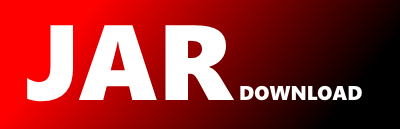
com.backendless.BackendlessUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
/*
* ********************************************************************************************************************
*
* BACKENDLESS.COM CONFIDENTIAL
*
* ********************************************************************************************************************
*
* Copyright 2012 BACKENDLESS.COM. All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Backendless.com and its suppliers,
* if any. The intellectual and technical concepts contained herein are proprietary to Backendless.com and its
* suppliers and may be covered by U.S. and Foreign Patents, patents in process, and are protected by trade secret
* or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden
* unless prior written permission is obtained from Backendless.com.
*
* ********************************************************************************************************************
*/
package com.backendless;
import java.io.Serializable;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class BackendlessUser implements Serializable
{
private final Map properties = new HashMap<>();
public static final String PASSWORD_KEY = "password";
public static final String EMAIL_KEY = "email";
public static final String ID_KEY = "objectId";
public static final String LOCALE = "blUserLocale";
public BackendlessUser()
{
properties.put( LOCALE, System.getProperty("user.language") );
}
/**
* Returns a COPY of user's properties
* (this means if you modify the return value, actual user properties won't be modified)
*
* @return a COPY of this user's properties
*/
public Map getProperties()
{
return new HashMap<>( properties );
}
public void setProperties( Map properties )
{
synchronized( this )
{
this.properties.clear();
putProperties( properties );
}
}
public void putProperties( Map properties )
{
synchronized( this )
{
this.properties.putAll( properties );
}
}
public Object getProperty( String key )
{
synchronized( this )
{
if( properties == null )
return null;
return properties.get( key );
}
}
public void setProperty( String key, Object value )
{
synchronized( this )
{
properties.put( key, value );
}
}
public String getObjectId()
{
return getUserId();
}
public String getUserId()
{
Object result = getProperty( ID_KEY );
return result == null ? null : String.valueOf( result );
}
public void setPassword( String password )
{
setProperty( PASSWORD_KEY, password );
}
public String getPassword()
{
Object result = getProperty( PASSWORD_KEY );
return result == null ? null : String.valueOf( result );
}
public void setEmail( String email )
{
setProperty( EMAIL_KEY, email );
}
public String getEmail()
{
Object result = getProperty( EMAIL_KEY );
return result == null ? null : String.valueOf( result );
}
public void clearProperties()
{
synchronized( this )
{
properties.clear();
}
}
public Object removeProperty( String key )
{
return properties.remove( key );
}
public boolean isEmpty()
{
// Second condition mean that only property that User has is default locale
return properties.isEmpty() || (properties.size() == 1 && properties.containsKey( LOCALE ));
}
@Override
public boolean equals( Object o )
{
if( this == o )
return true;
if( o == null || getClass() != o.getClass() )
return false;
BackendlessUser that = (BackendlessUser) o;
return properties.equals( that.properties );
}
private Object marker;// = new Object();
@Override
public int hashCode()
{
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy