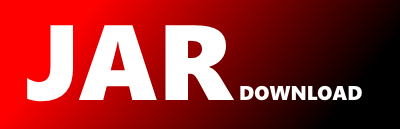
com.azure.messaging.eventhubs.EventHubProducerClient Maven / Gradle / Ivy
Show all versions of azure-messaging-eventhubs Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.messaging.eventhubs;
import com.azure.core.amqp.exception.AmqpException;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.util.IterableStream;
import com.azure.messaging.eventhubs.models.CreateBatchOptions;
import com.azure.messaging.eventhubs.models.SendOptions;
import java.io.Closeable;
import java.util.Objects;
/**
* A synchronous producer responsible for transmitting {@link EventData} to a specific Event Hub, grouped
* together in batches. Depending on the {@link CreateBatchOptions options} specified when creating an
* {@link EventDataBatch}, the events may be automatically routed to an available partition or specific to a partition.
* More information and specific recommendations for strategies to use when publishing events is in:
*
* Distribute events to partitions
*
* Allowing automatic routing of partitions is recommended when:
*
* - The sending of events needs to be highly available.
* - The event data should be evenly distributed among all available partitions.
*
*
* If no partition id is specified, the following rules are used for automatically selecting one:
*
* - Distribute the events equally amongst all available partitions using a round-robin approach.
* - If a partition becomes unavailable, the Event Hubs service will automatically detect it and forward the
* message to another available partition.
*
*
* The examples shown in this document use a credential object named DefaultAzureCredential for authentication,
* which is appropriate for most scenarios, including local development and production environments. Additionally, we
* recommend using
* managed identity
* for authentication in production environments. You can find more information on different ways of authenticating and
* their corresponding credential types in the
* Azure Identity documentation".
*
*
* Sample: Construct a {@link EventHubProducerClient}
*
* The following code sample demonstrates the creation of the synchronous client {@link EventHubProducerClient}.
* The {@code fullyQualifiedNamespace} is the Event Hubs Namespace's host name. It is listed under the "Essentials"
* panel after navigating to the Event Hubs Namespace via Azure Portal.
*
*
*
* TokenCredential credential = new DefaultAzureCredentialBuilder().build();
*
* // "<<fully-qualified-namespace>>" will look similar to "{your-namespace}.servicebus.windows.net"
* // "<<event-hub-name>>" will be the name of the Event Hub instance you created inside the Event Hubs namespace.
* EventHubProducerClient producer = new EventHubClientBuilder()
* .credential("<<fully-qualified-namespace>>", "<<event-hub-name>>",
* credential)
* .buildProducerClient();
*
*
*
* Sample: Create a producer and publish events to any partition
*
* The following code sample demonstrates publishing events and allowing the service to distribute the events
* round-robin between all partitions.
*
*
*
* TokenCredential credential = new DefaultAzureCredentialBuilder().build();
*
* EventHubProducerClient producer = new EventHubClientBuilder()
* .credential("<<fully-qualified-namespace>>", "<<event-hub-name>>",
* credential)
* .buildProducerClient();
*
* List<EventData> allEvents = Arrays.asList(new EventData("Foo"), new EventData("Bar"));
* EventDataBatch eventDataBatch = producer.createBatch();
*
* for (EventData eventData : allEvents) {
* if (!eventDataBatch.tryAdd(eventData)) {
* producer.send(eventDataBatch);
* eventDataBatch = producer.createBatch();
*
* // Try to add that event that couldn't fit before.
* if (!eventDataBatch.tryAdd(eventData)) {
* throw new IllegalArgumentException("Event is too large for an empty batch. Max size: "
* + eventDataBatch.getMaxSizeInBytes());
* }
* }
* }
*
* // send the last batch of remaining events
* if (eventDataBatch.getCount() > 0) {
* producer.send(eventDataBatch);
* }
*
* // Clients are expected to be long-lived objects.
* // Dispose of the producer to close any underlying resources when we are finished with it.
* producer.close();
*
*
*
* Sample: Publish events to partition "0"
*
* The following code sample demonstrates publishing events to a specific partition. In the scenario below, all
* events are sent to partition "0".
*
*
*
* TokenCredential credential = new DefaultAzureCredentialBuilder().build();
*
* EventHubProducerClient producer = new EventHubClientBuilder()
* .credential("<<fully-qualified-namespace>>", "<<event-hub-name>>",
* credential)
* .buildProducerClient();
*
* // Creating a batch with partitionId set will route all events in that batch to partition `0`.
* CreateBatchOptions options = new CreateBatchOptions().setPartitionId("0");
* EventDataBatch batch = producer.createBatch(options);
*
* // Add events to batch and when you want to send the batch, send it using the producer.
* producer.send(batch);
*
*
*
* Sample: Publish events to the same partition, grouped together using partition key
*
* The sample code below uses {@link CreateBatchOptions#setPartitionKey(String)} when creating the
* {@link EventDataBatch}. All events added to this batch will be published to the same partition. In general, events
* with the same {@code partitionKey} end up in the same partition.
*
*
*
* List<EventData> events = Arrays.asList(new EventData("sourdough"), new EventData("rye"),
* new EventData("wheat"));
*
* // Creating a batch with partitionKey set will tell the service to hash the partitionKey and decide which
* // partition to send the events to. Events with the same partitionKey are always routed to the same partition.
* CreateBatchOptions options = new CreateBatchOptions().setPartitionKey("bread");
* EventDataBatch batch = producer.createBatch(options);
*
* events.forEach(event -> batch.tryAdd(event));
* producer.send(batch);
*
*
*
* Sample: Publish events using a size-limited {@link EventDataBatch}
*
* The sample code below uses {@link CreateBatchOptions#setMaximumSizeInBytes(int)} when creating the
* {@link EventDataBatch}. In the example, it limits the size of the batch to 256 bytes. This is useful for scenarios
* where there are constraints like network throughput, memory, etc.
*
*
*
* List<EventData> telemetryEvents = Arrays.asList(firstEvent, secondEvent, thirdEvent);
*
* // Setting `setMaximumSizeInBytes` when creating a batch, limits the size of that batch.
* // In this case, all the batches created with these options are limited to 256 bytes.
* CreateBatchOptions options = new CreateBatchOptions().setMaximumSizeInBytes(256);
*
* EventDataBatch currentBatch = producer.createBatch(options);
*
* // For each telemetry event, we try to add it to the current batch.
* // When the batch is full, send it then create another batch to add more events to.
* for (EventData event : telemetryEvents) {
* if (!currentBatch.tryAdd(event)) {
* producer.send(currentBatch);
* currentBatch = producer.createBatch(options);
*
* // Add the event we couldn't before.
* if (!currentBatch.tryAdd(event)) {
* throw new IllegalArgumentException("Event is too large for an empty batch.");
* }
* }
* }
*
*
*
* @see com.azure.messaging.eventhubs
* @see EventHubClientBuilder
* @see EventHubProducerAsyncClient To asynchronously generate events to an Event Hub, see EventHubProducerAsyncClient.
*/
@ServiceClient(builder = EventHubClientBuilder.class)
public class EventHubProducerClient implements Closeable {
private final EventHubProducerAsyncClient producer;
/**
* Creates a new instance of {@link EventHubProducerClient} that sends messages to an Azure Event Hub.
*
* @throws NullPointerException if {@code producer} or {@code tryTimeout} is null.
*/
EventHubProducerClient(EventHubProducerAsyncClient producer) {
this.producer = Objects.requireNonNull(producer, "'producer' cannot be null.");
}
/**
* Gets the Event Hub name this client interacts with.
*
* @return The Event Hub name this client interacts with.
*/
public String getEventHubName() {
return producer.getEventHubName();
}
/**
* Gets the fully qualified Event Hubs namespace that the connection is associated with. This is likely similar to
* {@code {yournamespace}.servicebus.windows.net}.
*
* @return The fully qualified Event Hubs namespace that the connection is associated with.
*/
public String getFullyQualifiedNamespace() {
return producer.getFullyQualifiedNamespace();
}
/**
* Retrieves information about an Event Hub, including the number of partitions present and their identifiers.
*
* @return The set of information for the Event Hub that this client is associated with.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public EventHubProperties getEventHubProperties() {
return producer.getEventHubProperties().block();
}
/**
* Retrieves the identifiers for the partitions of an Event Hub.
*
* @return A Flux of identifiers for the partitions of an Event Hub.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public IterableStream getPartitionIds() {
return new IterableStream<>(producer.getPartitionIds());
}
/**
* Retrieves information about a specific partition for an Event Hub, including elements that describe the available
* events in the partition event stream.
*
* @param partitionId The unique identifier of a partition associated with the Event Hub.
* @return The set of information for the requested partition under the Event Hub this client is associated with.
* @throws NullPointerException if {@code partitionId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public PartitionProperties getPartitionProperties(String partitionId) {
return producer.getPartitionProperties(partitionId).block();
}
/**
* Creates an {@link EventDataBatch} that can fit as many events as the transport allows.
*
* @return A new {@link EventDataBatch} that can fit as many events as the transport allows.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public EventDataBatch createBatch() {
return producer.createBatch().block();
}
/**
* Creates an {@link EventDataBatch} configured with the options specified.
*
* @param options A set of options used to configure the {@link EventDataBatch}.
*
* @return A new {@link EventDataBatch} that can fit as many events as the transport allows.
*
* @throws NullPointerException if {@code options} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public EventDataBatch createBatch(CreateBatchOptions options) {
return producer.createBatch(options).block();
}
/**
* Sends a single event to the associated Event Hub. If the size of the single event exceeds the maximum size
* allowed, an exception will be triggered and the send will fail.
*
*
* For more information regarding the maximum event size allowed, see
* Azure Event Hubs Quotas and
* Limits.
*
*
* @param event Event to send to the service.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void send(EventData event) {
producer.send(event).block();
}
/**
* Sends a single event to the associated Event Hub with the send options. If the size of the single event exceeds
* the maximum size allowed, an exception will be triggered and the send will fail.
*
*
* For more information regarding the maximum event size allowed, see
* Azure Event Hubs Quotas and
* Limits.
*
*
* @param event Event to send to the service.
* @param options The set of options to consider when sending this event.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void send(EventData event, SendOptions options) {
producer.send(event, options).block();
}
/**
* Sends a set of events to the associated Event Hub using a batched approach. If the size of events exceed the
* maximum size of a single batch, an exception will be triggered and the send will fail. By default, the message
* size is the max amount allowed on the link.
*
*
*
* List<EventData> events = Arrays.asList(new EventData("maple"), new EventData("aspen"),
* new EventData("oak"));
* producer.send(events);
*
*
*
*
* For more information regarding the maximum event size allowed, see
* Azure Event Hubs Quotas and
* Limits.
*
*
* @param events Events to send to the service.
* @throws AmqpException if the size of {@code events} exceed the maximum size of a single batch.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void send(Iterable events) {
producer.send(events).block();
}
/**
* Sends a set of events to the associated Event Hub using a batched approach. If the size of events exceed the
* maximum size of a single batch, an exception will be triggered and the send will fail. By default, the message
* size is the max amount allowed on the link.
*
*
*
* TokenCredential credential = new DefaultAzureCredentialBuilder().build();
*
* EventHubProducerClient producer = new EventHubClientBuilder()
* .credential("<<fully-qualified-namespace>>", "<<event-hub-name>>",
* credential)
* .buildProducerClient();
*
* List<EventData> events = Arrays.asList(new EventData("Melbourne"), new EventData("London"),
* new EventData("New York"));
*
* SendOptions sendOptions = new SendOptions().setPartitionKey("cities");
* producer.send(events, sendOptions);
*
*
*
*
* For more information regarding the maximum event size allowed, see
* Azure Event Hubs Quotas and
* Limits.
*
*
* @param events Events to send to the service.
* @param options The set of options to consider when sending this batch.
* @throws AmqpException if the size of {@code events} exceed the maximum size of a single batch.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void send(Iterable events, SendOptions options) {
producer.send(events, options).block();
}
/**
* Sends the batch to the associated Event Hub.
*
* @param batch The batch to send to the service.
* @throws NullPointerException if {@code batch} is {@code null}.
* @see EventHubProducerClient#createBatch()
* @see EventHubProducerClient#createBatch(CreateBatchOptions)
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void send(EventDataBatch batch) {
producer.send(batch).block();
}
/**
* {@inheritDoc}
*/
@Override
public void close() {
producer.close();
}
/**
* Gets the client identifier.
*
* @return The unique identifier string for current client.
*/
public String getIdentifier() {
return producer.getIdentifier();
}
}