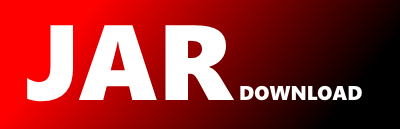
com.azure.storage.blob.models.BlobServiceProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
This module contains client library for Microsoft Azure Blob Storage.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
import java.util.ArrayList;
import java.util.List;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
/**
* Storage Service Properties.
*/
@Fluent
public final class BlobServiceProperties implements XmlSerializable {
/*
* Azure Analytics Logging settings.
*/
private BlobAnalyticsLogging logging;
/*
* a summary of request statistics grouped by API in hour or minute aggregates for blobs
*/
private BlobMetrics hourMetrics;
/*
* a summary of request statistics grouped by API in hour or minute aggregates for blobs
*/
private BlobMetrics minuteMetrics;
/*
* The set of CORS rules.
*/
private List cors;
/*
* The default version to use for requests to the Blob service if an incoming request's version is not specified. Possible values include version 2008-10-27 and all more recent versions
*/
private String defaultServiceVersion;
/*
* the retention policy which determines how long the associated data should persist
*/
private BlobRetentionPolicy deleteRetentionPolicy;
/*
* The properties that enable an account to host a static website
*/
private StaticWebsite staticWebsite;
/**
* Creates an instance of BlobServiceProperties class.
*/
public BlobServiceProperties() {
}
/**
* Get the logging property: Azure Analytics Logging settings.
*
* @return the logging value.
*/
public BlobAnalyticsLogging getLogging() {
return this.logging;
}
/**
* Set the logging property: Azure Analytics Logging settings.
*
* @param logging the logging value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setLogging(BlobAnalyticsLogging logging) {
this.logging = logging;
return this;
}
/**
* Get the hourMetrics property: a summary of request statistics grouped by API in hour or minute aggregates for
* blobs.
*
* @return the hourMetrics value.
*/
public BlobMetrics getHourMetrics() {
return this.hourMetrics;
}
/**
* Set the hourMetrics property: a summary of request statistics grouped by API in hour or minute aggregates for
* blobs.
*
* @param hourMetrics the hourMetrics value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setHourMetrics(BlobMetrics hourMetrics) {
this.hourMetrics = hourMetrics;
return this;
}
/**
* Get the minuteMetrics property: a summary of request statistics grouped by API in hour or minute aggregates for
* blobs.
*
* @return the minuteMetrics value.
*/
public BlobMetrics getMinuteMetrics() {
return this.minuteMetrics;
}
/**
* Set the minuteMetrics property: a summary of request statistics grouped by API in hour or minute aggregates for
* blobs.
*
* @param minuteMetrics the minuteMetrics value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setMinuteMetrics(BlobMetrics minuteMetrics) {
this.minuteMetrics = minuteMetrics;
return this;
}
/**
* Get the cors property: The set of CORS rules.
*
* @return the cors value.
*/
public List getCors() {
if (this.cors == null) {
this.cors = new ArrayList<>();
}
return this.cors;
}
/**
* Set the cors property: The set of CORS rules.
*
* @param cors the cors value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setCors(List cors) {
this.cors = cors;
return this;
}
/**
* Get the defaultServiceVersion property: The default version to use for requests to the Blob service if an
* incoming request's version is not specified. Possible values include version 2008-10-27 and all more recent
* versions.
*
* @return the defaultServiceVersion value.
*/
public String getDefaultServiceVersion() {
return this.defaultServiceVersion;
}
/**
* Set the defaultServiceVersion property: The default version to use for requests to the Blob service if an
* incoming request's version is not specified. Possible values include version 2008-10-27 and all more recent
* versions.
*
* @param defaultServiceVersion the defaultServiceVersion value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setDefaultServiceVersion(String defaultServiceVersion) {
this.defaultServiceVersion = defaultServiceVersion;
return this;
}
/**
* Get the deleteRetentionPolicy property: the retention policy which determines how long the associated data should
* persist.
*
* @return the deleteRetentionPolicy value.
*/
public BlobRetentionPolicy getDeleteRetentionPolicy() {
return this.deleteRetentionPolicy;
}
/**
* Set the deleteRetentionPolicy property: the retention policy which determines how long the associated data should
* persist.
*
* @param deleteRetentionPolicy the deleteRetentionPolicy value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setDeleteRetentionPolicy(BlobRetentionPolicy deleteRetentionPolicy) {
this.deleteRetentionPolicy = deleteRetentionPolicy;
return this;
}
/**
* Get the staticWebsite property: The properties that enable an account to host a static website.
*
* @return the staticWebsite value.
*/
public StaticWebsite getStaticWebsite() {
return this.staticWebsite;
}
/**
* Set the staticWebsite property: The properties that enable an account to host a static website.
*
* @param staticWebsite the staticWebsite value to set.
* @return the BlobServiceProperties object itself.
*/
public BlobServiceProperties setStaticWebsite(StaticWebsite staticWebsite) {
this.staticWebsite = staticWebsite;
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "StorageServiceProperties" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeXml(this.logging, "Logging");
xmlWriter.writeXml(this.hourMetrics, "HourMetrics");
xmlWriter.writeXml(this.minuteMetrics, "MinuteMetrics");
if (this.cors != null) {
xmlWriter.writeStartElement("Cors");
for (BlobCorsRule element : this.cors) {
xmlWriter.writeXml(element, "CorsRule");
}
xmlWriter.writeEndElement();
}
xmlWriter.writeStringElement("DefaultServiceVersion", this.defaultServiceVersion);
xmlWriter.writeXml(this.deleteRetentionPolicy, "DeleteRetentionPolicy");
xmlWriter.writeXml(this.staticWebsite, "StaticWebsite");
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of BlobServiceProperties from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of BlobServiceProperties if the XmlReader was pointing to an instance of it, or null if it
* was pointing to XML null.
* @throws XMLStreamException If an error occurs while reading the BlobServiceProperties.
*/
public static BlobServiceProperties fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of BlobServiceProperties from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of BlobServiceProperties if the XmlReader was pointing to an instance of it, or null if it
* was pointing to XML null.
* @throws XMLStreamException If an error occurs while reading the BlobServiceProperties.
*/
public static BlobServiceProperties fromXml(XmlReader xmlReader, String rootElementName) throws XMLStreamException {
String finalRootElementName
= CoreUtils.isNullOrEmpty(rootElementName) ? "StorageServiceProperties" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
BlobServiceProperties deserializedBlobServiceProperties = new BlobServiceProperties();
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Logging".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.logging = BlobAnalyticsLogging.fromXml(reader, "Logging");
} else if ("HourMetrics".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.hourMetrics = BlobMetrics.fromXml(reader, "HourMetrics");
} else if ("MinuteMetrics".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.minuteMetrics = BlobMetrics.fromXml(reader, "MinuteMetrics");
} else if ("Cors".equals(elementName.getLocalPart())) {
while (reader.nextElement() != XmlToken.END_ELEMENT) {
elementName = reader.getElementName();
if ("CorsRule".equals(elementName.getLocalPart())) {
if (deserializedBlobServiceProperties.cors == null) {
deserializedBlobServiceProperties.cors = new ArrayList<>();
}
deserializedBlobServiceProperties.cors.add(BlobCorsRule.fromXml(reader, "CorsRule"));
} else {
reader.skipElement();
}
}
} else if ("DefaultServiceVersion".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.defaultServiceVersion = reader.getStringElement();
} else if ("DeleteRetentionPolicy".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.deleteRetentionPolicy
= BlobRetentionPolicy.fromXml(reader, "DeleteRetentionPolicy");
} else if ("StaticWebsite".equals(elementName.getLocalPart())) {
deserializedBlobServiceProperties.staticWebsite = StaticWebsite.fromXml(reader, "StaticWebsite");
} else {
reader.skipElement();
}
}
return deserializedBlobServiceProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy