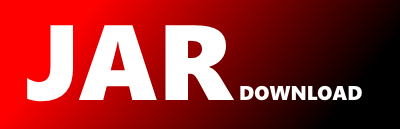
com.backendless.hive.Hive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless.hive;
import java.util.Set;
import java.util.WeakHashMap;
import java.util.concurrent.CompletableFuture;
public final class Hive
{
private final static WeakHashMap hives = new WeakHashMap<>();
private final static WeakHashMap> listHives = new WeakHashMap<>();
private final static WeakHashMap> setHives = new WeakHashMap<>();
private final static WeakHashMap> sortedSetHives = new WeakHashMap<>();
private final static WeakHashMap> mapHives = new WeakHashMap<>();
private static final HiveManagement hiveManagement = HiveManagement.getInstance();
private final String hiveName;
private final HiveKeyValue hiveKeyValue;
private final HiveGeneralWithoutStoreKey generalKeyValueOps;
private final HiveGeneralWithoutStoreKey generalListOps;
private final HiveGeneralWithoutStoreKeyForSet generalSetOps;
private final HiveGeneralWithoutStoreKeyForSortedSet generalSortedSetOps;
private final HiveGeneralWithoutStoreKey generalMapOps;
private Hive( String name )
{
this.hiveName = name;
this.generalKeyValueOps = new HiveGeneralWithoutStoreKey( hiveName, StoreType.KeyValue, hiveManagement );
this.hiveKeyValue = new HiveKeyValue( hiveName, generalKeyValueOps );
this.generalListOps = new HiveGeneralWithoutStoreKey( hiveName, StoreType.List, hiveManagement );
this.generalSetOps = new HiveGeneralWithoutStoreKeyForSet( hiveName, StoreType.Set, hiveManagement );
this.generalSortedSetOps = new HiveGeneralWithoutStoreKeyForSortedSet( hiveName, StoreType.SortedSet, hiveManagement );
this.generalMapOps = new HiveGeneralWithoutStoreKey( hiveName, StoreType.Map, hiveManagement );
}
public static Hive getOrCreate( String name )
{
Hive hive = hives.get( name );
if( hive == null )
{
hive = new Hive( name );
hives.put( name, hive );
}
return hive;
}
// ----------------------------------------
public static CompletableFuture> getNames()
{
return hiveManagement.getNames();
}
public static CompletableFuture create( String name )
{
return hiveManagement.create( name );
}
public static CompletableFuture rename( String name, String newName )
{
return hiveManagement.rename( name, newName );
}
public static CompletableFuture delete( String name )
{
return hiveManagement.delete( name );
}
public static CompletableFuture deleteAll()
{
return hiveManagement.deleteAll();
}
// ----------------------------------------
public HiveKeyValue KeyValueStore()
{
return hiveKeyValue;
}
public HiveGeneralWithoutStoreKey ListStore()
{
return this.generalListOps;
}
public HiveList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy