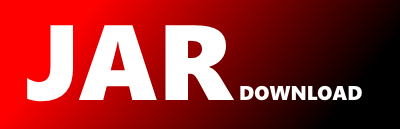
com.azure.storage.blob.models.StaticWebsite Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.storage.blob.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.xml.XmlReader;
import com.azure.xml.XmlSerializable;
import com.azure.xml.XmlToken;
import com.azure.xml.XmlWriter;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLStreamException;
/**
* The properties that enable an account to host a static website.
*/
@Fluent
public final class StaticWebsite implements XmlSerializable {
/*
* Indicates whether this account is hosting a static website
*/
private boolean enabled;
/*
* The default name of the index page under each directory
*/
private String indexDocument;
/*
* The absolute path of the custom 404 page
*/
private String errorDocument404Path;
/*
* Absolute path of the default index page
*/
private String defaultIndexDocumentPath;
/**
* Creates an instance of StaticWebsite class.
*/
public StaticWebsite() {
}
/**
* Get the enabled property: Indicates whether this account is hosting a static website.
*
* @return the enabled value.
*/
public boolean isEnabled() {
return this.enabled;
}
/**
* Set the enabled property: Indicates whether this account is hosting a static website.
*
* @param enabled the enabled value to set.
* @return the StaticWebsite object itself.
*/
public StaticWebsite setEnabled(boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get the indexDocument property: The default name of the index page under each directory.
*
* @return the indexDocument value.
*/
public String getIndexDocument() {
return this.indexDocument;
}
/**
* Set the indexDocument property: The default name of the index page under each directory.
*
* @param indexDocument the indexDocument value to set.
* @return the StaticWebsite object itself.
*/
public StaticWebsite setIndexDocument(String indexDocument) {
this.indexDocument = indexDocument;
return this;
}
/**
* Get the errorDocument404Path property: The absolute path of the custom 404 page.
*
* @return the errorDocument404Path value.
*/
public String getErrorDocument404Path() {
return this.errorDocument404Path;
}
/**
* Set the errorDocument404Path property: The absolute path of the custom 404 page.
*
* @param errorDocument404Path the errorDocument404Path value to set.
* @return the StaticWebsite object itself.
*/
public StaticWebsite setErrorDocument404Path(String errorDocument404Path) {
this.errorDocument404Path = errorDocument404Path;
return this;
}
/**
* Get the defaultIndexDocumentPath property: Absolute path of the default index page.
*
* @return the defaultIndexDocumentPath value.
*/
public String getDefaultIndexDocumentPath() {
return this.defaultIndexDocumentPath;
}
/**
* Set the defaultIndexDocumentPath property: Absolute path of the default index page.
*
* @param defaultIndexDocumentPath the defaultIndexDocumentPath value to set.
* @return the StaticWebsite object itself.
*/
public StaticWebsite setDefaultIndexDocumentPath(String defaultIndexDocumentPath) {
this.defaultIndexDocumentPath = defaultIndexDocumentPath;
return this;
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter) throws XMLStreamException {
return toXml(xmlWriter, null);
}
@Override
public XmlWriter toXml(XmlWriter xmlWriter, String rootElementName) throws XMLStreamException {
rootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "StaticWebsite" : rootElementName;
xmlWriter.writeStartElement(rootElementName);
xmlWriter.writeBooleanElement("Enabled", this.enabled);
xmlWriter.writeStringElement("IndexDocument", this.indexDocument);
xmlWriter.writeStringElement("ErrorDocument404Path", this.errorDocument404Path);
xmlWriter.writeStringElement("DefaultIndexDocumentPath", this.defaultIndexDocumentPath);
return xmlWriter.writeEndElement();
}
/**
* Reads an instance of StaticWebsite from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @return An instance of StaticWebsite if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the StaticWebsite.
*/
public static StaticWebsite fromXml(XmlReader xmlReader) throws XMLStreamException {
return fromXml(xmlReader, null);
}
/**
* Reads an instance of StaticWebsite from the XmlReader.
*
* @param xmlReader The XmlReader being read.
* @param rootElementName Optional root element name to override the default defined by the model. Used to support
* cases where the model can deserialize from different root element names.
* @return An instance of StaticWebsite if the XmlReader was pointing to an instance of it, or null if it was
* pointing to XML null.
* @throws IllegalStateException If the deserialized XML object was missing any required properties.
* @throws XMLStreamException If an error occurs while reading the StaticWebsite.
*/
public static StaticWebsite fromXml(XmlReader xmlReader, String rootElementName) throws XMLStreamException {
String finalRootElementName = CoreUtils.isNullOrEmpty(rootElementName) ? "StaticWebsite" : rootElementName;
return xmlReader.readObject(finalRootElementName, reader -> {
StaticWebsite deserializedStaticWebsite = new StaticWebsite();
while (reader.nextElement() != XmlToken.END_ELEMENT) {
QName elementName = reader.getElementName();
if ("Enabled".equals(elementName.getLocalPart())) {
deserializedStaticWebsite.enabled = reader.getBooleanElement();
} else if ("IndexDocument".equals(elementName.getLocalPart())) {
deserializedStaticWebsite.indexDocument = reader.getStringElement();
} else if ("ErrorDocument404Path".equals(elementName.getLocalPart())) {
deserializedStaticWebsite.errorDocument404Path = reader.getStringElement();
} else if ("DefaultIndexDocumentPath".equals(elementName.getLocalPart())) {
deserializedStaticWebsite.defaultIndexDocumentPath = reader.getStringElement();
} else {
reader.skipElement();
}
}
return deserializedStaticWebsite;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy