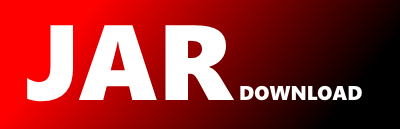
com.azure.storage.blob.changefeed.BlobChangefeedAsyncClient Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.storage.blob.changefeed;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import java.time.OffsetDateTime;
/**
* This class provides a client that contains all operations that apply to Azure Storage Blob changefeed.
*
* @see BlobChangefeedClientBuilder
*/
@ServiceClient(builder = BlobChangefeedClientBuilder.class, isAsync = true)
public class BlobChangefeedAsyncClient {
final ChangefeedFactory changefeedFactory;
/**
* Package-private constructor for use by {@link BlobChangefeedClientBuilder}.
*
* @param changefeedFactory The changefeed factory to create the Changefeed from.
*/
BlobChangefeedAsyncClient(ChangefeedFactory changefeedFactory) {
this.changefeedFactory = changefeedFactory;
}
/**
* Returns a reactive Publisher emitting all the changefeed events for this account lazily as needed.
*
*
* Changefeed events are returned in approximate temporal order.
*
*
For more information, see the
* Azure Docs.
*
*
Code Samples
*
*
*
* client.getEvents().subscribe(event ->
* System.out.printf("Topic: %s, Subject: %s%n", event.getTopic(), event.getSubject()));
*
*
*
* @return A reactive response emitting the changefeed events.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public BlobChangefeedPagedFlux getEvents() {
return getEvents(null, null);
}
/**
* Returns a reactive Publisher emitting all the changefeed events for this account lazily as needed.
*
*
* Changefeed events are returned in approximate temporal order.
*
*
For more information, see the
* Azure Docs.
*
*
Code Samples
*
*
*
* OffsetDateTime startTime = OffsetDateTime.MIN;
* OffsetDateTime endTime = OffsetDateTime.now();
*
* client.getEvents(startTime, endTime).subscribe(event ->
* System.out.printf("Topic: %s, Subject: %s%n", event.getTopic(), event.getSubject()));
*
*
*
* @param startTime Filters the results to return events approximately after the start time. Note: A few events
* belonging to the previous hour can also be returned. A few events belonging to this hour can be missing; to
* ensure all events from the hour are returned, round the start time down by an hour.
* @param endTime Filters the results to return events approximately before the end time. Note: A few events
* belonging to the next hour can also be returned. A few events belonging to this hour can be missing; to ensure
* all events from the hour are returned, round the end time up by an hour.
* @return A reactive response emitting the changefeed events.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public BlobChangefeedPagedFlux getEvents(OffsetDateTime startTime, OffsetDateTime endTime) {
return new BlobChangefeedPagedFlux(changefeedFactory, startTime, endTime);
}
/**
* Returns a reactive Publisher emitting all the changefeed events for this account lazily as needed.
*
*
* Changefeed events are returned in approximate temporal order.
*
*
For more information, see the
* Azure Docs.
*
*
Code Samples
*
*
*
* String cursor = "cursor";
*
* client.getEvents(cursor).subscribe(event ->
* System.out.printf("Topic: %s, Subject: %s%n", event.getTopic(), event.getSubject()));
*
*
*
* @param cursor Identifies the portion of the events to be returned with the next get operation. Events that
* take place after the event identified by the cursor will be returned.
* @return A reactive response emitting the changefeed events.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public BlobChangefeedPagedFlux getEvents(String cursor) {
return new BlobChangefeedPagedFlux(changefeedFactory, cursor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy