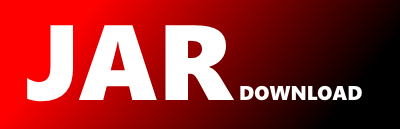
com.azure.storage.blob.implementation.models.BlobContainersSegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
This module contains client library for Microsoft Azure Blob Storage.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.storage.blob.models.BlobContainerItem;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
import java.util.ArrayList;
import java.util.List;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
/**
* An enumeration of containers.
*/
@Fluent
public final class BlobContainersSegment implements XmlSerializable {
/*
* The ServiceEndpoint property.
*/
private String serviceEndpoint;
/*
* The Prefix property.
*/
private String prefix;
/*
* The Marker property.
*/
private String marker;
/*
* The MaxResults property.
*/
private Integer maxResults;
/*
* The NextMarker property.
*/
private String nextMarker;
/*
* The BlobContainerItems property.
*/
private List blobContainerItems;
/**
* Creates an instance of BlobContainersSegment class.
*/
public BlobContainersSegment() {
}
/**
* Get the serviceEndpoint property: The ServiceEndpoint property.
*
* @return the serviceEndpoint value.
*/
public String getServiceEndpoint() {
return this.serviceEndpoint;
}
/**
* Set the serviceEndpoint property: The ServiceEndpoint property.
*
* @param serviceEndpoint the serviceEndpoint value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setServiceEndpoint(String serviceEndpoint) {
this.serviceEndpoint = serviceEndpoint;
return this;
}
/**
* Get the prefix property: The Prefix property.
*
* @return the prefix value.
*/
public String getPrefix() {
return this.prefix;
}
/**
* Set the prefix property: The Prefix property.
*
* @param prefix the prefix value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setPrefix(String prefix) {
this.prefix = prefix;
return this;
}
/**
* Get the marker property: The Marker property.
*
* @return the marker value.
*/
public String getMarker() {
return this.marker;
}
/**
* Set the marker property: The Marker property.
*
* @param marker the marker value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setMarker(String marker) {
this.marker = marker;
return this;
}
/**
* Get the maxResults property: The MaxResults property.
*
* @return the maxResults value.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
* Set the maxResults property: The MaxResults property.
*
* @param maxResults the maxResults value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Get the nextMarker property: The NextMarker property.
*
* @return the nextMarker value.
*/
public String getNextMarker() {
return this.nextMarker;
}
/**
* Set the nextMarker property: The NextMarker property.
*
* @param nextMarker the nextMarker value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setNextMarker(String nextMarker) {
this.nextMarker = nextMarker;
return this;
}
/**
* Get the blobContainerItems property: The BlobContainerItems property.
*
* @return the blobContainerItems value.
*/
public List getBlobContainerItems() {
if (this.blobContainerItems == null) {
this.blobContainerItems = new ArrayList<>();
}
return this.blobContainerItems;
}
/**
* Set the blobContainerItems property: The BlobContainerItems property.
*
* @param blobContainerItems the blobContainerItems value to set.
* @return the BlobContainersSegment object itself.
*/
public BlobContainersSegment setBlobContainerItems(List blobContainerItems) {
this.blobContainerItems = blobContainerItems;
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "EnumerationResults" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeStringAttribute("ServiceEndpoint", this.serviceEndpoint);
xmlWriter.writeStringElement("Prefix", this.prefix);
xmlWriter.writeStringElement("Marker", this.marker);
xmlWriter.writeNumberElement("MaxResults", this.maxResults);
xmlWriter.writeStringElement("NextMarker", this.nextMarker);
if (this.blobContainerItems != null) {
xmlWriter.writeStartElement("Containers");
for (BlobContainerItem element : this.blobContainerItems) {
xmlWriter.writeXml(element, "Container");
}
xmlWriter.writeEndElement();
}
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of BlobContainersSegment from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of BlobContainersSegment if the XmlReader was pointing to an instance of it, or null if it
* was pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobContainersSegment.
*/
public static BlobContainersSegment fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of BlobContainersSegment from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of BlobContainersSegment if the XmlReader was pointing to an instance of it, or null if it
* was pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobContainersSegment.
*/
public static BlobContainersSegment fromXml(XmlReader xmlReader, String rootElementName) throws XMLStreamException {
String finalRootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "EnumerationResults" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
BlobContainersSegment deserializedBlobContainersSegment = new BlobContainersSegment();
deserializedBlobContainersSegment.serviceEndpoint = reader.getStringAttribute(null, "ServiceEndpoint");
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Prefix".equals(elementName.getLocalPart())) {
deserializedBlobContainersSegment.prefix = reader.getStringElement();
} else if ("Marker".equals(elementName.getLocalPart())) {
deserializedBlobContainersSegment.marker = reader.getStringElement();
} else if ("MaxResults".equals(elementName.getLocalPart())) {
deserializedBlobContainersSegment.maxResults = reader.getNullableElement(Integer::parseInt);
} else if ("NextMarker".equals(elementName.getLocalPart())) {
deserializedBlobContainersSegment.nextMarker = reader.getStringElement();
} else if ("Containers".equals(elementName.getLocalPart())) {
while (reader.nextElement() != XmlToken.END_ELEMENT) {
elementName = reader.getElementName();
if ("Container".equals(elementName.getLocalPart())) {
if (deserializedBlobContainersSegment.blobContainerItems == null) {
deserializedBlobContainersSegment.blobContainerItems = new ArrayList<>();
}
deserializedBlobContainersSegment.blobContainerItems
.add(BlobContainerItem.fromXml(reader, "Container"));
} else {
reader.skipElement();
}
}
} else {
reader.skipElement();
}
}
return deserializedBlobContainersSegment;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy