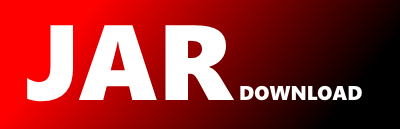
com.azure.messaging.eventgrid.systemevents.StorageTaskCompletedEventData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-messaging-eventgrid Show documentation
Show all versions of azure-messaging-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.messaging.eventgrid.systemevents;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Schema of the Data property of an EventGridEvent for an Microsoft.Storage.StorageTaskCompleted event.
*/
@Fluent
public final class StorageTaskCompletedEventData implements JsonSerializable {
/*
* The status for a storage task.
*/
private StorageTaskCompletedStatus status;
/*
* The time at which a storage task was completed.
*/
private OffsetDateTime completedDateTime;
/*
* The execution id for a storage task.
*/
private String taskExecutionId;
/*
* The task name for a storage task.
*/
private String taskName;
/*
* The summary report blob url for a storage task
*/
private String summaryReportBlobUrl;
/**
* Creates an instance of StorageTaskCompletedEventData class.
*/
public StorageTaskCompletedEventData() {
}
/**
* Get the status property: The status for a storage task.
*
* @return the status value.
*/
public StorageTaskCompletedStatus getStatus() {
return this.status;
}
/**
* Set the status property: The status for a storage task.
*
* @param status the status value to set.
* @return the StorageTaskCompletedEventData object itself.
*/
public StorageTaskCompletedEventData setStatus(StorageTaskCompletedStatus status) {
this.status = status;
return this;
}
/**
* Get the completedDateTime property: The time at which a storage task was completed.
*
* @return the completedDateTime value.
*/
public OffsetDateTime getCompletedDateTime() {
return this.completedDateTime;
}
/**
* Set the completedDateTime property: The time at which a storage task was completed.
*
* @param completedDateTime the completedDateTime value to set.
* @return the StorageTaskCompletedEventData object itself.
*/
public StorageTaskCompletedEventData setCompletedDateTime(OffsetDateTime completedDateTime) {
this.completedDateTime = completedDateTime;
return this;
}
/**
* Get the taskExecutionId property: The execution id for a storage task.
*
* @return the taskExecutionId value.
*/
public String getTaskExecutionId() {
return this.taskExecutionId;
}
/**
* Set the taskExecutionId property: The execution id for a storage task.
*
* @param taskExecutionId the taskExecutionId value to set.
* @return the StorageTaskCompletedEventData object itself.
*/
public StorageTaskCompletedEventData setTaskExecutionId(String taskExecutionId) {
this.taskExecutionId = taskExecutionId;
return this;
}
/**
* Get the taskName property: The task name for a storage task.
*
* @return the taskName value.
*/
public String getTaskName() {
return this.taskName;
}
/**
* Set the taskName property: The task name for a storage task.
*
* @param taskName the taskName value to set.
* @return the StorageTaskCompletedEventData object itself.
*/
public StorageTaskCompletedEventData setTaskName(String taskName) {
this.taskName = taskName;
return this;
}
/**
* Get the summaryReportBlobUrl property: The summary report blob url for a storage task.
*
* @return the summaryReportBlobUrl value.
*/
public String getSummaryReportBlobUrl() {
return this.summaryReportBlobUrl;
}
/**
* Set the summaryReportBlobUrl property: The summary report blob url for a storage task.
*
* @param summaryReportBlobUrl the summaryReportBlobUrl value to set.
* @return the StorageTaskCompletedEventData object itself.
*/
public StorageTaskCompletedEventData setSummaryReportBlobUrl(String summaryReportBlobUrl) {
this.summaryReportBlobUrl = summaryReportBlobUrl;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("status", this.status == null ? null : this.status.toString());
jsonWriter.writeStringField("completedDateTime",
this.completedDateTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.completedDateTime));
jsonWriter.writeStringField("taskExecutionId", this.taskExecutionId);
jsonWriter.writeStringField("taskName", this.taskName);
jsonWriter.writeStringField("summaryReportBlobUrl", this.summaryReportBlobUrl);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StorageTaskCompletedEventData from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StorageTaskCompletedEventData if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the StorageTaskCompletedEventData.
*/
public static StorageTaskCompletedEventData fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StorageTaskCompletedEventData deserializedStorageTaskCompletedEventData
= new StorageTaskCompletedEventData();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("status".equals(fieldName)) {
deserializedStorageTaskCompletedEventData.status
= StorageTaskCompletedStatus.fromString(reader.getString());
} else if ("completedDateTime".equals(fieldName)) {
deserializedStorageTaskCompletedEventData.completedDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("taskExecutionId".equals(fieldName)) {
deserializedStorageTaskCompletedEventData.taskExecutionId = reader.getString();
} else if ("taskName".equals(fieldName)) {
deserializedStorageTaskCompletedEventData.taskName = reader.getString();
} else if ("summaryReportBlobUrl".equals(fieldName)) {
deserializedStorageTaskCompletedEventData.summaryReportBlobUrl = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedStorageTaskCompletedEventData;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy