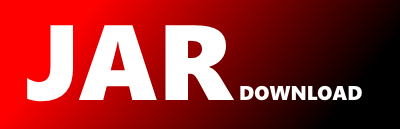
com.backendless.hive.HiveList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless.hive;
import com.backendless.core.responder.AdaptingResponder;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
public final class HiveList extends HiveGeneralForComplexStore
{
public final static String HIVE_LIST_ALIAS = "com.backendless.services.hive.HiveListService";
HiveList( String hiveName, String storeKey )
{
super( hiveName, StoreType.List, storeKey );
}
public CompletableFuture> get()
{
return this.makeRemoteCall( "get" )
.thenApply( HiveSerializer::deserializeAsList );
}
public CompletableFuture> get( int start, int stop )
{
return this.makeRemoteCall( "get", start, stop )
.thenApply( HiveSerializer::deserializeAsList );
}
public CompletableFuture get( int index )
{
return this.makeRemoteCall( "get", index )
.thenApply( HiveSerializer::deserialize );
}
public CompletableFuture set( List values )
{
return makeRemoteCall( "set", new AdaptingResponder<>( Long.class ), HiveSerializer.serializeAsList( values ) );
}
public CompletableFuture set( int index, Object value )
{
return makeRemoteCall( "set", index, HiveSerializer.serialize( value ) );
}
public CompletableFuture insertBefore( Object targetValue, Object value )
{
return insert( targetValue, value, true );
}
public CompletableFuture insertAfter( Object targetValue, Object value )
{
return insert( targetValue, value, false );
}
private CompletableFuture insert( Object targetValue, Object value, boolean before )
{
return makeRemoteCall( "insert", new AdaptingResponder<>( Long.class ), HiveSerializer.serialize( targetValue ), HiveSerializer.serialize( value ), before );
}
public CompletableFuture addFirst( T value )
{
return addFirst( Collections.singletonList( value ) );
}
public CompletableFuture addFirst( List values )
{
return makeRemoteCall( "addFirst", new AdaptingResponder<>( Long.class ), HiveSerializer.serializeAsList( values ) );
}
public CompletableFuture addLast( T value )
{
return addLast( Collections.singletonList( value ) );
}
public CompletableFuture addLast( List values )
{
return makeRemoteCall( "addLast", new AdaptingResponder<>( Long.class ), HiveSerializer.serializeAsList( values ) );
}
public CompletableFuture deleteAndReturnFirst()
{
return this.makeRemoteCall( "removeAndReturnFirst" )
.thenApply( HiveSerializer::deserialize );
}
public CompletableFuture deleteAndReturnLast()
{
return this.makeRemoteCall( "removeAndReturnLast" )
.thenApply( HiveSerializer::deserialize );
}
public CompletableFuture> deleteAndReturnFirst( int count )
{
return this.makeRemoteCall( "removeAndReturnFirst", count )
.thenApply( HiveSerializer::deserializeAsList );
}
public CompletableFuture> deleteAndReturnLast( int count )
{
return this.makeRemoteCall( "removeAndReturnLast", count )
.thenApply( HiveSerializer::deserializeAsList );
}
public CompletableFuture deleteValue( T value, int count )
{
return makeRemoteCall( "removeValue", new AdaptingResponder<>( Long.class ), HiveSerializer.serialize( value ), count );
}
public CompletableFuture length()
{
return makeRemoteCall( "length", new AdaptingResponder<>( Long.class ) );
}
// ----------------------------------------
private CompletableFuture makeRemoteCall( String methodName, Object... args )
{
return makeRemoteCallWithStoreKey( HIVE_LIST_ALIAS, methodName, args );
}
private CompletableFuture makeRemoteCall( String methodName, AdaptingResponder adaptingResponder, Object... args )
{
return makeRemoteCallWithStoreKey( HIVE_LIST_ALIAS, methodName, adaptingResponder, args );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy