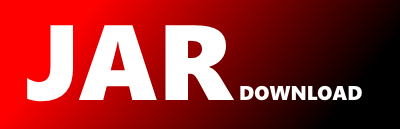
com.backblaze.b2.client.structures.B2CreatedApplicationKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of b2-sdk-core Show documentation
Show all versions of b2-sdk-core Show documentation
The core logic for B2 SDK for Java. Does not include any implementations of B2WebApiClient.
/*
* Copyright 2018, Backblaze Inc. All Rights Reserved.
* License https://www.backblaze.com/using_b2_code.html
*/
package com.backblaze.b2.client.structures;
import com.backblaze.b2.json.B2Json;
import java.util.Objects;
import java.util.TreeSet;
/**
* Information returned from b2_create_key.
*
* This is like B2ApplicationKey, with the addition of the secret applicationKey,
* which is returned when a key is created, but not returned by b2_list_keys,
* or b2_delete_key.
*/
public class B2CreatedApplicationKey {
@B2Json.required
private final String accountId;
@B2Json.required
private final String applicationKeyId;
@B2Json.required
private final String applicationKey;
@B2Json.required
private final String keyName;
@B2Json.required
private final TreeSet capabilities;
@B2Json.optional
private final String bucketId;
@B2Json.optional
private final String namePrefix;
@B2Json.optional
private final Long expirationTimestamp;
@SuppressWarnings("unused")
@B2Json.constructor(
params =
"accountId, " +
"applicationKeyId, " +
"applicationKey, " +
"keyName, " +
"capabilities, " +
"bucketId, " +
"namePrefix, " +
"expirationTimestamp"
)
public B2CreatedApplicationKey(String accountId,
String applicationKeyId,
String applicationKey,
String keyName,
TreeSet capabilities,
String bucketId,
String namePrefix,
Long expirationTimestamp) {
this.accountId = accountId;
this.applicationKeyId = applicationKeyId;
this.applicationKey = applicationKey;
this.keyName = keyName;
this.capabilities = capabilities;
this.bucketId = bucketId;
this.namePrefix = namePrefix;
this.expirationTimestamp = expirationTimestamp;
}
public String getAccountId() {
return accountId;
}
@SuppressWarnings("unused")
public String getApplicationKeyId() {
return applicationKeyId;
}
@SuppressWarnings("unused")
public String getApplicationKey() {
return applicationKey;
}
@SuppressWarnings("unused")
public String getKeyName() {
return keyName;
}
@SuppressWarnings("unused")
public TreeSet getCapabilities() {
return capabilities;
}
@SuppressWarnings("unused")
public String getBucketId() {
return bucketId;
}
@SuppressWarnings("unused")
public String getNamePrefix() {
return namePrefix;
}
@SuppressWarnings("unused")
public Long getExpirationTimestamp() {
return expirationTimestamp;
}
/**
* Converts to the B2ApplicationKey structure, as returned from b2_list_keys,
* which does not contain the secret key.
*/
public B2ApplicationKey toApplicationKey() {
return new B2ApplicationKey(
accountId,
applicationKeyId,
keyName,
capabilities,
bucketId,
namePrefix,
expirationTimestamp
);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
B2CreatedApplicationKey that = (B2CreatedApplicationKey) o;
return Objects.equals(accountId, that.accountId) &&
Objects.equals(applicationKeyId, that.applicationKeyId) &&
Objects.equals(applicationKey, that.applicationKey) &&
Objects.equals(keyName, that.keyName) &&
Objects.equals(capabilities, that.capabilities) &&
Objects.equals(bucketId, that.bucketId) &&
Objects.equals(namePrefix, that.namePrefix) &&
Objects.equals(expirationTimestamp, that.expirationTimestamp);
}
@Override
public int hashCode() {
return Objects.hash(accountId, applicationKeyId, applicationKey, keyName, capabilities, bucketId, namePrefix, expirationTimestamp);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy