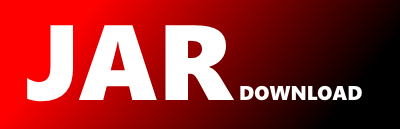
com.backblaze.b2.client.structures.B2CreateBucketRequestReal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of b2-sdk-core Show documentation
Show all versions of b2-sdk-core Show documentation
The core logic for B2 SDK for Java. Does not include any implementations of B2WebApiClient.
/*
* Copyright 2017, Backblaze Inc. All Rights Reserved.
* License https://www.backblaze.com/using_b2_code.html
*/
package com.backblaze.b2.client.structures;
import com.backblaze.b2.json.B2Json;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* NOTE:
* B2CreateBucketRequestReal has the attributes needed by the B2 API. That's why it's name ends with 'Real'.
* Code that calls B2StorageClient uses B2CreateBucketRequest (with no 'Real' at the end) instead.
* The B2StorageClient creates a 'Real' request by adding the accountId to the non-real version before
* sending it to the webifier.
*/
public class B2CreateBucketRequestReal {
@B2Json.required
private final String accountId;
@B2Json.required
private final String bucketName;
@B2Json.optional
private final String bucketType;
@B2Json.optional
private final Map bucketInfo;
@B2Json.optional
private final List corsRules;
@B2Json.optional
private final List lifecycleRules;
@B2Json.constructor(params = "accountId,bucketName,bucketType,bucketInfo,corsRules,lifecycleRules")
private B2CreateBucketRequestReal(String accountId,
String bucketName,
String bucketType,
Map bucketInfo,
List corsRules,
List lifecycleRules) {
this.accountId = accountId;
this.bucketName = bucketName;
this.bucketType = bucketType;
this.bucketInfo = bucketInfo;
this.corsRules = corsRules;
this.lifecycleRules = lifecycleRules;
}
public B2CreateBucketRequestReal(String accountId,
B2CreateBucketRequest mostOfRequest) {
this(accountId,
mostOfRequest.getBucketName(),
mostOfRequest.getBucketType(),
mostOfRequest.getBucketInfo(),
mostOfRequest.getCorsRules(),
mostOfRequest.getLifecycleRules());
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
B2CreateBucketRequestReal that = (B2CreateBucketRequestReal) o;
return Objects.equals(accountId, that.accountId) &&
Objects.equals(bucketName, that.bucketName) &&
Objects.equals(bucketType, that.bucketType) &&
Objects.equals(bucketInfo, that.bucketInfo) &&
Objects.equals(corsRules, that.corsRules) &&
Objects.equals(lifecycleRules, that.lifecycleRules);
}
@Override
public int hashCode() {
return Objects.hash(accountId, bucketName, bucketType, bucketInfo, corsRules, lifecycleRules);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy