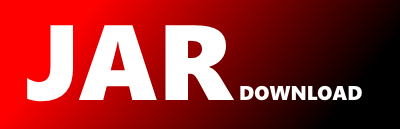
com.azure.security.attestation.models.AttestationOptions Maven / Gradle / Ivy
Show all versions of azure-security-attestation Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.security.attestation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.BinaryData;
/**
*
* AttestationOptions represent the parameters sent to the
* {@link com.azure.security.attestation.AttestationClient#attestOpenEnclave}
* or {@link com.azure.security.attestation.AttestationClient#attestSgxEnclave}API.
*
* Each {@link AttestationOptions} object expresses the options to attest an SGX enclave.
*
* An enclave (or Trusted Execution Environment) is a chunk of code that is isolated
* from its host. When code is running inside an enclave, there is a real challenge - if
* the customer wants to communicate with code in the enclave, there is no way of
* establishing a secure communication channel that can verify communication to the enclave.
*
*
*
* To support the "Secure Key Release" protocol which enables that communication, there are three
* key pieces of information required:
*
*
* - Attestation Evidence (typically an SGX quote or OpenEnclave report
* - InitTime Data - this is data specified when the TEE is created. (OPTIONAL)
* - RunTime Data - this can be a public key or other information. (OPTIONAL)
*
*
*
* To perform an attestation operation, you need at minimum a set of attestation evidence.
*
*
* For the Secure Key Release scenario, the InitTime Data and RunTime Data are expressed in the token
* returned by the Attestation Service, a relying party can use the InitTime Data to make decisions
* about whether the TEE can be trusted, and can use the RunTime Data as data which is known to have
* come from inside the enclave (if, for instance the RunTime Data is an asymmetric cryptographic
* key, it can be used to encrypt data that can only be decrypted by code inside the TEE).
*
*
*
* For both InitTime and RunTime data, the data can be expressed in the token as either BINARY or
* JSON data. This can simplify the evaluation process in the relying party.
*
*
*
*
* In addition to the evidence, InitTime and RunTime data, an Attest request can also take a "draft"
* attestation policy. This can be used to determine how an attestation policy effects the claims
* generated by the attestation service.
*
*
*
* Note that when a client specifies a draft attestation policy the returned attestation token will not
* be signed by the attestation service. That is to ensure that the token cannot be used by a relying
* party.
*
*
*
* The reason that both binary and JSON are represented as binary is that JSON encodings are ambiguous
* – there are many possible semantically identical JSON encodings of the same data. Since we’re going
* to take the SHA256 hash of the data, we accept a sequence of octets (byte[]) instead of a String
* (depending on your encoding, the same string can be encoded as different sequences of octets).
*
*
*/
@Fluent
public final class AttestationOptions {
private final BinaryData evidence;
private AttestationData runTimeData;
private AttestationData initTimeData;
private String draftPolicyForAttestation;
private AttestationTokenValidationOptions validationOptions;
/**
* Creates a new AttestOpenEnclaveRequest object with the OpenEnclave report from the enclave to be attested.
*
* The evidence will typically take the form of either an OpenEnclave report or an Intel SGX quote.
*
* Create an AttestationOptions from an SGX quote:
*
*
* AttestationOptions options = new AttestationOptions(sgxQuote);
*
*
* @param evidence to be used in the attest request.
*/
public AttestationOptions(BinaryData evidence) {
this.evidence = evidence;
}
/**
* Returns the "evidence" to be presented to the attestation service.
* @return The attestation evidence to be presented to the attestation service.
*/
public BinaryData getEvidence() {
return evidence;
}
/**
* Set the RunTime Data property.
* Runtime data provided by the enclave at the time the evidence
* was generated. The attestation service will verify that the first 32 bytes of the {@code report_data}
* field of the quote contains the SHA256 hash of the runtime data, this ensures that the
* RunTime Data was known to the enclave.
*
* When the attestation service generates the attestation token, the RunTimeData will
* be added as one of two possible claims: {@link AttestationResult#getRuntimeClaims()} if
* the {@link AttestationDataInterpretation} specified for the RunTime Data was set to "JSON", or
* {@link AttestationResult#getEnclaveHeldData()} if
* the {@link AttestationDataInterpretation} specified for the RunTime Data was set to "BINARY", or
*
*
Setting RunTime Data Property
*
*
* AttestationOptions optionsWithInitTimeData = new AttestationOptions(openEnclaveReport)
* .setInitTimeData(new AttestationData(inittimeData, AttestationDataInterpretation.BINARY));
*
*
* @param attestationData the runtimeData value to set.
* @return this {@link AttestationOptions} object itself.
*/
public AttestationOptions setRunTimeData(AttestationData attestationData) {
this.runTimeData = attestationData;
return this;
}
/**
* Retrieves the RunTimeData property to be sent to the service.
* Retrieve the RunTimeData value.
*
*
* AttestationData existingRuntimeData = attestationOptions.getRunTimeData();
*
*
* @return The RunTimeData value set by {@link AttestationOptions#setRunTimeData}
*/
public AttestationData getRunTimeData() {
return runTimeData != null ? new AttestationData(runTimeData) : null;
}
/**
*
* Set the initTimeData property: The initTimeData is data presented at the time that the
* execution environment was instantiated. The MAA will verify that the init data was
* known to the execution environment. Note that InitTimeData is invalid for CoffeeLake processors.
*
* When the attestation service generates the attestation token, the InitTimeData will
* be added as {@link AttestationResult#getInitTimeClaims()} if the {@link AttestationDataInterpretation}
* for the {@link AttestationData} is set to "JSON".
*
*
Setting InitTime Data Property
*
*
* AttestationOptions optionsWithInitTimeData = new AttestationOptions(openEnclaveReport)
* .setInitTimeData(new AttestationData(inittimeData, AttestationDataInterpretation.BINARY));
*
*
* @param attestationData the InitTimeData value to set.
* @return this {@link AttestationOptions} object itself.
*/
public AttestationOptions setInitTimeData(AttestationData attestationData) {
this.initTimeData = attestationData;
return this;
}
/**
* Retrieves the InitTimeData property to be sent to the service.
* Retrieve the InitTimeData value.
*
*
* AttestationOptions attestationOptions = new AttestationOptions(openEnclaveReport)
* .setInitTimeData(new AttestationData(inittimeData, AttestationDataInterpretation.JSON));
*
* AttestationData existingRuntimeData = attestationOptions.getInitTimeData();
*
*
* @return The InitTimeData value set by {@link AttestationOptions#setInitTimeData}
*/
public AttestationData getInitTimeData() {
return initTimeData != null ? new AttestationData(initTimeData) : null;
}
/**
* Set the draftPolicyForAttestation property: Attest against the provided draft policy.
* The setDraftPolicyForAttestation API can be used to
* determine how a proposed attestation policy would affect an attestation token.
* Note that the resulting token cannot be validated.
* Example of setting AttestationOptions with a draft policy.
*
*
* AttestationOptions request = new AttestationOptions(openEnclaveReport)
* .setDraftPolicyForAttestation("version=1.0; authorizationrules{=> permit();}; issuancerules{};");
*
*
* @param draftPolicyForAttestation the draftPolicyForAttestation value to set.
* @return this {@link AttestationOptions} object itself.
*/
public AttestationOptions setDraftPolicyForAttestation(String draftPolicyForAttestation) {
this.draftPolicyForAttestation = draftPolicyForAttestation;
return this;
}
/**
* Gets the draftPolicyForAttestation property which is used to attest against the draft policy.
*
* Gets the previously set draft policy for attestation.
*
*
* AttestationOptions getOptions = new AttestationOptions(openEnclaveReport)
* .setDraftPolicyForAttestation("version=1.0; authorizationrules{=> permit();}; issuancerules{};");
*
* String draftPolicy = getOptions.getDraftPolicyForAttestation();
*
*
* @return The draft policy if set.
*/
public String getDraftPolicyForAttestation() {
return this.draftPolicyForAttestation;
}
/**
* Sets the options used to validate attestation tokens returned from the service.
* @param validationOptions Token Validation options to be used to enhance the validations
* already performed by the SDK.
* @return this {@link AttestationOptions} object.
*/
public AttestationOptions setValidationOptions(AttestationTokenValidationOptions validationOptions) {
this.validationOptions = validationOptions;
return this;
}
/**
* Returns the options used for token validation.
* @return attestation token validation options.
*/
public AttestationTokenValidationOptions getValidationOptions() {
return validationOptions;
}
}