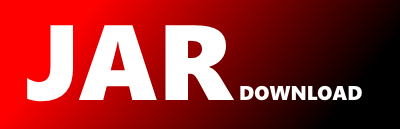
com.backendless.Messaging Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless;
import com.backendless.async.callback.AsyncCallback;
import com.backendless.exceptions.BackendlessException;
import com.backendless.messaging.BodyParts;
import com.backendless.messaging.DeliveryOptions;
import com.backendless.messaging.EmailEnvelope;
import com.backendless.messaging.Message;
import com.backendless.messaging.MessageStatus;
import com.backendless.messaging.PublishOptions;
import com.backendless.push.DeviceRegistrationResult;
import com.backendless.rt.messaging.Channel;
import java.util.Date;
import java.util.List;
import java.util.Map;
public interface Messaging
{
static String getDeviceId()
{
return GeneralMessaging.prefs.getDeviceId();
}
static String getOS()
{
return GeneralMessaging.prefs.getOs();
}
static String getOsVersion()
{
return GeneralMessaging.prefs.getOsVersion();
}
void registerDevice();
void registerDevice( AsyncCallback callback );
void registerDevice( List channels );
void registerDevice( List channels, Date expiration );
void registerDevice( List channels, AsyncCallback callback );
void registerDevice( List channels, Date expiration, AsyncCallback callback );
void unregisterDevice();
void unregisterDevice( List channels );
void unregisterDevice( AsyncCallback callback );
void unregisterDevice( final List channels, final AsyncCallback callback );
boolean refreshDeviceToken( String newDeviceToken );
void refreshDeviceToken( String newDeviceToken, final AsyncCallback responder );
DeviceRegistration getDeviceRegistration();
DeviceRegistration getRegistrations();
void getDeviceRegistration( AsyncCallback responder );
void getRegistrations( AsyncCallback responder );
/**
* Publishes message to "default" channel. The message is not a push notification, it does not have any headers and
* does not go into any subtopics.
*
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @return a data structure which contains ID of the published message and the status of the publish operation.
* @throws BackendlessException
*/
MessageStatus publish( Object message );
/**
* Publishes message to specified channel. The message is not a push notification, it does not have any headers and
* does not go into any subtopics.
*
* @param channelName name of a channel to publish the message to. If the channel does not exist, Backendless
* automatically creates it.
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @return ${@link com.backendless.messaging.MessageStatus} - a data structure which contains ID of the published
* message and the status of the publish operation.
* @throws BackendlessException
*/
MessageStatus publish( String channelName, Object message );
/**
* Publishes message to specified channel. The message is not a push notification, it may have headers and/or subtopic
* defined in the publishOptions argument.
*
* @param channelName name of a channel to publish the message to. If the channel does not exist, Backendless
* automatically creates it.
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @param publishOptions an instance of ${@link PublishOptions}. When provided may contain
* publisher ID (an arbitrary, application-specific string value identifying the publisher),
* subtopic value and/or a collection of headers.
* @return a data structure which contains ID of the published message and the status of the publish operation.
*/
MessageStatus publish( String channelName, Object message, PublishOptions publishOptions );
/**
* Publishes message to specified channel.The message may be configured as a push notification. It may have headers
* and/or subtopic defined in the publishOptions argument.
*
* @param channelName name of a channel to publish the message to. If the channel does not exist, Backendless
* automatically creates it.
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @param publishOptions an instance of ${@link PublishOptions}. When provided may contain
* publisher ID (an arbitrary, application-specific string value identifying the publisher),
* subtopic value and/or a collection of headers.
* @param deliveryOptions an instance of ${@link DeliveryOptions}. When provided may specify
* options for message delivery such as: deliver as a push notification, deliver to specific
* devices (or a group of devices grouped by the operating system), delayed delivery or repeated
* delivery.
* @return a data structure which contains ID of the published message and the status of the publish operation.
*/
MessageStatus publish( String channelName, Object message, PublishOptions publishOptions, DeliveryOptions deliveryOptions );
/**
* Publishes message to "default" channel. The message is not a push notification, it does not have any headers and
* does not go into any subtopics.
*
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @param publishOptions an instance of ${@link PublishOptions}. When provided may contain
* publisher ID (an arbitrary, application-specific string value identifying the publisher),
* subtopic value and/or a collection of headers.
* @return a data structure which contains ID of the published message and the status of the publish operation.
* @throws BackendlessException
*/
MessageStatus publish( Object message, PublishOptions publishOptions );
/**
* Publishes message to "default" channel.The message may be configured as a push notification. It may have headers
* and/or subtopic defined in the publishOptions argument.
*
* @param message object to publish. The object can be of any data type - a primitive value, String, Date, a
* user-defined complex type, a collection or an array of these types.
* @param publishOptions an instance of ${@link PublishOptions}. When provided may contain
* publisher ID (an arbitrary, application-specific string value identifying the publisher),
* subtopic value and/or a collection of headers.
* @param deliveryOptions an instance of ${@link DeliveryOptions}. When provided may specify
* options for message delivery such as: deliver as a push notification, deliver to specific
* devices (or a group of devices grouped by the operating system), delayed delivery or repeated
* delivery.
* @return a data structure which contains ID of the published message and the status of the publish operation.
*/
MessageStatus publish( Object message, PublishOptions publishOptions, DeliveryOptions deliveryOptions );
void publish( Object message, final AsyncCallback responder );
void publish( String channelName, Object message, final AsyncCallback responder );
void publish( String channelName, Object message, PublishOptions publishOptions, final AsyncCallback responder );
void publish( String channelName, Object message, PublishOptions publishOptions, DeliveryOptions deliveryOptions,
final AsyncCallback responder );
void publish( Object message, PublishOptions publishOptions, final AsyncCallback responder );
void publish( Object message, PublishOptions publishOptions, DeliveryOptions deliveryOptions,
final AsyncCallback responder );
MessageStatus pushWithTemplate( String templateName );
MessageStatus pushWithTemplate( String templateName, Map templateValues );
void pushWithTemplate( String templateName, final AsyncCallback responder );
void pushWithTemplate( String templateName, Map templateValues, final AsyncCallback responder );
MessageStatus getMessageStatus( String messageId );
void getMessageStatus( String messageId, AsyncCallback responder );
boolean cancel( String messageId );
void cancel( String messageId, AsyncCallback responder );
Channel subscribe();
Channel subscribe( String channelName );
List pollMessages( String channelName, String subscriptionId );
MessageStatus sendTextEmail( String subject, String messageBody, List recipients );
MessageStatus sendTextEmail( String subject, String messageBody, String recipient );
MessageStatus sendHTMLEmail( String subject, String messageBody, List recipients );
MessageStatus sendHTMLEmail( String subject, String messageBody, String recipient );
MessageStatus sendEmail( String subject, BodyParts bodyParts, String recipient, List attachments );
MessageStatus sendEmail( String subject, BodyParts bodyParts, String recipient );
MessageStatus sendEmail( String subject, BodyParts bodyParts, List recipients, List attachments );
void sendTextEmail( String subject, String messageBody, List recipients, final AsyncCallback responder );
void sendTextEmail( String subject, String messageBody, String recipient, final AsyncCallback responder );
void sendHTMLEmail( String subject, String messageBody, List recipients, final AsyncCallback responder );
void sendHTMLEmail( String subject, String messageBody, String recipient, final AsyncCallback responder );
void sendEmail( String subject, BodyParts bodyParts, String recipient, List attachments,
final AsyncCallback responder );
void sendEmail( String subject, BodyParts bodyParts, String recipient, final AsyncCallback responder );
void sendEmail( String subject, BodyParts bodyParts, List recipients, List attachments,
final AsyncCallback responder );
MessageStatus sendEmailFromTemplate( String templateName, EmailEnvelope envelope );
MessageStatus sendEmailFromTemplate( String templateName, EmailEnvelope envelope, Map templateValues );
MessageStatus sendEmailFromTemplate( String templateName, EmailEnvelope envelope, List attachments );
MessageStatus sendEmailFromTemplate( String templateName, EmailEnvelope envelope, Map templateValues,
List attachments );
void sendEmailFromTemplate( String templateName, EmailEnvelope envelope, AsyncCallback responder );
void sendEmailFromTemplate( String templateName, EmailEnvelope envelope, Map templateValues,
AsyncCallback responder );
void sendEmailFromTemplate( String templateName, EmailEnvelope envelope, List attachments,
AsyncCallback responder );
void sendEmailFromTemplate( String templateName, EmailEnvelope envelope, Map templateValues, List attachments,
AsyncCallback responder );
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy