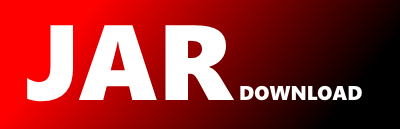
com.backendless.hive.ScoreValuePair Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless.hive;
import lombok.Getter;
import lombok.ToString;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Objects;
@Getter
@ToString
public class ScoreValuePair
{
final private double score;
final private T value;
public ScoreValuePair( double score, T value )
{
if( value == null )
throw new NullPointerException( "Value is null for score: " + score );
this.score = score;
this.value = value;
}
public static LinkedHashSet> from( Object[] arrayItems )
{
if( arrayItems.length % 2 != 0 )
throw new IllegalArgumentException( "Wrong length of incoming array for ScoreValuePair. It should contain even numbers of elements." );
LinkedHashSet> items = new LinkedHashSet<>();
for( int i = 0; i < arrayItems.length; i += 2 )
items.add( new ScoreValuePair<>( ((Number) arrayItems[ i ]).doubleValue(), (T) arrayItems[ i + 1 ] ) );
return items;
}
static Object[] toObjectArray( Collection> items )
{
Object[] arrayItems = new Object[ items.size() * 2 ];
int i = 0;
for( ScoreValuePair item : items )
{
arrayItems[ i * 2 ] = item.getScore();
arrayItems[ i * 2 + 1 ] = HiveSerializer.serialize( item.getValue() );
i++;
}
return arrayItems;
}
static ArrayList> fromObjectArrayToList( Object[] arrayItems )
{
if( arrayItems.length % 2 != 0 )
throw new IllegalArgumentException( "Wrong length of incoming array for ScoreValuePair. It should contain even numbers of elements." );
ArrayList> items = new ArrayList<>();
for( int i = 0; i < arrayItems.length; i += 2 )
items.add( new ScoreValuePair<>( ((Number) arrayItems[ i ]).doubleValue(), HiveSerializer.deserialize( (String) arrayItems[ i + 1 ] ) ) );
return items;
}
static LinkedHashSet> fromObjectArray( Object[] arrayItems )
{
if( arrayItems.length % 2 != 0 )
throw new IllegalArgumentException( "Wrong length of incoming array for ScoreValuePair. It should contain even numbers of elements." );
LinkedHashSet> items = new LinkedHashSet<>();
for( int i = 0; i < arrayItems.length; i += 2 )
items.add( new ScoreValuePair<>( ((Number) arrayItems[ i ]).doubleValue(), HiveSerializer.deserialize( (String) arrayItems[ i + 1 ] ) ) );
return items;
}
@Override
public boolean equals( Object o )
{
if( this == o )
return true;
if( !(o instanceof ScoreValuePair) )
return false;
ScoreValuePair> that = (ScoreValuePair>) o;
return Double.compare( that.score, score ) == 0 && value.equals( that.value );
}
@Override
public int hashCode()
{
return Objects.hash( value );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy