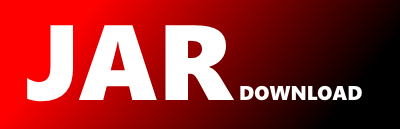
com.azure.storage.blob.changefeed.Segment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob-changefeed Show documentation
Show all versions of azure-storage-blob-changefeed Show documentation
This module contains client library for Microsoft Azure Blob Storage changefeed.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.storage.blob.changefeed;
import com.azure.storage.blob.BlobContainerAsyncClient;
import com.azure.storage.blob.changefeed.implementation.models.BlobChangefeedEventWrapper;
import com.azure.storage.blob.changefeed.implementation.models.ChangefeedCursor;
import com.azure.storage.blob.changefeed.implementation.models.SegmentCursor;
import com.azure.storage.blob.changefeed.implementation.models.ShardCursor;
import com.azure.storage.blob.changefeed.implementation.util.DownloadUtils;
import reactor.core.publisher.Flux;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Represents a Segment in Changefeed.
*
* A segment is a blob that points to a manifest file.
* The segment manifest file (meta.json) shows the path of the change feed files for that segment in the
* chunkFilePaths property. (Note: these chunkFilePaths are really shardPaths in this implementation.)
* The chunkFilePaths property looks something like this.
* { ...
* "chunkFilePaths": [
* "$blobchangefeed/log/00/2019/02/22/1810/",
* "$blobchangefeed/log/01/2019/02/22/1810/"
* ],
* ...}
*/
class Segment {
private static final String CHUNK_FILE_PATHS = "chunkFilePaths";
private final BlobContainerAsyncClient client; /* Changefeed container */
private final String segmentPath; /* Segment manifest location. */
private final ChangefeedCursor changefeedCursor; /* Cursor associated with parent changefeed. */
private final SegmentCursor userCursor; /* User provided cursor. */
private final ShardFactory shardFactory;
/**
* Creates a new Segment.
*/
Segment(BlobContainerAsyncClient client, String segmentPath, ChangefeedCursor changefeedCursor,
SegmentCursor userCursor, ShardFactory shardFactory) {
this.client = client;
this.segmentPath = segmentPath;
this.changefeedCursor = changefeedCursor;
this.userCursor = userCursor;
this.shardFactory = shardFactory;
}
/**
* Get all the events for the Segment.
* @return A reactive stream of {@link BlobChangefeedEventWrapper}
*/
Flux getEvents() {
/* Download JSON manifest file. */
/* We can keep the entire metadata file in memory since it is expected to only be a few hundred bytes. */
return DownloadUtils.downloadToByteArray(client, segmentPath)
.flatMap(DownloadUtils::parseJson)
/* Parse the shards from the manifest. */
.flatMapIterable(this::getShards)
/* Get all events for each shard. */
.concatMap(Shard::getEvents);
}
@SuppressWarnings("unchecked")
private List getShards(Map node) {
List shards = new ArrayList<>();
/* Iterate over each shard element. */
for (Object shard : (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy