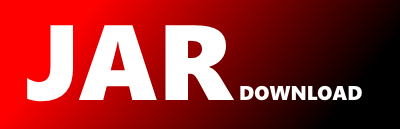
com.backendless.IDataStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
/*
* ********************************************************************************************************************
*
* BACKENDLESS.COM CONFIDENTIAL
*
* ********************************************************************************************************************
*
* Copyright 2012 BACKENDLESS.COM. All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Backendless.com and its suppliers,
* if any. The intellectual and technical concepts contained herein are proprietary to Backendless.com and its
* suppliers and may be covered by U.S. and Foreign Patents, patents in process, and are protected by trade secret
* or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden
* unless prior written permission is obtained from Backendless.com.
*
* ********************************************************************************************************************
*/
package com.backendless;
import com.backendless.async.callback.AsyncCallback;
import com.backendless.commons.persistence.GroupResult;
import com.backendless.exceptions.BackendlessException;
import com.backendless.persistence.DataQueryBuilder;
import com.backendless.persistence.GroupDataQueryBuilder;
import com.backendless.persistence.LoadRelationsQueryBuilder;
import com.backendless.rt.data.EventHandler;
import java.util.Collection;
import java.util.List;
import java.util.Map;
public interface IDataStore
{
List create( List objects ) throws BackendlessException;
void create( List objects, AsyncCallback> responder ) throws BackendlessException;
E save( E entity ) throws BackendlessException;
E save( E entity, boolean isUpsert ) throws BackendlessException;
void save( E entity, AsyncCallback responder );
void save( E entity, boolean isUpsert, AsyncCallback responder );
E deepSave( E entity ) throws BackendlessException;
void deepSave( E entity, AsyncCallback responder );
Long remove( E entity ) throws BackendlessException;
void remove( E entity, AsyncCallback responder );
int remove( String whereClause ) throws BackendlessException;
void remove( String whereClause, AsyncCallback responder ) throws BackendlessException;
int update( String whereClause, Map changes ) throws BackendlessException;
void update( String whereClause, Map changes, AsyncCallback responder ) throws BackendlessException;
E findFirst() throws BackendlessException;
E findFirst( Integer relationsDepth ) throws BackendlessException;
E findFirst( List relations ) throws BackendlessException;
E findFirst( List relations, Integer relationsDepth, Integer relationsPageSize ) throws BackendlessException;
void findFirst( AsyncCallback responder );
void findFirst( Integer relationsDepth, AsyncCallback responder );
void findFirst( List relations, AsyncCallback responder );
void findFirst( List relations, Integer relationsDepth, Integer relationsPageSize, final AsyncCallback responder );
E findLast() throws BackendlessException;
E findLast( Integer relationsDepth ) throws BackendlessException;
E findLast( List relations ) throws BackendlessException;
E findLast( List relations, Integer relationsDepth, Integer relationsPageSize ) throws BackendlessException;
void findLast( AsyncCallback responder );
void findLast( Integer relationsDepth, AsyncCallback responder );
void findLast( List relations, AsyncCallback responder );
void findLast( List relations, Integer relationsDepth, Integer relationsPageSize, final AsyncCallback responder );
List find() throws BackendlessException;
List find( DataQueryBuilder dataQueryBuilder ) throws BackendlessException;
void find( AsyncCallback> responder );
void find( DataQueryBuilder dataQueryBuilder, AsyncCallback> responder );
GroupResult,E> group( GroupDataQueryBuilder dataQueryBuilder );
void group( GroupDataQueryBuilder dataQueryBuilder, AsyncCallback> responder );
E findById( String id ) throws BackendlessException;
E findById( String id, List relations ) throws BackendlessException;
E findById( String id, Integer relationsDepth ) throws BackendlessException;
E findById( String id, List relations, Integer relationsDepth ) throws BackendlessException;
E findById( String id, DataQueryBuilder queryBuilder ) throws BackendlessException;
E findById( E entity ) throws BackendlessException;
E findById( E entity, List relations ) throws BackendlessException;
E findById( E entity, Integer relationsDepth ) throws BackendlessException;
E findById( E entity, List relations, Integer relationsDepth ) throws BackendlessException;
E findById( E entity, DataQueryBuilder queryBuilder ) throws BackendlessException;
int getObjectCount();
int getObjectCount( DataQueryBuilder dataQueryBuilder );
int getObjectCountInGroup( GroupDataQueryBuilder dataQueryBuilder );
void findById( String id, AsyncCallback responder );
void findById( String id, List relations, AsyncCallback responder );
void findById( String id, Integer relationsDepth, AsyncCallback responder );
void findById( String id, List relations, Integer relationsDepth, AsyncCallback responder );
void findById( String id, DataQueryBuilder queryBuilder, AsyncCallback responder );
void findById( E entity, AsyncCallback responder );
void findById( E entity, List relations, AsyncCallback responder );
void findById( E entity, Integer relationsDepth, AsyncCallback responder );
void findById( E entity, List relations, Integer relationsDepth, AsyncCallback responder );
void findById( E entity, DataQueryBuilder queryBuilder, AsyncCallback responder );
/**
* @see LoadRelationsQueryBuilder
*
* @param objectId parentObjectId
* @param child relation type
*/
List loadRelations( String objectId, LoadRelationsQueryBuilder queryBuilder );
/**
* @see LoadRelationsQueryBuilder
*
* @param objectId parentObjectId
* @param child relation type
* @param responder asynchronous callback
*/
void loadRelations( String objectId, LoadRelationsQueryBuilder queryBuilder, AsyncCallback> responder );
void getObjectCount( AsyncCallback responder );
void getObjectCount( DataQueryBuilder dataQueryBuilder, AsyncCallback responder );
void getObjectCountInGroup( GroupDataQueryBuilder dataQueryBuilder, AsyncCallback responder );
int addRelation( E parent, String relationColumnName, Collection children );
void addRelation( E parent, String relationColumnName, Collection children, AsyncCallback callback );
int addRelation( E parent, String relationColumnName, String whereClause );
void addRelation( E parent, String relationColumnName, String whereClause, AsyncCallback callback );
int setRelation( E parent, String relationColumnName, Collection children );
void setRelation( E parent, String relationColumnName, Collection children, AsyncCallback callback );
int setRelation( E parent, String relationColumnName, String whereClause );
void setRelation( E parent, String relationColumnName, String whereClause, AsyncCallback callback );
int deleteRelation( E parent, String relationColumnName, Collection children );
void deleteRelation( E parent, String relationColumnName, Collection children, AsyncCallback callback );
int deleteRelation( E parent, String relationColumnName, String whereClause );
void deleteRelation( E parent, String relationColumnName, String whereClause, AsyncCallback callback );
int addRelation( String parentObjectId, String relationColumnName, Collection childrenObjectIds );
void addRelation( String parentObjectId, String relationColumnName, Collection childrenObjectIds, AsyncCallback callback );
int addRelation( String parentObjectId, String relationColumnName, String whereClause );
void addRelation( String parentObjectId, String relationColumnName, String whereClause, AsyncCallback callback );
int setRelation( String parentObjectId, String relationColumnName, Collection childrenObjectIds );
void setRelation( String parentObjectId, String relationColumnName, Collection childrenObjectIds, AsyncCallback callback );
int setRelation( String parentObjectId, String relationColumnName, String whereClause );
void setRelation( String parentObjectId, String relationColumnName, String whereClause, AsyncCallback callback );
int deleteRelation( String parentObjectId, String relationColumnName, Collection childrenObjectIds );
void deleteRelation(String parentObjectId, String relationColumnName, Collection childrenObjectIds, AsyncCallback callback );
int deleteRelation( String parentObjectId, String relationColumnName, String whereClause );
void deleteRelation( String parentObjectId, String relationColumnName, String whereClause, AsyncCallback callback );
EventHandler rt();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy