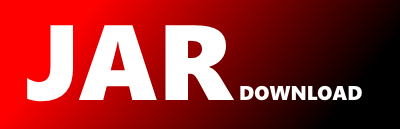
com.backblaze.b2.util.B2Collections Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of b2-sdk-core Show documentation
Show all versions of b2-sdk-core Show documentation
The core logic for B2 SDK for Java. Does not include any implementations of B2WebApiClient.
/*
* Copyright 2017, Backblaze Inc. All Rights Reserved.
* License https://www.backblaze.com/using_b2_code.html
*/
package com.backblaze.b2.util;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
public class B2Collections {
public static Set unmodifiableSet(T[] elements) {
final Set set = new HashSet<>(elements.length);
set.addAll(Arrays.asList(elements));
return Collections.unmodifiableSet(set);
}
public static Map unmodifiableMap(Map orig) {
final Map map = new TreeMap<>();
map.putAll(orig);
return Collections.unmodifiableMap(map);
}
public static Map mapOf() {
return new TreeMap<>();
}
public static Map mapOf(K k1, V v1) {
final Map map = new TreeMap<>();
map.put(k1, v1);
return map;
}
public static Map mapOf(K k1, V v1,
K k2, V v2) {
final Map map = new TreeMap<>();
map.put(k1, v1);
map.put(k2, v2);
return map;
}
public static Map mapOf(K k1, V v1,
K k2, V v2,
K k3, V v3) {
final Map map = new TreeMap<>();
map.put(k1, v1);
map.put(k2, v2);
map.put(k3, v3);
return map;
}
@SafeVarargs
public static List listOf(T... orig) {
return Arrays.asList(orig);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy