assignment is for an initiative. May be empty.
*
* @return the policyDefinitionReferenceId value.
*/
public String getPolicyDefinitionReferenceId() {
return this.policyDefinitionReferenceId;
}
/**
* Set the policyDefinitionReferenceId property: The reference ID for the policy definition inside the initiative
* definition, if the policy assignment is for an initiative. May be empty.
*
* @param policyDefinitionReferenceId the policyDefinitionReferenceId value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData
setPolicyDefinitionReferenceId(String policyDefinitionReferenceId) {
this.policyDefinitionReferenceId = policyDefinitionReferenceId;
return this;
}
/**
* Get the complianceState property: The compliance state of the resource with respect to the policy assignment.
*
* @return the complianceState value.
*/
public String getComplianceState() {
return this.complianceState;
}
/**
* Set the complianceState property: The compliance state of the resource with respect to the policy assignment.
*
* @param complianceState the complianceState value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setComplianceState(String complianceState) {
this.complianceState = complianceState;
return this;
}
/**
* Get the subscriptionId property: The subscription ID of the resource.
*
* @return the subscriptionId value.
*/
public String getSubscriptionId() {
return this.subscriptionId;
}
/**
* Set the subscriptionId property: The subscription ID of the resource.
*
* @param subscriptionId the subscriptionId value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setSubscriptionId(String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* Get the complianceReasonCode property: The compliance reason code. May be empty.
*
* @return the complianceReasonCode value.
*/
public String getComplianceReasonCode() {
return this.complianceReasonCode;
}
/**
* Set the complianceReasonCode property: The compliance reason code. May be empty.
*
* @param complianceReasonCode the complianceReasonCode value to set.
* @return the PolicyInsightsPolicyStateCreatedEventData object itself.
*/
public PolicyInsightsPolicyStateCreatedEventData setComplianceReasonCode(String complianceReasonCode) {
this.complianceReasonCode = complianceReasonCode;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("timestamp",
this.timestamp == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.timestamp));
jsonWriter.writeStringField("policyAssignmentId", this.policyAssignmentId);
jsonWriter.writeStringField("policyDefinitionId", this.policyDefinitionId);
jsonWriter.writeStringField("policyDefinitionReferenceId", this.policyDefinitionReferenceId);
jsonWriter.writeStringField("complianceState", this.complianceState);
jsonWriter.writeStringField("subscriptionId", this.subscriptionId);
jsonWriter.writeStringField("complianceReasonCode", this.complianceReasonCode);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of PolicyInsightsPolicyStateCreatedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of PolicyInsightsPolicyStateCreatedEventData if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the PolicyInsightsPolicyStateCreatedEventData.
*/
public static PolicyInsightsPolicyStateCreatedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
PolicyInsightsPolicyStateCreatedEventData deserializedPolicyInsightsPolicyStateCreatedEventData
= new PolicyInsightsPolicyStateCreatedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("timestamp".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.timestamp = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("policyAssignmentId".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.policyAssignmentId = reader.getString();
} else if ("policyDefinitionId".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.policyDefinitionId = reader.getString();
} else if ("policyDefinitionReferenceId".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.policyDefinitionReferenceId
= reader.getString();
} else if ("complianceState".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.complianceState = reader.getString();
} else if ("subscriptionId".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.subscriptionId = reader.getString();
} else if ("complianceReasonCode".equals(fieldName)) {
deserializedPolicyInsightsPolicyStateCreatedEventData.complianceReasonCode = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedPolicyInsightsPolicyStateCreatedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy