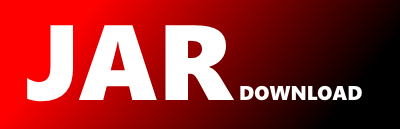
com.azure.storage.blob.models.GeoReplication Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-storage-blob Show documentation
Show all versions of azure-storage-blob Show documentation
This module contains client library for Microsoft Azure Blob Storage.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.DateTimeRfc1123;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
import java.time.OffsetDateTime;
import java.util.Objects;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
/**
* Geo-Replication information for the Secondary Storage Service.
*/
@Fluent
public final class GeoReplication implements XmlSerializable {
/*
* The status of the secondary location
*/
private GeoReplicationStatus status;
/*
* A GMT date/time value, to the second. All primary writes preceding this value are guaranteed to be available for read operations at the secondary. Primary writes after this point in time may or may not be available for reads.
*/
private DateTimeRfc1123 lastSyncTime;
/**
* Creates an instance of GeoReplication class.
*/
public GeoReplication() {
}
/**
* Get the status property: The status of the secondary location.
*
* @return the status value.
*/
public GeoReplicationStatus getStatus() {
return this.status;
}
/**
* Set the status property: The status of the secondary location.
*
* @param status the status value to set.
* @return the GeoReplication object itself.
*/
public GeoReplication setStatus(GeoReplicationStatus status) {
this.status = status;
return this;
}
/**
* Get the lastSyncTime property: A GMT date/time value, to the second. All primary writes preceding this value are
* guaranteed to be available for read operations at the secondary. Primary writes after this point in time may or
* may not be available for reads.
*
* @return the lastSyncTime value.
*/
public OffsetDateTime getLastSyncTime() {
if (this.lastSyncTime == null) {
return null;
}
return this.lastSyncTime.getDateTime();
}
/**
* Set the lastSyncTime property: A GMT date/time value, to the second. All primary writes preceding this value are
* guaranteed to be available for read operations at the secondary. Primary writes after this point in time may or
* may not be available for reads.
*
* @param lastSyncTime the lastSyncTime value to set.
* @return the GeoReplication object itself.
*/
public GeoReplication setLastSyncTime(OffsetDateTime lastSyncTime) {
if (lastSyncTime == null) {
this.lastSyncTime = null;
} else {
this.lastSyncTime = new DateTimeRfc1123(lastSyncTime);
}
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "GeoReplication" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeStringElement("Status", this.status == null ? null : this.status.toString());
xmlWriter.writeStringElement("LastSyncTime", Objects.toString(this.lastSyncTime, null));
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of GeoReplication from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of GeoReplication if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the GeoReplication.
*/
public static GeoReplication fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of GeoReplication from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of GeoReplication if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the GeoReplication.
*/
public static GeoReplication fromXml(XmlReader xmlReader, String rootElementName) throws XMLStreamException {
String finalRootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "GeoReplication" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
GeoReplication deserializedGeoReplication = new GeoReplication();
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Status".equals(elementName.getLocalPart())) {
deserializedGeoReplication.status = GeoReplicationStatus.fromString(reader.getStringElement());
} else if ("LastSyncTime".equals(elementName.getLocalPart())) {
deserializedGeoReplication.lastSyncTime = reader.getNullableElement(DateTimeRfc1123::new);
} else {
reader.skipElement();
}
}
return deserializedGeoReplication;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy