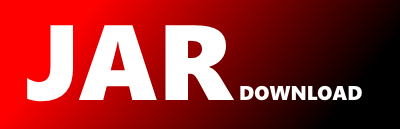
com.azure.messaging.eventgrid.systemevents.ResourceNotificationsResourceDeletedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Describes the schema of the common properties across all ARN system topic delete events.
*/
@Fluent
public class ResourceNotificationsResourceDeletedEventData
implements JsonSerializable {
/*
* resourceInfo details for delete event
*/
private ResourceNotificationsResourceDeletedDetails resourceDetails;
/*
* details about operational info
*/
private ResourceNotificationsOperationalDetails operationalDetails;
/**
* Creates an instance of ResourceNotificationsResourceDeletedEventData class.
*/
public ResourceNotificationsResourceDeletedEventData() {
}
/**
* Get the resourceDetails property: resourceInfo details for delete event.
*
* @return the resourceDetails value.
*/
public ResourceNotificationsResourceDeletedDetails getResourceDetails() {
return this.resourceDetails;
}
/**
* Set the resourceDetails property: resourceInfo details for delete event.
*
* @param resourceDetails the resourceDetails value to set.
* @return the ResourceNotificationsResourceDeletedEventData object itself.
*/
public ResourceNotificationsResourceDeletedEventData
setResourceDetails(ResourceNotificationsResourceDeletedDetails resourceDetails) {
this.resourceDetails = resourceDetails;
return this;
}
/**
* Get the operationalDetails property: details about operational info.
*
* @return the operationalDetails value.
*/
public ResourceNotificationsOperationalDetails getOperationalDetails() {
return this.operationalDetails;
}
/**
* Set the operationalDetails property: details about operational info.
*
* @param operationalDetails the operationalDetails value to set.
* @return the ResourceNotificationsResourceDeletedEventData object itself.
*/
public ResourceNotificationsResourceDeletedEventData
setOperationalDetails(ResourceNotificationsOperationalDetails operationalDetails) {
this.operationalDetails = operationalDetails;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("resourceInfo", this.resourceDetails);
jsonWriter.writeJsonField("operationalInfo", this.operationalDetails);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ResourceNotificationsResourceDeletedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ResourceNotificationsResourceDeletedEventData if the JsonReader was pointing to an
* instance of it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ResourceNotificationsResourceDeletedEventData.
*/
public static ResourceNotificationsResourceDeletedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ResourceNotificationsResourceDeletedEventData deserializedResourceNotificationsResourceDeletedEventData
= new ResourceNotificationsResourceDeletedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("resourceInfo".equals(fieldName)) {
deserializedResourceNotificationsResourceDeletedEventData.resourceDetails
= ResourceNotificationsResourceDeletedDetails.fromJson(reader);
} else if ("operationalInfo".equals(fieldName)) {
deserializedResourceNotificationsResourceDeletedEventData.operationalDetails
= ResourceNotificationsOperationalDetails.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedResourceNotificationsResourceDeletedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy