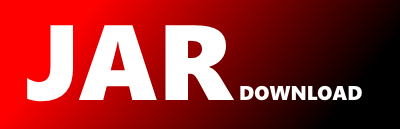
com.azure.messaging.eventgrid.systemevents.StorageBlobCreatedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Schema of the Data property of an EventGridEvent for a Microsoft.Storage.BlobCreated event.
*/
@Fluent
public final class StorageBlobCreatedEventData implements JsonSerializable {
/*
* The name of the API/operation that triggered this event.
*/
private String api;
/*
* A request id provided by the client of the storage API operation that triggered this event.
*/
private String clientRequestId;
/*
* The request id generated by the Storage service for the storage API operation that triggered this event.
*/
private String requestId;
/*
* The etag of the blob at the time this event was triggered.
*/
private String eTag;
/*
* The content type of the blob. This is the same as what would be returned in the Content-Type header from the
* blob.
*/
private String contentType;
/*
* The size of the blob in bytes. This is the same as what would be returned in the Content-Length header from the
* blob.
*/
private Long contentLength;
/*
* The offset of the blob in bytes.
*/
private Long contentOffset;
/*
* The type of blob.
*/
private String blobType;
/*
* The current tier of the blob.
*/
private StorageBlobAccessTier accessTier;
/*
* The path to the blob.
*/
private String url;
/*
* An opaque string value representing the logical sequence of events for any particular blob name. Users can use
* standard string comparison to understand the relative sequence of two events on the same blob name.
*/
private String sequencer;
/*
* The identity of the requester that triggered this event.
*/
private String identity;
/*
* For service use only. Diagnostic data occasionally included by the Azure Storage service. This property should be
* ignored by event consumers.
*/
private Object storageDiagnostics;
/**
* Creates an instance of StorageBlobCreatedEventData class.
*/
public StorageBlobCreatedEventData() {
}
/**
* Get the api property: The name of the API/operation that triggered this event.
*
* @return the api value.
*/
public String getApi() {
return this.api;
}
/**
* Set the api property: The name of the API/operation that triggered this event.
*
* @param api the api value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setApi(String api) {
this.api = api;
return this;
}
/**
* Get the clientRequestId property: A request id provided by the client of the storage API operation that triggered
* this event.
*
* @return the clientRequestId value.
*/
public String getClientRequestId() {
return this.clientRequestId;
}
/**
* Set the clientRequestId property: A request id provided by the client of the storage API operation that triggered
* this event.
*
* @param clientRequestId the clientRequestId value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setClientRequestId(String clientRequestId) {
this.clientRequestId = clientRequestId;
return this;
}
/**
* Get the requestId property: The request id generated by the Storage service for the storage API operation that
* triggered this event.
*
* @return the requestId value.
*/
public String getRequestId() {
return this.requestId;
}
/**
* Set the requestId property: The request id generated by the Storage service for the storage API operation that
* triggered this event.
*
* @param requestId the requestId value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Get the eTag property: The etag of the blob at the time this event was triggered.
*
* @return the eTag value.
*/
public String getETag() {
return this.eTag;
}
/**
* Set the eTag property: The etag of the blob at the time this event was triggered.
*
* @param eTag the eTag value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setETag(String eTag) {
this.eTag = eTag;
return this;
}
/**
* Get the contentType property: The content type of the blob. This is the same as what would be returned in the
* Content-Type header from the blob.
*
* @return the contentType value.
*/
public String getContentType() {
return this.contentType;
}
/**
* Set the contentType property: The content type of the blob. This is the same as what would be returned in the
* Content-Type header from the blob.
*
* @param contentType the contentType value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setContentType(String contentType) {
this.contentType = contentType;
return this;
}
/**
* Get the contentLength property: The size of the blob in bytes. This is the same as what would be returned in the
* Content-Length header from the blob.
*
* @return the contentLength value.
*/
public Long getContentLength() {
return this.contentLength;
}
/**
* Set the contentLength property: The size of the blob in bytes. This is the same as what would be returned in the
* Content-Length header from the blob.
*
* @param contentLength the contentLength value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setContentLength(Long contentLength) {
this.contentLength = contentLength;
return this;
}
/**
* Get the contentOffset property: The offset of the blob in bytes.
*
* @return the contentOffset value.
*/
public Long getContentOffset() {
return this.contentOffset;
}
/**
* Set the contentOffset property: The offset of the blob in bytes.
*
* @param contentOffset the contentOffset value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setContentOffset(Long contentOffset) {
this.contentOffset = contentOffset;
return this;
}
/**
* Get the blobType property: The type of blob.
*
* @return the blobType value.
*/
public String getBlobType() {
return this.blobType;
}
/**
* Set the blobType property: The type of blob.
*
* @param blobType the blobType value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setBlobType(String blobType) {
this.blobType = blobType;
return this;
}
/**
* Get the accessTier property: The current tier of the blob.
*
* @return the accessTier value.
*/
public StorageBlobAccessTier getAccessTier() {
return this.accessTier;
}
/**
* Set the accessTier property: The current tier of the blob.
*
* @param accessTier the accessTier value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setAccessTier(StorageBlobAccessTier accessTier) {
this.accessTier = accessTier;
return this;
}
/**
* Get the url property: The path to the blob.
*
* @return the url value.
*/
public String getUrl() {
return this.url;
}
/**
* Set the url property: The path to the blob.
*
* @param url the url value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setUrl(String url) {
this.url = url;
return this;
}
/**
* Get the sequencer property: An opaque string value representing the logical sequence of events for any particular
* blob name. Users can use standard string comparison to understand the relative sequence of two events on the same
* blob name.
*
* @return the sequencer value.
*/
public String getSequencer() {
return this.sequencer;
}
/**
* Set the sequencer property: An opaque string value representing the logical sequence of events for any particular
* blob name. Users can use standard string comparison to understand the relative sequence of two events on the same
* blob name.
*
* @param sequencer the sequencer value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setSequencer(String sequencer) {
this.sequencer = sequencer;
return this;
}
/**
* Get the identity property: The identity of the requester that triggered this event.
*
* @return the identity value.
*/
public String getIdentity() {
return this.identity;
}
/**
* Set the identity property: The identity of the requester that triggered this event.
*
* @param identity the identity value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setIdentity(String identity) {
this.identity = identity;
return this;
}
/**
* Get the storageDiagnostics property: For service use only. Diagnostic data occasionally included by the Azure
* Storage service. This property should be ignored by event consumers.
*
* @return the storageDiagnostics value.
*/
public Object getStorageDiagnostics() {
return this.storageDiagnostics;
}
/**
* Set the storageDiagnostics property: For service use only. Diagnostic data occasionally included by the Azure
* Storage service. This property should be ignored by event consumers.
*
* @param storageDiagnostics the storageDiagnostics value to set.
* @return the StorageBlobCreatedEventData object itself.
*/
public StorageBlobCreatedEventData setStorageDiagnostics(Object storageDiagnostics) {
this.storageDiagnostics = storageDiagnostics;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("accessTier", this.accessTier == null ? null : this.accessTier.toString());
jsonWriter.writeStringField("api", this.api);
jsonWriter.writeStringField("clientRequestId", this.clientRequestId);
jsonWriter.writeStringField("requestId", this.requestId);
jsonWriter.writeStringField("eTag", this.eTag);
jsonWriter.writeStringField("contentType", this.contentType);
jsonWriter.writeNumberField("contentLength", this.contentLength);
jsonWriter.writeNumberField("contentOffset", this.contentOffset);
jsonWriter.writeStringField("blobType", this.blobType);
jsonWriter.writeStringField("url", this.url);
jsonWriter.writeStringField("sequencer", this.sequencer);
jsonWriter.writeStringField("identity", this.identity);
jsonWriter.writeUntypedField("storageDiagnostics", this.storageDiagnostics);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StorageBlobCreatedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StorageBlobCreatedEventData if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the StorageBlobCreatedEventData.
*/
public static StorageBlobCreatedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StorageBlobCreatedEventData deserializedStorageBlobCreatedEventData = new StorageBlobCreatedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("accessTier".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.accessTier
= StorageBlobAccessTier.fromString(reader.getString());
} else if ("api".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.api = reader.getString();
} else if ("clientRequestId".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.clientRequestId = reader.getString();
} else if ("requestId".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.requestId = reader.getString();
} else if ("eTag".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.eTag = reader.getString();
} else if ("contentType".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.contentType = reader.getString();
} else if ("contentLength".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.contentLength = reader.getNullable(JsonReader::getLong);
} else if ("contentOffset".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.contentOffset = reader.getNullable(JsonReader::getLong);
} else if ("blobType".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.blobType = reader.getString();
} else if ("url".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.url = reader.getString();
} else if ("sequencer".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.sequencer = reader.getString();
} else if ("identity".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.identity = reader.getString();
} else if ("storageDiagnostics".equals(fieldName)) {
deserializedStorageBlobCreatedEventData.storageDiagnostics = reader.readUntyped();
} else {
reader.skipChildren();
}
}
return deserializedStorageBlobCreatedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy