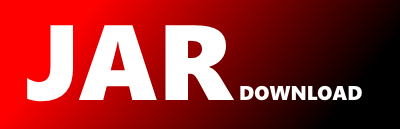
com.backendless.hive.HiveSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless.hive;
import com.backendless.core.responder.AdaptingResponder;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
public final class HiveSet extends HiveGeneralForComplexStore
{
public final static String HIVE_SET_ALIAS = "com.backendless.services.hive.HiveSetService";
HiveSet( String hiveName, String storeKey )
{
super( hiveName, StoreType.Set, storeKey );
}
public CompletableFuture> get()
{
return this.makeRemoteCall( "get" )
.thenApply( HiveSerializer::deserializeAsSet );
}
public CompletableFuture> getRandom( int count )
{
return this.makeRemoteCall( "getRandom", count )
.thenApply( HiveSerializer::deserializeAsList );
}
public CompletableFuture> getRandomAndDel( int count )
{
return this.makeRemoteCall( "getRandomAndDel", count )
.thenApply( HiveSerializer::deserializeAsSet );
}
public CompletableFuture isValueMember( T value )
{
return makeRemoteCall( "contains", HiveSerializer.serialize( value ) );
}
public CompletableFuture> isValueMember( Set values )
{
return makeRemoteCall( "contains", new AdaptingResponder<>( List.class ), HiveSerializer.serializeAsList( values ) )
.thenApply( result -> (List) result );
}
public CompletableFuture length()
{
return this.makeRemoteCall( "size", new AdaptingResponder<>( Long.class ) );
}
public CompletableFuture add( T value )
{
return add( Collections.singleton( value ) );
}
public CompletableFuture add( Set values )
{
return makeRemoteCall( "add", new AdaptingResponder<>( Long.class ), HiveSerializer.serializeAsList( values ) );
}
public CompletableFuture delete( Set values )
{
return this.makeRemoteCall( "del", new AdaptingResponder<>( Long.class ), HiveSerializer.serializeAsList( values ) );
}
// ----------------------------------------
private CompletableFuture makeRemoteCall( String methodName, Object... args )
{
return makeRemoteCallWithStoreKey( HIVE_SET_ALIAS, methodName, args );
}
private CompletableFuture makeRemoteCall( String methodName, AdaptingResponder adaptingResponder, Object... args )
{
return makeRemoteCallWithStoreKey( HIVE_SET_ALIAS, methodName, adaptingResponder, args );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy