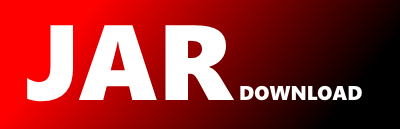
com.azure.storage.blob.models.BlobContainerItemProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.models;
import java.time.OffsetDateTime;
import java.util.Objects;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.DateTimeRfc1123;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
/**
* Properties of a container.
*/
@Fluent
public final class BlobContainerItemProperties implements XmlSerializable {
/*
* The Last-Modified property.
*/
private DateTimeRfc1123 lastModified;
/*
* The Etag property.
*/
private String eTag;
/*
* The LeaseStatus property.
*/
private LeaseStatusType leaseStatus;
/*
* The LeaseState property.
*/
private LeaseStateType leaseState;
/*
* The LeaseDuration property.
*/
private LeaseDurationType leaseDuration;
/*
* The PublicAccess property.
*/
private PublicAccessType publicAccess;
/*
* The HasImmutabilityPolicy property.
*/
private Boolean hasImmutabilityPolicy;
/*
* The HasLegalHold property.
*/
private Boolean hasLegalHold;
/*
* The DefaultEncryptionScope property.
*/
private String defaultEncryptionScope;
/*
* The DenyEncryptionScopeOverride property.
*/
private Boolean encryptionScopeOverridePrevented;
/*
* The DeletedTime property.
*/
private DateTimeRfc1123 deletedTime;
/*
* The RemainingRetentionDays property.
*/
private Integer remainingRetentionDays;
/*
* Indicates if version level worm is enabled on this container.
*/
private Boolean isImmutableStorageWithVersioningEnabled;
/**
* Creates an instance of BlobContainerItemProperties class.
*/
public BlobContainerItemProperties() {
}
/**
* Get the lastModified property: The Last-Modified property.
*
* @return the lastModified value.
*/
public OffsetDateTime getLastModified() {
if (this.lastModified == null) {
return null;
}
return this.lastModified.getDateTime();
}
/**
* Set the lastModified property: The Last-Modified property.
*
* @param lastModified the lastModified value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setLastModified(OffsetDateTime lastModified) {
if (lastModified == null) {
this.lastModified = null;
} else {
this.lastModified = new DateTimeRfc1123(lastModified);
}
return this;
}
/**
* Get the eTag property: The Etag property.
*
* @return the eTag value.
*/
public String getETag() {
return this.eTag;
}
/**
* Set the eTag property: The Etag property.
*
* @param eTag the eTag value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setETag(String eTag) {
this.eTag = eTag;
return this;
}
/**
* Get the leaseStatus property: The LeaseStatus property.
*
* @return the leaseStatus value.
*/
public LeaseStatusType getLeaseStatus() {
return this.leaseStatus;
}
/**
* Set the leaseStatus property: The LeaseStatus property.
*
* @param leaseStatus the leaseStatus value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setLeaseStatus(LeaseStatusType leaseStatus) {
this.leaseStatus = leaseStatus;
return this;
}
/**
* Get the leaseState property: The LeaseState property.
*
* @return the leaseState value.
*/
public LeaseStateType getLeaseState() {
return this.leaseState;
}
/**
* Set the leaseState property: The LeaseState property.
*
* @param leaseState the leaseState value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setLeaseState(LeaseStateType leaseState) {
this.leaseState = leaseState;
return this;
}
/**
* Get the leaseDuration property: The LeaseDuration property.
*
* @return the leaseDuration value.
*/
public LeaseDurationType getLeaseDuration() {
return this.leaseDuration;
}
/**
* Set the leaseDuration property: The LeaseDuration property.
*
* @param leaseDuration the leaseDuration value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setLeaseDuration(LeaseDurationType leaseDuration) {
this.leaseDuration = leaseDuration;
return this;
}
/**
* Get the publicAccess property: The PublicAccess property.
*
* @return the publicAccess value.
*/
public PublicAccessType getPublicAccess() {
return this.publicAccess;
}
/**
* Set the publicAccess property: The PublicAccess property.
*
* @param publicAccess the publicAccess value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setPublicAccess(PublicAccessType publicAccess) {
this.publicAccess = publicAccess;
return this;
}
/**
* Get the hasImmutabilityPolicy property: The HasImmutabilityPolicy property.
*
* @return the hasImmutabilityPolicy value.
*/
public Boolean isHasImmutabilityPolicy() {
return this.hasImmutabilityPolicy;
}
/**
* Set the hasImmutabilityPolicy property: The HasImmutabilityPolicy property.
*
* @param hasImmutabilityPolicy the hasImmutabilityPolicy value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setHasImmutabilityPolicy(Boolean hasImmutabilityPolicy) {
this.hasImmutabilityPolicy = hasImmutabilityPolicy;
return this;
}
/**
* Get the hasLegalHold property: The HasLegalHold property.
*
* @return the hasLegalHold value.
*/
public Boolean isHasLegalHold() {
return this.hasLegalHold;
}
/**
* Set the hasLegalHold property: The HasLegalHold property.
*
* @param hasLegalHold the hasLegalHold value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setHasLegalHold(Boolean hasLegalHold) {
this.hasLegalHold = hasLegalHold;
return this;
}
/**
* Get the defaultEncryptionScope property: The DefaultEncryptionScope property.
*
* @return the defaultEncryptionScope value.
*/
public String getDefaultEncryptionScope() {
return this.defaultEncryptionScope;
}
/**
* Set the defaultEncryptionScope property: The DefaultEncryptionScope property.
*
* @param defaultEncryptionScope the defaultEncryptionScope value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setDefaultEncryptionScope(String defaultEncryptionScope) {
this.defaultEncryptionScope = defaultEncryptionScope;
return this;
}
/**
* Get the encryptionScopeOverridePrevented property: The DenyEncryptionScopeOverride property.
*
* @return the encryptionScopeOverridePrevented value.
*/
public boolean isEncryptionScopeOverridePrevented() {
Boolean returnValue = this.encryptionScopeOverridePrevented;
return Boolean.TRUE.equals(returnValue);
}
/**
* Set the encryptionScopeOverridePrevented property: The DenyEncryptionScopeOverride property.
*
* @param encryptionScopeOverridePrevented the encryptionScopeOverridePrevented value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setEncryptionScopeOverridePrevented(boolean encryptionScopeOverridePrevented) {
this.encryptionScopeOverridePrevented = encryptionScopeOverridePrevented;
return this;
}
/**
* Get the deletedTime property: The DeletedTime property.
*
* @return the deletedTime value.
*/
public OffsetDateTime getDeletedTime() {
if (this.deletedTime == null) {
return null;
}
return this.deletedTime.getDateTime();
}
/**
* Set the deletedTime property: The DeletedTime property.
*
* @param deletedTime the deletedTime value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setDeletedTime(OffsetDateTime deletedTime) {
if (deletedTime == null) {
this.deletedTime = null;
} else {
this.deletedTime = new DateTimeRfc1123(deletedTime);
}
return this;
}
/**
* Get the remainingRetentionDays property: The RemainingRetentionDays property.
*
* @return the remainingRetentionDays value.
*/
public Integer getRemainingRetentionDays() {
return this.remainingRetentionDays;
}
/**
* Set the remainingRetentionDays property: The RemainingRetentionDays property.
*
* @param remainingRetentionDays the remainingRetentionDays value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties setRemainingRetentionDays(Integer remainingRetentionDays) {
this.remainingRetentionDays = remainingRetentionDays;
return this;
}
/**
* Get the isImmutableStorageWithVersioningEnabled property: Indicates if version level worm is enabled on this
* container.
*
* @return the isImmutableStorageWithVersioningEnabled value.
*/
public Boolean isImmutableStorageWithVersioningEnabled() {
return this.isImmutableStorageWithVersioningEnabled;
}
/**
* Set the isImmutableStorageWithVersioningEnabled property: Indicates if version level worm is enabled on this
* container.
*
* @param isImmutableStorageWithVersioningEnabled the isImmutableStorageWithVersioningEnabled value to set.
* @return the BlobContainerItemProperties object itself.
*/
public BlobContainerItemProperties
setImmutableStorageWithVersioningEnabled(Boolean isImmutableStorageWithVersioningEnabled) {
this.isImmutableStorageWithVersioningEnabled = isImmutableStorageWithVersioningEnabled;
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "BlobContainerItemProperties" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeStringElement("Last-Modified", Objects.toString(this.lastModified, null));
xmlWriter.writeStringElement("Etag", this.eTag);
xmlWriter.writeStringElement("LeaseStatus", this.leaseStatus == null ? null : this.leaseStatus.toString());
xmlWriter.writeStringElement("LeaseState", this.leaseState == null ? null : this.leaseState.toString());
xmlWriter.writeStringElement("LeaseDuration",
this.leaseDuration == null ? null : this.leaseDuration.toString());
xmlWriter.writeStringElement("PublicAccess", this.publicAccess == null ? null : this.publicAccess.toString());
xmlWriter.writeBooleanElement("HasImmutabilityPolicy", this.hasImmutabilityPolicy);
xmlWriter.writeBooleanElement("HasLegalHold", this.hasLegalHold);
xmlWriter.writeStringElement("DefaultEncryptionScope", this.defaultEncryptionScope);
xmlWriter.writeBooleanElement("DenyEncryptionScopeOverride", this.encryptionScopeOverridePrevented);
xmlWriter.writeStringElement("DeletedTime", Objects.toString(this.deletedTime, null));
xmlWriter.writeNumberElement("RemainingRetentionDays", this.remainingRetentionDays);
xmlWriter.writeBooleanElement("ImmutableStorageWithVersioningEnabled",
this.isImmutableStorageWithVersioningEnabled);
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of BlobContainerItemProperties from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of BlobContainerItemProperties if the XmlReader was pointing to an instance of it, or null if
* it was pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobContainerItemProperties.
*/
public static BlobContainerItemProperties fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of BlobContainerItemProperties from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of BlobContainerItemProperties if the XmlReader was pointing to an instance of it, or null if
* it was pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobContainerItemProperties.
*/
public static BlobContainerItemProperties fromXml(XmlReader xmlReader, String rootElementName)
throws XMLStreamException {
String finalRootElementName
= CoreUtils.isNullOrEmpty(rootElementName) ? "BlobContainerItemProperties" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
BlobContainerItemProperties deserializedBlobContainerItemProperties = new BlobContainerItemProperties();
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Last-Modified".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.lastModified
= reader.getNullableElement(DateTimeRfc1123::new);
} else if ("Etag".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.eTag = reader.getStringElement();
} else if ("LeaseStatus".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.leaseStatus
= LeaseStatusType.fromString(reader.getStringElement());
} else if ("LeaseState".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.leaseState
= LeaseStateType.fromString(reader.getStringElement());
} else if ("LeaseDuration".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.leaseDuration
= LeaseDurationType.fromString(reader.getStringElement());
} else if ("PublicAccess".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.publicAccess
= PublicAccessType.fromString(reader.getStringElement());
} else if ("HasImmutabilityPolicy".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.hasImmutabilityPolicy
= reader.getNullableElement(Boolean::parseBoolean);
} else if ("HasLegalHold".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.hasLegalHold
= reader.getNullableElement(Boolean::parseBoolean);
} else if ("DefaultEncryptionScope".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.defaultEncryptionScope = reader.getStringElement();
} else if ("DenyEncryptionScopeOverride".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.encryptionScopeOverridePrevented
= reader.getNullableElement(Boolean::parseBoolean);
} else if ("DeletedTime".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.deletedTime
= reader.getNullableElement(DateTimeRfc1123::new);
} else if ("RemainingRetentionDays".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.remainingRetentionDays
= reader.getNullableElement(Integer::parseInt);
} else if ("ImmutableStorageWithVersioningEnabled".equals(elementName.getLocalPart())) {
deserializedBlobContainerItemProperties.isImmutableStorageWithVersioningEnabled
= reader.getNullableElement(Boolean::parseBoolean);
} else {
reader.skipElement();
}
}
return deserializedBlobContainerItemProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy