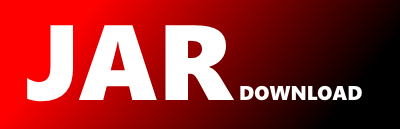
com.backblaze.b2.client.structures.B2CreateBucketRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of b2-sdk-core Show documentation
Show all versions of b2-sdk-core Show documentation
The core logic for B2 SDK for Java. Does not include any implementations of B2WebApiClient.
/*
* Copyright 2017, Backblaze Inc. All Rights Reserved.
* License https://www.backblaze.com/using_b2_code.html
*/
package com.backblaze.b2.client.structures;
import java.util.List;
import java.util.Map;
public class B2CreateBucketRequest {
private final String bucketName;
private final String bucketType;
private final Map bucketInfo;
private final List corsRules;
private final List lifecycleRules;
public B2CreateBucketRequest(String bucketName,
String bucketType,
Map bucketInfo,
List corsRules,
List lifecycleRules) {
this.bucketName = bucketName;
this.bucketType = bucketType;
this.bucketInfo = bucketInfo;
this.corsRules = corsRules;
this.lifecycleRules = lifecycleRules;
}
public String getBucketName() {
return bucketName;
}
public String getBucketType() {
return bucketType;
}
public Map getBucketInfo() {
return bucketInfo;
}
public List getCorsRules() {
return corsRules;
}
public List getLifecycleRules() {
return lifecycleRules;
}
public static Builder builder(String bucketName, String bucketType) {
return new Builder(bucketName, bucketType);
}
public static class Builder {
private final String bucketName;
private final String bucketType;
private Map bucketInfo;
private List corsRules;
private List lifecycleRules;
Builder(String bucketName,
String bucketType) {
this.bucketName = bucketName;
this.bucketType = bucketType;
}
public Builder setBucketInfo(Map bucketInfo) {
this.bucketInfo = bucketInfo;
return this;
}
public Builder setCorsRules(List corsRules){
this.corsRules = corsRules;
return this;
}
public Builder setLifecycleRules(List lifecycleRules) {
this.lifecycleRules = lifecycleRules;
return this;
}
public B2CreateBucketRequest build() {
return new B2CreateBucketRequest(bucketName, bucketType, bucketInfo, corsRules, lifecycleRules);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy