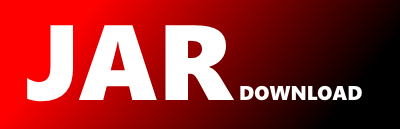
com.azure.storage.blob.models.BlobRetentionPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
This module contains client library for Microsoft Azure Blob Storage.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
/**
* the retention policy which determines how long the associated data should persist.
*/
@Fluent
public final class BlobRetentionPolicy implements XmlSerializable {
/*
* Indicates whether a retention policy is enabled for the storage service
*/
private boolean enabled;
/*
* Indicates the number of days that metrics or logging or soft-deleted data should be retained. All data older than this value will be deleted
*/
private Integer days;
/**
* Creates an instance of BlobRetentionPolicy class.
*/
public BlobRetentionPolicy() {
}
/**
* Get the enabled property: Indicates whether a retention policy is enabled for the storage service.
*
* @return the enabled value.
*/
public boolean isEnabled() {
return this.enabled;
}
/**
* Set the enabled property: Indicates whether a retention policy is enabled for the storage service.
*
* @param enabled the enabled value to set.
* @return the BlobRetentionPolicy object itself.
*/
public BlobRetentionPolicy setEnabled(boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get the days property: Indicates the number of days that metrics or logging or soft-deleted data should be
* retained. All data older than this value will be deleted.
*
* @return the days value.
*/
public Integer getDays() {
return this.days;
}
/**
* Set the days property: Indicates the number of days that metrics or logging or soft-deleted data should be
* retained. All data older than this value will be deleted.
*
* @param days the days value to set.
* @return the BlobRetentionPolicy object itself.
*/
public BlobRetentionPolicy setDays(Integer days) {
this.days = days;
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "BlobRetentionPolicy" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeBooleanElement("Enabled", this.enabled);
xmlWriter.writeNumberElement("Days", this.days);
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of BlobRetentionPolicy from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of BlobRetentionPolicy if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobRetentionPolicy.
*/
public static BlobRetentionPolicy fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of BlobRetentionPolicy from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of BlobRetentionPolicy if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the BlobRetentionPolicy.
*/
public static BlobRetentionPolicy fromXml(XmlReader xmlReader, String rootElementName) throws XMLStreamException {
String finalRootElementName
= CoreUtils.isNullOrEmpty(rootElementName) ? "BlobRetentionPolicy" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
BlobRetentionPolicy deserializedBlobRetentionPolicy = new BlobRetentionPolicy();
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Enabled".equals(elementName.getLocalPart())) {
deserializedBlobRetentionPolicy.enabled = reader.getBooleanElement();
} else if ("Days".equals(elementName.getLocalPart())) {
deserializedBlobRetentionPolicy.days = reader.getNullableElement(Integer::parseInt);
} else {
reader.skipElement();
}
}
return deserializedBlobRetentionPolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy