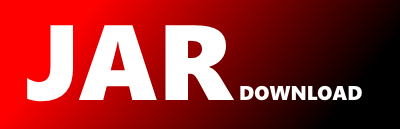
com.azure.storage.blob.implementation.ServicesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
This module contains client library for Microsoft Azure Blob Storage.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.ResponseBase;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.http.rest.StreamResponse;
import com.azure.core.util.BinaryData;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.storage.blob.implementation.models.BlobContainersSegment;
import com.azure.storage.blob.implementation.models.BlobStorageExceptionInternal;
import com.azure.storage.blob.implementation.models.FilterBlobSegment;
import com.azure.storage.blob.implementation.models.FilterBlobsIncludeItem;
import com.azure.storage.blob.implementation.models.ServicesFilterBlobsHeaders;
import com.azure.storage.blob.implementation.models.ServicesGetAccountInfoHeaders;
import com.azure.storage.blob.implementation.models.ServicesGetPropertiesHeaders;
import com.azure.storage.blob.implementation.models.ServicesGetStatisticsHeaders;
import com.azure.storage.blob.implementation.models.ServicesGetUserDelegationKeyHeaders;
import com.azure.storage.blob.implementation.models.ServicesListBlobContainersSegmentHeaders;
import com.azure.storage.blob.implementation.models.ServicesListBlobContainersSegmentNextHeaders;
import com.azure.storage.blob.implementation.models.ServicesSetPropertiesHeaders;
import com.azure.storage.blob.implementation.models.ServicesSubmitBatchHeaders;
import com.azure.storage.blob.models.BlobContainerItem;
import com.azure.storage.blob.models.BlobServiceProperties;
import com.azure.storage.blob.models.BlobServiceStatistics;
import com.azure.storage.blob.models.KeyInfo;
import com.azure.storage.blob.models.ListBlobContainersIncludeType;
import com.azure.storage.blob.models.UserDelegationKey;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import com.azure.storage.blob.implementation.util.ModelHelper;
/**
* An instance of this class provides access to all the operations defined in Services.
*/
public final class ServicesImpl {
/**
* The proxy service used to perform REST calls.
*/
private final ServicesService service;
/**
* The service client containing this operation class.
*/
private final AzureBlobStorageImpl client;
/**
* Initializes an instance of ServicesImpl.
*
* @param client the instance of the service client containing this operation class.
*/
ServicesImpl(AzureBlobStorageImpl client) {
this.service = RestProxy.create(ServicesService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for AzureBlobStorageServices to be used by the proxy service to perform
* REST calls.
*/
@Host("{url}")
@ServiceInterface(name = "AzureBlobStorageServ")
public interface ServicesService {
@Put("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> setProperties(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId,
@BodyParam("application/xml") BlobServiceProperties blobServiceProperties,
@HeaderParam("Accept") String accept, Context context);
@Put("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> setPropertiesNoCustomHeaders(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId,
@BodyParam("application/xml") BlobServiceProperties blobServiceProperties,
@HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getProperties(
@HostParam("url") String url, @QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getPropertiesNoCustomHeaders(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getStatistics(
@HostParam("url") String url, @QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getStatisticsNoCustomHeaders(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> listBlobContainersSegment(
@HostParam("url") String url, @QueryParam("comp") String comp, @QueryParam("prefix") String prefix,
@QueryParam("marker") String marker, @QueryParam("maxresults") Integer maxresults,
@QueryParam("include") String listBlobContainersIncludeType, @QueryParam("timeout") Integer timeout,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> listBlobContainersSegmentNoCustomHeaders(@HostParam("url") String url,
@QueryParam("comp") String comp, @QueryParam("prefix") String prefix, @QueryParam("marker") String marker,
@QueryParam("maxresults") Integer maxresults, @QueryParam("include") String listBlobContainersIncludeType,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Post("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getUserDelegationKey(
@HostParam("url") String url, @QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @BodyParam("application/xml") KeyInfo keyInfo,
@HeaderParam("Accept") String accept, Context context);
@Post("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getUserDelegationKeyNoCustomHeaders(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @BodyParam("application/xml") KeyInfo keyInfo,
@HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getAccountInfo(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@HeaderParam("x-ms-version") String version, @HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> getAccountInfoNoCustomHeaders(@HostParam("url") String url,
@QueryParam("restype") String restype, @QueryParam("comp") String comp,
@HeaderParam("x-ms-version") String version, @HeaderParam("Accept") String accept, Context context);
@Post("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono>> submitBatch(@HostParam("url") String url,
@QueryParam("comp") String comp, @HeaderParam("Content-Length") long contentLength,
@HeaderParam("Content-Type") String multipartContentType, @QueryParam("timeout") Integer timeout,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@BodyParam("application/xml") Flux body, @HeaderParam("Accept") String accept, Context context);
@Post("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono submitBatchNoCustomHeaders(@HostParam("url") String url, @QueryParam("comp") String comp,
@HeaderParam("Content-Length") long contentLength, @HeaderParam("Content-Type") String multipartContentType,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId,
@BodyParam("application/xml") Flux body, @HeaderParam("Accept") String accept, Context context);
@Post("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono>> submitBatch(@HostParam("url") String url,
@QueryParam("comp") String comp, @HeaderParam("Content-Length") long contentLength,
@HeaderParam("Content-Type") String multipartContentType, @QueryParam("timeout") Integer timeout,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@BodyParam("application/xml") BinaryData body, @HeaderParam("Accept") String accept, Context context);
@Post("/")
@ExpectedResponses({ 202 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono submitBatchNoCustomHeaders(@HostParam("url") String url, @QueryParam("comp") String comp,
@HeaderParam("Content-Length") long contentLength, @HeaderParam("Content-Type") String multipartContentType,
@QueryParam("timeout") Integer timeout, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @BodyParam("application/xml") BinaryData body,
@HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> filterBlobs(@HostParam("url") String url,
@QueryParam("comp") String comp, @QueryParam("timeout") Integer timeout,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@QueryParam("where") String where, @QueryParam("marker") String marker,
@QueryParam("maxresults") Integer maxresults, @QueryParam("include") String include,
@HeaderParam("Accept") String accept, Context context);
@Get("/")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> filterBlobsNoCustomHeaders(@HostParam("url") String url,
@QueryParam("comp") String comp, @QueryParam("timeout") Integer timeout,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@QueryParam("where") String where, @QueryParam("marker") String marker,
@QueryParam("maxresults") Integer maxresults, @QueryParam("include") String include,
@HeaderParam("Accept") String accept, Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono>
listBlobContainersSegmentNext(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("url") String url, @HeaderParam("x-ms-version") String version,
@HeaderParam("x-ms-client-request-id") String requestId, @HeaderParam("Accept") String accept,
Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(BlobStorageExceptionInternal.class)
Mono> listBlobContainersSegmentNextNoCustomHeaders(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("url") String url,
@HeaderParam("x-ms-version") String version, @HeaderParam("x-ms-client-request-id") String requestId,
@HeaderParam("Accept") String accept, Context context);
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
setPropertiesWithResponseAsync(BlobServiceProperties blobServiceProperties, Integer timeout, String requestId) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.setProperties(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, blobServiceProperties, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> setPropertiesWithResponseAsync(
BlobServiceProperties blobServiceProperties, Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return service
.setProperties(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(), requestId,
blobServiceProperties, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono setPropertiesAsync(BlobServiceProperties blobServiceProperties, Integer timeout,
String requestId) {
return setPropertiesWithResponseAsync(blobServiceProperties, timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(ignored -> Mono.empty());
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono setPropertiesAsync(BlobServiceProperties blobServiceProperties, Integer timeout, String requestId,
Context context) {
return setPropertiesWithResponseAsync(blobServiceProperties, timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(ignored -> Mono.empty());
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> setPropertiesNoCustomHeadersWithResponseAsync(
BlobServiceProperties blobServiceProperties, Integer timeout, String requestId) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.setPropertiesNoCustomHeaders(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, blobServiceProperties, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Sets properties for a storage account's Blob service endpoint, including properties for Storage Analytics and
* CORS (Cross-Origin Resource Sharing) rules.
*
* @param blobServiceProperties The StorageService properties.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> setPropertiesNoCustomHeadersWithResponseAsync(
BlobServiceProperties blobServiceProperties, Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return service
.setPropertiesNoCustomHeaders(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(),
requestId, blobServiceProperties, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getPropertiesWithResponseAsync(Integer timeout, String requestId) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getProperties(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getPropertiesWithResponseAsync(Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return service
.getProperties(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(), requestId, accept,
context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getPropertiesAsync(Integer timeout, String requestId) {
return getPropertiesWithResponseAsync(timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getPropertiesAsync(Integer timeout, String requestId, Context context) {
return getPropertiesWithResponseAsync(timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getPropertiesNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getPropertiesNoCustomHeaders(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* gets the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the properties of a storage account's Blob service, including properties for Storage Analytics and CORS
* (Cross-Origin Resource Sharing) rules along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getPropertiesNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId, Context context) {
final String restype = "service";
final String comp = "properties";
final String accept = "application/xml";
return service
.getPropertiesNoCustomHeaders(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(),
requestId, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getStatisticsWithResponseAsync(Integer timeout, String requestId) {
final String restype = "service";
final String comp = "stats";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getStatistics(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getStatisticsWithResponseAsync(Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "stats";
final String accept = "application/xml";
return service
.getStatistics(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(), requestId, accept,
context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getStatisticsAsync(Integer timeout, String requestId) {
return getStatisticsWithResponseAsync(timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getStatisticsAsync(Integer timeout, String requestId, Context context) {
return getStatisticsWithResponseAsync(timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getStatisticsNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId) {
final String restype = "service";
final String comp = "stats";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getStatisticsNoCustomHeaders(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves statistics related to replication for the Blob service. It is only available on the secondary location
* endpoint when read-access geo-redundant replication is enabled for the storage account.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return stats for the storage service along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getStatisticsNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId, Context context) {
final String restype = "service";
final String comp = "stats";
final String accept = "application/xml";
return service
.getStatisticsNoCustomHeaders(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(),
requestId, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentSinglePageAsync(String prefix, String marker,
Integer maxresults, List listBlobContainersIncludeType, Integer timeout,
String requestId) {
final String comp = "list";
final String accept = "application/xml";
String listBlobContainersIncludeTypeConverted = (listBlobContainersIncludeType == null)
? null
: listBlobContainersIncludeType.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return FluxUtil
.withContext(context -> service.listBlobContainersSegment(this.client.getUrl(), comp, prefix, marker,
maxresults, listBlobContainersIncludeTypeConverted, timeout, this.client.getVersion(), requestId,
accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), res.getDeserializedHeaders()));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentSinglePageAsync(String prefix, String marker,
Integer maxresults, List listBlobContainersIncludeType, Integer timeout,
String requestId, Context context) {
final String comp = "list";
final String accept = "application/xml";
String listBlobContainersIncludeTypeConverted = (listBlobContainersIncludeType == null)
? null
: listBlobContainersIncludeType.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return service
.listBlobContainersSegment(this.client.getUrl(), comp, prefix, marker, maxresults,
listBlobContainersIncludeTypeConverted, timeout, this.client.getVersion(), requestId, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), res.getDeserializedHeaders()));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listBlobContainersSegmentAsync(String prefix, String marker, Integer maxresults,
List listBlobContainersIncludeType, Integer timeout, String requestId) {
return new PagedFlux<>(() -> listBlobContainersSegmentSinglePageAsync(prefix, marker, maxresults,
listBlobContainersIncludeType, timeout, requestId),
nextLink -> listBlobContainersSegmentNextSinglePageAsync(nextLink, requestId));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listBlobContainersSegmentAsync(String prefix, String marker, Integer maxresults,
List listBlobContainersIncludeType, Integer timeout, String requestId,
Context context) {
return new PagedFlux<>(
() -> listBlobContainersSegmentSinglePageAsync(prefix, marker, maxresults, listBlobContainersIncludeType,
timeout, requestId, context),
nextLink -> listBlobContainersSegmentNextSinglePageAsync(nextLink, requestId, context));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentNoCustomHeadersSinglePageAsync(String prefix,
String marker, Integer maxresults, List listBlobContainersIncludeType,
Integer timeout, String requestId) {
final String comp = "list";
final String accept = "application/xml";
String listBlobContainersIncludeTypeConverted = (listBlobContainersIncludeType == null)
? null
: listBlobContainersIncludeType.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return FluxUtil
.withContext(context -> service.listBlobContainersSegmentNoCustomHeaders(this.client.getUrl(), comp, prefix,
marker, maxresults, listBlobContainersIncludeTypeConverted, timeout, this.client.getVersion(),
requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), null));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentNoCustomHeadersSinglePageAsync(String prefix,
String marker, Integer maxresults, List listBlobContainersIncludeType,
Integer timeout, String requestId, Context context) {
final String comp = "list";
final String accept = "application/xml";
String listBlobContainersIncludeTypeConverted = (listBlobContainersIncludeType == null)
? null
: listBlobContainersIncludeType.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return service
.listBlobContainersSegmentNoCustomHeaders(this.client.getUrl(), comp, prefix, marker, maxresults,
listBlobContainersIncludeTypeConverted, timeout, this.client.getVersion(), requestId, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), null));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listBlobContainersSegmentNoCustomHeadersAsync(String prefix, String marker,
Integer maxresults, List listBlobContainersIncludeType, Integer timeout,
String requestId) {
return new PagedFlux<>(
() -> listBlobContainersSegmentNoCustomHeadersSinglePageAsync(prefix, marker, maxresults,
listBlobContainersIncludeType, timeout, requestId),
nextLink -> listBlobContainersSegmentNextSinglePageAsync(nextLink, requestId));
}
/**
* The List Containers Segment operation returns a list of the containers under the specified account.
*
* @param prefix Filters the results to return only containers whose name begins with the specified prefix.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param listBlobContainersIncludeType Include this parameter to specify that the container's metadata be returned
* as part of the response body.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listBlobContainersSegmentNoCustomHeadersAsync(String prefix, String marker,
Integer maxresults, List listBlobContainersIncludeType, Integer timeout,
String requestId, Context context) {
return new PagedFlux<>(
() -> listBlobContainersSegmentNoCustomHeadersSinglePageAsync(prefix, marker, maxresults,
listBlobContainersIncludeType, timeout, requestId, context),
nextLink -> listBlobContainersSegmentNextSinglePageAsync(nextLink, requestId, context));
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getUserDelegationKeyWithResponseAsync(KeyInfo keyInfo, Integer timeout, String requestId) {
final String restype = "service";
final String comp = "userdelegationkey";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getUserDelegationKey(this.client.getUrl(), restype, comp, timeout,
this.client.getVersion(), requestId, keyInfo, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getUserDelegationKeyWithResponseAsync(KeyInfo keyInfo, Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "userdelegationkey";
final String accept = "application/xml";
return service
.getUserDelegationKey(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(), requestId,
keyInfo, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getUserDelegationKeyAsync(KeyInfo keyInfo, Integer timeout, String requestId) {
return getUserDelegationKeyWithResponseAsync(keyInfo, timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getUserDelegationKeyAsync(KeyInfo keyInfo, Integer timeout, String requestId,
Context context) {
return getUserDelegationKeyWithResponseAsync(keyInfo, timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getUserDelegationKeyNoCustomHeadersWithResponseAsync(KeyInfo keyInfo,
Integer timeout, String requestId) {
final String restype = "service";
final String comp = "userdelegationkey";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getUserDelegationKeyNoCustomHeaders(this.client.getUrl(), restype, comp,
timeout, this.client.getVersion(), requestId, keyInfo, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Retrieves a user delegation key for the Blob service. This is only a valid operation when using bearer token
* authentication.
*
* @param keyInfo Key information.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a user delegation key along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getUserDelegationKeyNoCustomHeadersWithResponseAsync(KeyInfo keyInfo,
Integer timeout, String requestId, Context context) {
final String restype = "service";
final String comp = "userdelegationkey";
final String accept = "application/xml";
return service
.getUserDelegationKeyNoCustomHeaders(this.client.getUrl(), restype, comp, timeout, this.client.getVersion(),
requestId, keyInfo, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Returns the sku name and account kind.
*
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getAccountInfoWithResponseAsync() {
final String restype = "account";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getAccountInfo(this.client.getUrl(), restype, comp,
this.client.getVersion(), accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Returns the sku name and account kind.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getAccountInfoWithResponseAsync(Context context) {
final String restype = "account";
final String comp = "properties";
final String accept = "application/xml";
return service.getAccountInfo(this.client.getUrl(), restype, comp, this.client.getVersion(), accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Returns the sku name and account kind.
*
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getAccountInfoAsync() {
return getAccountInfoWithResponseAsync()
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(ignored -> Mono.empty());
}
/**
* Returns the sku name and account kind.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getAccountInfoAsync(Context context) {
return getAccountInfoWithResponseAsync(context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(ignored -> Mono.empty());
}
/**
* Returns the sku name and account kind.
*
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getAccountInfoNoCustomHeadersWithResponseAsync() {
final String restype = "account";
final String comp = "properties";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.getAccountInfoNoCustomHeaders(this.client.getUrl(), restype, comp,
this.client.getVersion(), accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Returns the sku name and account kind.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getAccountInfoNoCustomHeadersWithResponseAsync(Context context) {
final String restype = "account";
final String comp = "properties";
final String accept = "application/xml";
return service
.getAccountInfoNoCustomHeaders(this.client.getUrl(), restype, comp, this.client.getVersion(), accept,
context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> submitBatchWithResponseAsync(
long contentLength, String multipartContentType, Flux body, Integer timeout, String requestId) {
final String comp = "batch";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.submitBatch(this.client.getUrl(), comp, contentLength, multipartContentType,
timeout, this.client.getVersion(), requestId, body, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> submitBatchWithResponseAsync(
long contentLength, String multipartContentType, Flux body, Integer timeout, String requestId,
Context context) {
final String comp = "batch";
final String accept = "application/xml";
return service
.submitBatch(this.client.getUrl(), comp, contentLength, multipartContentType, timeout,
this.client.getVersion(), requestId, body, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Flux submitBatchAsync(long contentLength, String multipartContentType, Flux body,
Integer timeout, String requestId) {
return submitBatchWithResponseAsync(contentLength, multipartContentType, body, timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMapMany(fluxByteBufferResponse -> fluxByteBufferResponse.getValue());
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Flux submitBatchAsync(long contentLength, String multipartContentType, Flux body,
Integer timeout, String requestId, Context context) {
return submitBatchWithResponseAsync(contentLength, multipartContentType, body, timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMapMany(fluxByteBufferResponse -> fluxByteBufferResponse.getValue());
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono submitBatchNoCustomHeadersWithResponseAsync(long contentLength,
String multipartContentType, Flux body, Integer timeout, String requestId) {
final String comp = "batch";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.submitBatchNoCustomHeaders(this.client.getUrl(), comp, contentLength,
multipartContentType, timeout, this.client.getVersion(), requestId, body, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono submitBatchNoCustomHeadersWithResponseAsync(long contentLength,
String multipartContentType, Flux body, Integer timeout, String requestId, Context context) {
final String comp = "batch";
final String accept = "application/xml";
return service
.submitBatchNoCustomHeaders(this.client.getUrl(), comp, contentLength, multipartContentType, timeout,
this.client.getVersion(), requestId, body, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> submitBatchWithResponseAsync(
long contentLength, String multipartContentType, BinaryData body, Integer timeout, String requestId) {
final String comp = "batch";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.submitBatch(this.client.getUrl(), comp, contentLength, multipartContentType,
timeout, this.client.getVersion(), requestId, body, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link ResponseBase} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> submitBatchWithResponseAsync(
long contentLength, String multipartContentType, BinaryData body, Integer timeout, String requestId,
Context context) {
final String comp = "batch";
final String accept = "application/xml";
return service
.submitBatch(this.client.getUrl(), comp, contentLength, multipartContentType, timeout,
this.client.getVersion(), requestId, body, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Flux submitBatchAsync(long contentLength, String multipartContentType, BinaryData body,
Integer timeout, String requestId) {
return submitBatchWithResponseAsync(contentLength, multipartContentType, body, timeout, requestId)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMapMany(fluxByteBufferResponse -> fluxByteBufferResponse.getValue());
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Flux submitBatchAsync(long contentLength, String multipartContentType, BinaryData body,
Integer timeout, String requestId, Context context) {
return submitBatchWithResponseAsync(contentLength, multipartContentType, body, timeout, requestId, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMapMany(fluxByteBufferResponse -> fluxByteBufferResponse.getValue());
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono submitBatchNoCustomHeadersWithResponseAsync(long contentLength,
String multipartContentType, BinaryData body, Integer timeout, String requestId) {
final String comp = "batch";
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.submitBatchNoCustomHeaders(this.client.getUrl(), comp, contentLength,
multipartContentType, timeout, this.client.getVersion(), requestId, body, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Batch operation allows multiple API calls to be embedded into a single HTTP request.
*
* @param contentLength The length of the request.
* @param multipartContentType Required. The value of this header must be multipart/mixed with a batch boundary.
* Example header value: multipart/mixed; boundary=batch_<GUID>.
* @param body Initial data.
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono submitBatchNoCustomHeadersWithResponseAsync(long contentLength,
String multipartContentType, BinaryData body, Integer timeout, String requestId, Context context) {
final String comp = "batch";
final String accept = "application/xml";
return service
.submitBatchNoCustomHeaders(this.client.getUrl(), comp, contentLength, multipartContentType, timeout,
this.client.getVersion(), requestId, body, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call along with {@link ResponseBase} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> filterBlobsWithResponseAsync(
Integer timeout, String requestId, String where, String marker, Integer maxresults,
List include) {
final String comp = "blobs";
final String accept = "application/xml";
String includeConverted = (include == null)
? null
: include.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return FluxUtil
.withContext(context -> service.filterBlobs(this.client.getUrl(), comp, timeout, this.client.getVersion(),
requestId, where, marker, maxresults, includeConverted, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call along with {@link ResponseBase} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> filterBlobsWithResponseAsync(
Integer timeout, String requestId, String where, String marker, Integer maxresults,
List include, Context context) {
final String comp = "blobs";
final String accept = "application/xml";
String includeConverted = (include == null)
? null
: include.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return service
.filterBlobs(this.client.getUrl(), comp, timeout, this.client.getVersion(), requestId, where, marker,
maxresults, includeConverted, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono filterBlobsAsync(Integer timeout, String requestId, String where, String marker,
Integer maxresults, List include) {
return filterBlobsWithResponseAsync(timeout, requestId, where, marker, maxresults, include)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono filterBlobsAsync(Integer timeout, String requestId, String where, String marker,
Integer maxresults, List include, Context context) {
return filterBlobsWithResponseAsync(timeout, requestId, where, marker, maxresults, include, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> filterBlobsNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId, String where, String marker, Integer maxresults, List include) {
final String comp = "blobs";
final String accept = "application/xml";
String includeConverted = (include == null)
? null
: include.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return FluxUtil
.withContext(context -> service.filterBlobsNoCustomHeaders(this.client.getUrl(), comp, timeout,
this.client.getVersion(), requestId, where, marker, maxresults, includeConverted, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* The Filter Blobs operation enables callers to list blobs across all containers whose tags match a given search
* expression. Filter blobs searches across all containers within a storage account but can be scoped within the
* expression to a single container.
*
* @param timeout The timeout parameter is expressed in seconds. For more information, see <a
* href="https://docs.microsoft.com/en-us/rest/api/storageservices/fileservices/setting-timeouts-for-blob-service-operations">Setting
* Timeouts for Blob Service Operations.</a>.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param where Filters the results to return only to return only blobs whose tags match the specified expression.
* @param marker A string value that identifies the portion of the list of containers to be returned with the next
* listing operation. The operation returns the NextMarker value within the response body if the listing operation
* did not return all containers remaining to be listed with the current page. The NextMarker value can be used as
* the value for the marker parameter in a subsequent call to request the next page of list items. The marker value
* is opaque to the client.
* @param maxresults Specifies the maximum number of containers to return. If the request does not specify
* maxresults, or specifies a value greater than 5000, the server will return up to 5000 items. Note that if the
* listing operation crosses a partition boundary, then the service will return a continuation token for retrieving
* the remainder of the results. For this reason, it is possible that the service will return fewer results than
* specified by maxresults, or than the default of 5000.
* @param include Include this parameter to specify one or more datasets to include in the response.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the result of a Filter Blobs API call along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> filterBlobsNoCustomHeadersWithResponseAsync(Integer timeout,
String requestId, String where, String marker, Integer maxresults, List include,
Context context) {
final String comp = "blobs";
final String accept = "application/xml";
String includeConverted = (include == null)
? null
: include.stream()
.map(paramItemValue -> Objects.toString(paramItemValue, ""))
.collect(Collectors.joining(","));
return service
.filterBlobsNoCustomHeaders(this.client.getUrl(), comp, timeout, this.client.getVersion(), requestId, where,
marker, maxresults, includeConverted, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException);
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentNextSinglePageAsync(String nextLink,
String requestId) {
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.listBlobContainersSegmentNext(nextLink, this.client.getUrl(),
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), res.getDeserializedHeaders()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentNextSinglePageAsync(String nextLink,
String requestId, Context context) {
final String accept = "application/xml";
return service
.listBlobContainersSegmentNext(nextLink, this.client.getUrl(), this.client.getVersion(), requestId, accept,
context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), res.getDeserializedHeaders()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
listBlobContainersSegmentNextNoCustomHeadersSinglePageAsync(String nextLink, String requestId) {
final String accept = "application/xml";
return FluxUtil
.withContext(context -> service.listBlobContainersSegmentNextNoCustomHeaders(nextLink, this.client.getUrl(),
this.client.getVersion(), requestId, accept, context))
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestId Provides a client-generated, opaque value with a 1 KB character limit that is recorded in the
* analytics logs when storage analytics logging is enabled.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws BlobStorageExceptionInternal thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an enumeration of containers along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> listBlobContainersSegmentNextNoCustomHeadersSinglePageAsync(
String nextLink, String requestId, Context context) {
final String accept = "application/xml";
return service
.listBlobContainersSegmentNextNoCustomHeaders(nextLink, this.client.getUrl(), this.client.getVersion(),
requestId, accept, context)
.onErrorMap(BlobStorageExceptionInternal.class, ModelHelper::mapToBlobStorageException)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().getBlobContainerItems(), res.getValue().getNextMarker(), null));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy